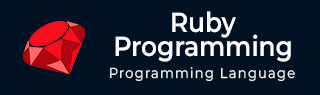
- Ruby基础知识
- Ruby - 主页
- Ruby - 概述
- Ruby - 环境设置
- Ruby - 语法
- Ruby - 类和对象
- Ruby - 变量
- Ruby - 运算符
- Ruby - 评论
- Ruby - IF...ELSE
- Ruby - 循环
- Ruby - 方法
- Ruby - 块
- Ruby - 模块
- Ruby - 字符串
- Ruby - 数组
- Ruby - 哈希
- Ruby - 日期和时间
- Ruby - 范围
- Ruby - 迭代器
- Ruby - 文件 I/O
- Ruby - 例外
Ruby 类案例研究
对于您的案例研究,您将创建一个名为 Customer 的 Ruby 类,并声明两个方法 -
display_details - 此方法将显示客户的详细信息。
Total_no_of_customers - 此方法将显示系统中创建的客户总数。
#!/usr/bin/ruby class Customer @@no_of_customers = 0 def initialize(id, name, addr) @cust_id = id @cust_name = name @cust_addr = addr end def display_details() puts "Customer id #@cust_id" puts "Customer name #@cust_name" puts "Customer address #@cust_addr" end def total_no_of_customers() @@no_of_customers += 1 puts "Total number of customers: #@@no_of_customers" end end
display_details方法包含三个put语句,显示客户 ID、客户名称和客户地址。put 语句将在一行中显示文本 Customer id,后跟变量 @cust_id 的值,如下所示 -
puts "Customer id #@cust_id"
当您想要在一行中显示文本和实例变量的值时,需要在 put 语句中在变量名称前面添加井号 (#)。文本和实例变量以及哈希符号 (#) 应括在双引号内。
第二个方法total_no_of_customers 是包含类变量@@no_of_customers 的方法。每次调用方法total_no_of_customers 时,表达式@@no_of_customers+=1 将变量no_of_customers 加1。这样,您将始终在类变量中拥有客户总数。
现在,创建两个客户,如下所示 -
cust1 = Customer.new("1", "John", "Wisdom Apartments, Ludhiya") cust2 = Customer.new("2", "Poul", "New Empire road, Khandala")
在这里,我们创建了 Customer 类的两个对象 cust1 和 cust2,并使用 new 方法传递必要的参数。调用initialize方法,并初始化对象的必要属性。
创建对象后,您需要使用这两个对象来调用类的方法。如果您想调用方法或任何数据成员,请编写以下内容 -
cust1.display_details() cust1.total_no_of_customers()
对象名称后面应始终跟有一个点,而点后面又跟有方法名称或任何数据成员。我们已经了解了如何使用 cust1 对象调用这两个方法。使用 cust2 对象,您可以调用这两种方法,如下所示 -
cust2.display_details() cust2.total_no_of_customers()
保存并执行代码
现在,将所有这些源代码放入 main.rb 文件中,如下所示 -
#!/usr/bin/ruby class Customer @@no_of_customers = 0 def initialize(id, name, addr) @@no_of_customers += 1 @cust_id = id @cust_name = name @cust_addr = addr end def display_details() puts "Customer id #@cust_id" puts "Customer name #@cust_name" puts "Customer address #@cust_addr" end def total_no_of_customers() puts "Total number of customers: #@@no_of_customers" end end # Create Objects cust1 = Customer.new("1", "John", "Wisdom Apartments, Ludhiya") cust2 = Customer.new("2", "Poul", "New Empire road, Khandala") # Call Methods cust1.display_details() cust1.total_no_of_customers() cust2.display_details() cust2.total_no_of_customers() cust3 = Customer.new("3", "Raghu", "Madapur, Hyderabad") cust4 = Customer.new("4", "Rahman", "Akkayya palem, Vishakhapatnam") cust4.total_no_of_customers()
现在,运行该程序如下 -
$ ruby main.rb
这将产生以下结果 -
Customer id 1 Customer name John Customer address Wisdom Apartments, Ludhiya Total number of customers: 2 Customer id 2 Customer name Poul Customer address New Empire road, Khandala Total number of customers: 2 Total number of customers: 4
ruby_classes.htm