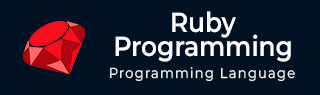
- Ruby基础知识
- Ruby - 主页
- Ruby - 概述
- Ruby - 环境设置
- Ruby - 语法
- Ruby - 类和对象
- Ruby - 变量
- Ruby - 运算符
- Ruby - 评论
- Ruby - IF...ELSE
- Ruby - 循环
- Ruby - 方法
- Ruby - 块
- Ruby - 模块
- Ruby - 字符串
- Ruby - 数组
- Ruby - 哈希
- Ruby - 日期和时间
- Ruby - 范围
- Ruby - 迭代器
- Ruby - 文件 I/O
- Ruby - 例外
Ruby - 日期和时间
Time类表示 Ruby 中的日期和时间。它是操作系统提供的系统日期和时间功能之上的一个薄层。此类可能无法在您的系统上表示 1970 年之前或 2038 年之后的日期。
本章使您熟悉所有最需要的日期和时间概念。
获取当前日期和时间
以下是获取当前日期和时间的简单示例 -
#!/usr/bin/ruby -w time1 = Time.new puts "Current Time : " + time1.inspect # Time.now is a synonym: time2 = Time.now puts "Current Time : " + time2.inspect
这将产生以下结果 -
Current Time : Mon Jun 02 12:02:39 -0700 2008 Current Time : Mon Jun 02 12:02:39 -0700 2008
获取日期和时间的组成部分
我们可以使用Time对象来获取日期和时间的各个组成部分。以下是显示相同内容的示例 -
#!/usr/bin/ruby -w time = Time.new # Components of a Time puts "Current Time : " + time.inspect puts time.year # => Year of the date puts time.month # => Month of the date (1 to 12) puts time.day # => Day of the date (1 to 31 ) puts time.wday # => 0: Day of week: 0 is Sunday puts time.yday # => 365: Day of year puts time.hour # => 23: 24-hour clock puts time.min # => 59 puts time.sec # => 59 puts time.usec # => 999999: microseconds puts time.zone # => "UTC": timezone name
这将产生以下结果 -
Current Time : Mon Jun 02 12:03:08 -0700 2008 2008 6 2 1 154 12 3 8 247476 UTC
Time.utc、Time.gm 和 Time.local 函数
这两个函数可用于以标准格式格式化日期,如下所示 -
# July 8, 2008 Time.local(2008, 7, 8) # July 8, 2008, 09:10am, local time Time.local(2008, 7, 8, 9, 10) # July 8, 2008, 09:10 UTC Time.utc(2008, 7, 8, 9, 10) # July 8, 2008, 09:10:11 GMT (same as UTC) Time.gm(2008, 7, 8, 9, 10, 11)
以下是按以下格式获取数组中所有组件的示例 -
[sec,min,hour,day,month,year,wday,yday,isdst,zone]
尝试以下操作 -
#!/usr/bin/ruby -w time = Time.new values = time.to_a p values
这将生成以下结果 -
[26, 10, 12, 2, 6, 2008, 1, 154, false, "MST"]
该数组可以传递给Time.utc或Time.local函数来获取不同格式的日期,如下所示 -
#!/usr/bin/ruby -w time = Time.new values = time.to_a puts Time.utc(*values)
这将生成以下结果 -
Mon Jun 02 12:15:36 UTC 2008
以下是获取自(平台相关)纪元以来内部以秒为单位表示的时间的方法 -
# Returns number of seconds since epoch time = Time.now.to_i # Convert number of seconds into Time object. Time.at(time) # Returns second since epoch which includes microseconds time = Time.now.to_f
时区和夏令时
您可以使用Time对象获取与时区和夏令时相关的所有信息,如下所示 -
time = Time.new # Here is the interpretation time.zone # => "UTC": return the timezone time.utc_offset # => 0: UTC is 0 seconds offset from UTC time.zone # => "PST" (or whatever your timezone is) time.isdst # => false: If UTC does not have DST. time.utc? # => true: if t is in UTC time zone time.localtime # Convert to local timezone. time.gmtime # Convert back to UTC. time.getlocal # Return a new Time object in local zone time.getutc # Return a new Time object in UTC
设置时间和日期的格式
设置日期和时间格式的方法有多种。这是一个例子,展示了一些 -
#!/usr/bin/ruby -w time = Time.new puts time.to_s puts time.ctime puts time.localtime puts time.strftime("%Y-%m-%d %H:%M:%S")
这将产生以下结果 -
Mon Jun 02 12:35:19 -0700 2008 Mon Jun 2 12:35:19 2008 Mon Jun 02 12:35:19 -0700 2008 2008-06-02 12:35:19
时间格式指令
下表中的这些指令与Time.strftime方法一起使用。
先生。 | 指令和说明 |
---|---|
1 | %A 缩写的工作日名称 (Sun)。 |
2 | %A 工作日的完整名称(星期日)。 |
3 | %b 月份名称的缩写 (Jan)。 |
4 | %B 完整的月份名称(一月)。 |
5 | %C 首选的本地日期和时间表示形式。 |
6 | %d 一个月中的某一天(01 到 31)。 |
7 | %H 一天中的小时,24 小时制(00 至 23)。 |
8 | %我 一天中的小时,12 小时制(01 到 12)。 |
9 | %j 一年中的某一天(001 到 366)。 |
10 | %m 一年中的月份(01 到 12)。 |
11 | %M 一小时中的分钟(00 到 59)。 |
12 | %p 子午线指示器(AM 或 PM)。 |
13 | %S 分钟的秒数(00 到 60)。 |
14 | %U 当前年份的周数,从第一个星期日开始作为第一周的第一天(00 到 53)。 |
15 | %W 当前年份的周数,从第一个星期一开始作为第一周的第一天(00 到 53)。 |
16 | %w 星期几(星期日是 0、0 到 6)。 |
17 号 | %X 首选只表示日期,不表示时间。 |
18 | %X 首选单独表示时间,没有日期。 |
19 | %y 没有世纪的年份(00 到 99)。 |
20 | %Y 年与世纪。 |
21 | %Z 时区名称。 |
22 | %% 文字 % 字符。 |
时间算术
您可以用时间执行简单的算术,如下所示 -
now = Time.now # Current time puts now past = now - 10 # 10 seconds ago. Time - number => Time puts past future = now + 10 # 10 seconds from now Time + number => Time puts future diff = future - past # => 10 Time - Time => number of seconds puts diff
这将产生以下结果 -
Thu Aug 01 20:57:05 -0700 2013 Thu Aug 01 20:56:55 -0700 2013 Thu Aug 01 20:57:15 -0700 2013 20.0