WCF - 创建 WCF 服务
使用 Microsoft Visual Studio 2012 创建 WCF 服务是一项简单的任务。下面给出了创建 WCF 服务的分步方法以及所有必需的编码,以便更好地理解该概念。
- 启动 Visual Studio 2012。
- 单击“新项目”,然后在“Visual C#”选项卡中选择“WCF”选项。

创建的 WCF 服务执行基本算术运算,例如加法、减法、乘法和除法。主要代码位于两个不同的文件中——一个接口和一个类。
WCF 包含一个或多个接口及其实现的类。
using System; using System.Collections.Generic; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.Text; namespace WcfServiceLibrary1 { // NOTE: You can use the "Rename" command on the "Refactor" menu to // change the interface name "IService1" in both code and config file // together. [ServiceContract] Public interface IService1 { [OperationContract] int sum(int num1, int num2); [OperationContract] int Subtract(int num1, int num2); [OperationContract] int Multiply(int num1, int num2); [OperationContract] int Divide(int num1, int num2); } // Use a data contract as illustrated in the sample below to add // composite types to service operations. [DataContract] Public class CompositeType { Bool boolValue = true; String stringValue = "Hello "; [DataMember] Public bool BoolValue { get { return boolValue; } set { boolValue = value; } } [DataMember] Public string StringValue { get { return stringValue; } set { stringValue = value; } } } }
下面给出了其类背后的代码。
using System; usingSystem.Collections.Generic; usingSystem.Linq; usingSystem.Runtime.Serialization; usingSystem.ServiceModel; usingSystem.Text; namespace WcfServiceLibrary1 { // NOTE: You can use the "Rename" command on the "Refactor" menu to // change the class name "Service1" in both code and config file // together. publicclassService1 :IService1 { // This Function Returns summation of two integer numbers publicint sum(int num1, int num2) { return num1 + num2; } // This function returns subtraction of two numbers. // If num1 is smaller than number two then this function returns 0 publicint Subtract(int num1, int num2) { if (num1 > num2) { return num1 - num2; } else { return 0; } } // This function returns multiplication of two integer numbers. publicint Multiply(int num1, int num2) { return num1 * num2; } // This function returns integer value of two integer number. // If num2 is 0 then this function returns 1. publicint Divide(int num1, int num2) { if (num2 != 0) { return (num1 / num2); } else { return 1; } } } }
要运行此服务,请单击 Visual Studio 中的“开始”按钮。

当我们运行此服务时,会出现以下屏幕。
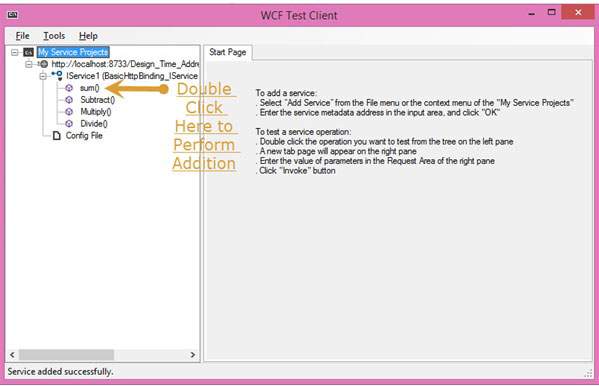
单击求和方法后,将打开以下页面。在这里,您可以输入任意两个整数,然后单击“调用”按钮。该服务将返回这两个数字的总和。
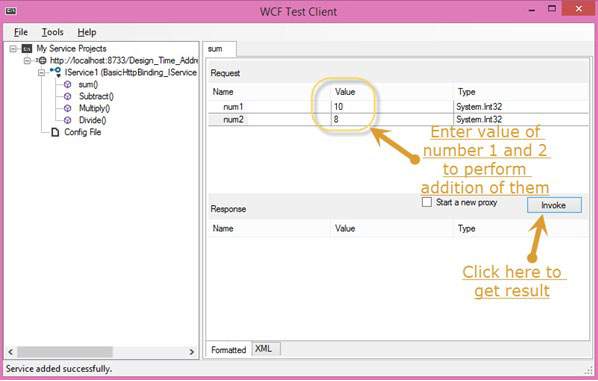

与求和一样,我们可以执行菜单中列出的所有其他算术运算。这是他们的快照。
单击“减去”方法后将出现以下页面。输入整数,单击“调用”按钮,并获得如下所示的输出 -

单击乘法方法后将出现以下页面。输入整数,单击“调用”按钮,并获得如下所示的输出 -

单击“除法”方法后将出现以下页面。输入整数,单击“调用”按钮,并获得如下所示的输出 -

调用服务后,您可以直接从此处在它们之间进行切换。
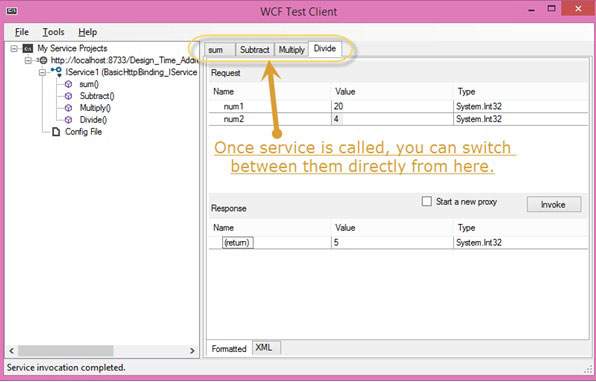