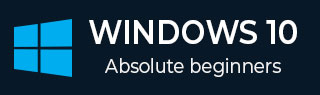
- Windows 10 开发教程
- Windows 10 - 主页
- Windows 10 - 简介
- Windows 10 – UWP
- Windows 10 – 第一个应用程序
- Windows 10 - 商店
- Windows 10 - XAML 控件
- Windows 10 - 数据绑定
- Windows 10 - XAML 性能
- Windows 10 - 自适应设计
- Windows 10 - 自适应 UI
- Windows 10 - 自适应代码
- Windows 10 - 文件管理
- Windows 10 - SQLite 数据库
- Windows 10 – 通讯
- Windows 10 - 应用程序本地化
- Windows 10 - 应用程序生命周期
- Windows 10 - 后台执行
- Windows 10 - 应用程序服务
- Windows 10 - 网络平台
- Windows 10 - 互联体验
- Windows 10 - 导航
- Windows 10 - 网络
- Windows 10 - 云服务
- Windows 10 - 动态磁贴
- Windows 10 - 共享合同
- Windows 10 - 移植到 Windows
- Windows 10 有用资源
- Windows 10 - 快速指南
- Windows 10 - 有用的资源
- Windows 10 - 讨论
Windows10开发-文件管理
在任何应用程序中,最重要的事情之一就是数据。如果您是.net开发人员,您可能了解隔离存储,并且通用 Windows 平台 (UWP) 应用程序遵循相同的概念。
文件位置
这些是您的应用程序可以访问数据的区域。该应用程序包含一些区域,该区域是该特定应用程序私有的,其他应用程序无法访问,但还有许多其他区域,您可以在其中存储和保存数据到文件中。

下面给出了每个文件夹的简要说明。
编号 | 文件夹和说明 |
---|---|
1 | 应用程序包文件夹 包管理器将应用程序的所有相关文件安装到应用程序包文件夹中,应用程序只能从该文件夹中读取数据。 |
2 | 本地文件夹 应用程序将本地数据存储到本地文件夹中。它可以存储数据达到存储设备的限制。 |
3 | 漫游文件夹 与应用程序相关的设置和属性存储在漫游文件夹中。其他设备也可以访问此文件夹中的数据。每个应用程序的大小限制为 100KB。 |
4 | 临时文件夹 使用临时存储,并不能保证当您的应用程序再次运行时它仍然可用。 |
5 | 出版商分享 来自同一发布者的所有应用程序的共享存储。它在应用程序清单中声明。 |
6 | 凭证储物柜 用于密码凭证对象的安全存储。 |
7 | 一个驱动器 OneDrive 是 Microsoft 帐户附带的免费在线存储。 |
8 | 云 将数据存储在云端。 |
9 | 已知文件夹 这些文件夹是已知的文件夹,例如“我的图片”、“视频”和“音乐”。 |
10 | 移动存储 USB 存储设备或外部硬盘驱动器等 |
文件处理 API
在 Windows 8 中,引入了新的 API 用于文件处理。这些 API 位于Windows.Storage和Windows.Storage.Streams命名空间中。您可以使用这些 API 而不是System.IO.IsolatedStorage命名空间。通过使用这些 API,您可以更轻松地将 Windows Phone 应用程序移植到 Windows 应用商店,并且您可以轻松地将应用程序升级到未来版本的 Windows。
要访问本地、漫游或临时文件夹,您需要调用这些 API -
StorageFolder localFolder = ApplicationData.Current.LocalFolder; StorageFolder roamingFolder = ApplicationData.Current.RoamingFolder; StorageFolder tempFolder = ApplicationData.Current.TemporaryFolder;
要在本地文件夹中创建新文件,请使用以下代码 -
StorageFolder localFolder = ApplicationData.Current.LocalFolder; StorageFile textFile = await localFolder.CreateFileAsync(filename, CreationCollisionOption.ReplaceExisting);
以下是打开新创建的文件并在该文件中写入一些内容的代码。
using (IRandomAccessStream textStream = await textFile.OpenAsync(FileAccessMode.ReadWrite)) { using (DataWriter textWriter = new DataWriter(textStream)){ textWriter.WriteString(contents); await textWriter.StoreAsync(); } }
您可以从本地文件夹再次打开同一文件,如下面给出的代码所示。
using (IRandomAccessStream textStream = await textFile.OpenReadAsync()) { using (DataReader textReader = new DataReader(textStream)){ uint textLength = (uint)textStream.Size; await textReader.LoadAsync(textLength); contents = textReader.ReadString(textLength); } }
为了理解数据的读写是如何工作的,让我们看一个简单的例子。下面给出的是添加了不同控件的 XAML 代码。
<Page x:Class = "UWPFileHandling.MainPage" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local = "using:UWPFileHandling" xmlns:d = "http://schemas.microsoft.com/expression/blend/2008" xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable = "d"> <Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}"> <Button x:Name = "readFile" Content = "Read Data From File" HorizontalAlignment = "Left" Margin = "62,518,0,0" VerticalAlignment = "Top" Height = "37" Width = "174" Click = "readFile_Click"/> <TextBox x:FieldModifier = "public" x:Name = "textBox" HorizontalAlignment = "Left" Margin = "58,145,0,0" TextWrapping = "Wrap" VerticalAlignment = "Top" Height = "276" Width = "245"/>. <Button x:Name = "writeFile" Content = "Write Data to File" HorizontalAlignment = "Left" Margin = "64,459,0,0" VerticalAlignment = "Top" Click = "writeFile_Click"/> <TextBlock x:Name = "textBlock" HorizontalAlignment = "Left" Margin = "386,149,0,0" TextWrapping = "Wrap" VerticalAlignment = "Top" Height = "266" Width = "250" Foreground = "#FF6231CD"/> </Grid> </Page>
下面给出了不同事件的 C# 实现,以及用于读取和写入数据到文本文件的FileHelper类的实现。
using System; using System.IO; using System.Threading.Tasks; using Windows.Storage; using Windows.Storage.Streams; using Windows.UI.Xaml; using Windows.UI.Xaml.Controls; // The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=402352&clcid=0x409 namespace UWPFileHandling { /// <summary> /// An empty page that can be used on its own or navigated to within a Frame. /// </summary> public partial class MainPage : Page { const string TEXT_FILE_NAME = "SampleTextFile.txt"; public MainPage(){ this.InitializeComponent(); } private async void readFile_Click(object sender, RoutedEventArgs e) { string str = await FileHelper.ReadTextFile(TEXT_FILE_NAME); textBlock.Text = str; } private async void writeFile_Click(object sender, RoutedEventArgs e) { string textFilePath = await FileHelper.WriteTextFile(TEXT_FILE_NAME, textBox.Text); } } public static class FileHelper { // Write a text file to the app's local folder. public static async Task<string> WriteTextFile(string filename, string contents) { StorageFolder localFolder = ApplicationData.Current.LocalFolder; StorageFile textFile = await localFolder.CreateFileAsync(filename, CreationCollisionOption.ReplaceExisting); using (IRandomAccessStream textStream = await textFile.OpenAsync(FileAccessMode.ReadWrite)){ using (DataWriter textWriter = new DataWriter(textStream)){ textWriter.WriteString(contents); await textWriter.StoreAsync(); } } return textFile.Path; } // Read the contents of a text file from the app's local folder. public static async Task<string> ReadTextFile(string filename) { string contents; StorageFolder localFolder = ApplicationData.Current.LocalFolder; StorageFile textFile = await localFolder.GetFileAsync(filename); using (IRandomAccessStream textStream = await textFile.OpenReadAsync()){ using (DataReader textReader = new DataReader(textStream)){ uint textLength = (uint)textStream.Size; await textReader.LoadAsync(textLength); contents = textReader.ReadString(textLength); } } return contents; } } }
编译并执行上述代码后,您将看到以下窗口。

现在,您在文本框中写入一些内容,然后单击“将数据写入文件”按钮。该程序会将数据写入本地文件夹中的文本文件中。如果单击“从文件读取数据”按钮,程序将从位于本地文件夹中的同一文本文件中读取数据并将其显示在文本块上。
