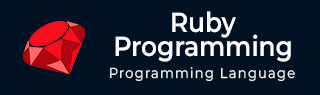
- Ruby基础知识
- Ruby - 主页
- Ruby - 概述
- Ruby - 环境设置
- Ruby - 语法
- Ruby - 类和对象
- Ruby - 变量
- Ruby - 运算符
- Ruby - 评论
- Ruby - IF...ELSE
- Ruby - 循环
- Ruby - 方法
- Ruby - 块
- Ruby - 模块
- Ruby - 字符串
- Ruby - 数组
- Ruby - 哈希
- Ruby - 日期和时间
- Ruby - 范围
- Ruby - 迭代器
- Ruby - 文件 I/O
- Ruby - 例外
Ruby - 循环
Ruby 中的循环用于执行同一代码块指定的次数。本章详细介绍了 Ruby 支持的所有循环语句。
Ruby while 语句
句法
while conditional [do] code end
当条件为真时执行代码。while循环的条件通过保留字 do、换行符、反斜杠 \ 或分号 ; 与代码分隔。
例子
#!/usr/bin/ruby $i = 0 $num = 5 while $i < $num do puts("Inside the loop i = #$i" ) $i +=1 end
这将产生以下结果 -
Inside the loop i = 0 Inside the loop i = 1 Inside the loop i = 2 Inside the loop i = 3 Inside the loop i = 4
Ruby while 修饰符
句法
code while condition OR begin code end while conditional
当条件为真时执行代码。
如果while修饰符跟在begin语句后面且没有救援或 Ensure 子句,则在评估条件之前执行一次代码。
例子
#!/usr/bin/ruby $i = 0 $num = 5 begin puts("Inside the loop i = #$i" ) $i +=1 end while $i < $num
这将产生以下结果 -
Inside the loop i = 0 Inside the loop i = 1 Inside the loop i = 2 Inside the loop i = 3 Inside the loop i = 4
Ruby 直到声明
until conditional [do] code end
当条件为 false时执行代码。Until语句的条件通过保留字do、换行符或分号与代码分隔。
例子
#!/usr/bin/ruby $i = 0 $num = 5 until $i > $num do puts("Inside the loop i = #$i" ) $i +=1; end
这将产生以下结果 -
Inside the loop i = 0 Inside the loop i = 1 Inside the loop i = 2 Inside the loop i = 3 Inside the loop i = 4 Inside the loop i = 5
Ruby 直到修饰符
句法
code until conditional OR begin code end until conditional
当条件为 false时执行代码。
如果在begin语句后面有一个until修饰符,但没有rescue或ensure 子句,则在计算条件之前会执行一次代码。
例子
#!/usr/bin/ruby $i = 0 $num = 5 begin puts("Inside the loop i = #$i" ) $i +=1; end until $i > $num
这将产生以下结果 -
Inside the loop i = 0 Inside the loop i = 1 Inside the loop i = 2 Inside the loop i = 3 Inside the loop i = 4 Inside the loop i = 5
Ruby for 语句
句法
for variable [, variable ...] in expression [do] code end
对expression中的每个元素执行一次代码。
例子
#!/usr/bin/ruby for i in 0..5 puts "Value of local variable is #{i}" end
在这里,我们定义了范围 0..5。for i in 0..5 的语句将允许i取 0 到 5 范围内的值(包括 5)。这将产生以下结果 -
Value of local variable is 0 Value of local variable is 1 Value of local variable is 2 Value of local variable is 3 Value of local variable is 4 Value of local variable is 5
for ...in循环几乎完全等同于以下内容 -
(expression).each do |variable[, variable...]| code end
除了for循环不会为局部变量创建新的作用域之外。for循环的表达式通过保留字 do、换行符或分号与代码分隔。
例子
#!/usr/bin/ruby (0..5).each do |i| puts "Value of local variable is #{i}" end
这将产生以下结果 -
Value of local variable is 0 Value of local variable is 1 Value of local variable is 2 Value of local variable is 3 Value of local variable is 4 Value of local variable is 5
Ruby 中断语句
句法
break
终止最内部的循环。如果在块内调用(方法返回 nil),则终止具有关联块的方法。
例子
#!/usr/bin/ruby for i in 0..5 if i > 2 then break end puts "Value of local variable is #{i}" end
这将产生以下结果 -
Value of local variable is 0 Value of local variable is 1 Value of local variable is 2
Ruby 下一个声明
句法
next
跳转到最内部循环的下一次迭代。如果在块内调用(yield或调用返回 nil),则终止块的执行。
例子
#!/usr/bin/ruby for i in 0..5 if i < 2 then next end puts "Value of local variable is #{i}" end
这将产生以下结果 -
Value of local variable is 2 Value of local variable is 3 Value of local variable is 4 Value of local variable is 5
Ruby 重做语句
句法
redo
重新启动最内部循环的迭代,而不检查循环条件。如果在块内调用,则重新启动yield或call 。
例子
#!/usr/bin/ruby for i in 0..5 if i < 2 then puts "Value of local variable is #{i}" redo end end
这将产生以下结果并将进入无限循环 -
Value of local variable is 0 Value of local variable is 0 ............................
Ruby 重试语句
句法
retry
如果重试出现在begin表达式的rescue子句中,则从begin主体的开头重新开始。
begin do_something # exception raised rescue # handles error retry # restart from beginning end
如果 retry 出现在迭代器、块或for表达式的主体中,则会重新启动迭代器调用。迭代器的参数被重新评估。
for i in 1..5 retry if some_condition # restart from i == 1 end
例子
#!/usr/bin/ruby for i in 0..5 retry if i > 2 puts "Value of local variable is #{i}" end
这将产生以下结果并将进入无限循环 -
Value of local variable is 1 Value of local variable is 2 Value of local variable is 1 Value of local variable is 2 Value of local variable is 1 Value of local variable is 2 ............................