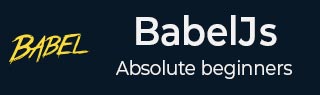
- BabelJs 教程
- BabelJs - 主页
- BabelJs - 概述
- BabelJs - 环境设置
- BabelJs-CLI
- BabelJs - ES6 代码执行
- BabelJs - 使用 Babel 6 进行项目设置
- BabelJs - 使用 Babel 7 进行项目设置
- 将 ES6 功能转换为 ES5
- 将 ES6 模块转译为 ES5
- 将 ES7 功能转换为 ES5
- 将 ES8 功能转译为 ES5
- BabelJs - Babel 插件
- BabelJs - Babel Polyfill
- BabelJs - Babel CLI
- BabelJs - Babel 预设
- 使用 Babel 和 Webpack
- 使用 Babel 和 JSX
- 使用 Babel 和 Flow
- 使用 BabelJS 和 Gulp
- BabelJs - 示例
- BabelJs 有用的资源
- BabelJs - 快速指南
- BabelJs - 有用的资源
- BabelJs - 讨论
BabelJS - Babel 插件
BabelJS 是一个 JavaScript 编译器,它根据可用的预设和插件更改给定代码的语法。babel 编译流程涉及以下 3 个部分 -
- 解析
- 转变
- 印刷
提供给 babel 的代码按原样返回,只是更改了语法。我们已经看到预设被添加到 .babelrc 文件中,以将代码从 es6 编译到 es5,反之亦然。预设只不过是一组插件。如果编译期间未给出预设或插件详细信息,Babel 将不会更改任何内容。
现在让我们讨论以下插件 -
- 变换类属性
- 变换求幂运算符
- for-of
- 物体静止和伸展
- 异步/等待
现在,我们将创建一个项目设置并处理几个插件,这将使您清楚地了解 babel 中插件的需求。
命令
npm init
我们必须安装 babel 所需的软件包 – babel cli、babel core、babel-preset 等。
babel 6 的软件包
npm install babel-cli babel-core babel-preset-es2015 --save-dev
babel 7 的软件包
npm install @babel/cli @babel/core @babel/preset-env --save-dev
在你的项目中创建一个js文件并编写你的js代码。
类 - 转换类属性
为此目的,请遵守下面给出的代码 -
例子
main.js
class Person { constructor(fname, lname, age, address) { this.fname = fname; this.lname = lname; this.age = age; this.address = address; } get fullname() { return this.fname + "-" + this.lname; } } var a = new Person("Siya", "Kapoor", "15", "Mumbai"); var persondet = a.fullname;
目前,我们还没有向 babel 提供任何预设或插件详细信息。如果我们碰巧使用命令转译代码 -
npx babel main.js --out-file main_out.js
main_out.js
class Person { constructor(fname, lname, age, address) { this.fname = fname; this.lname = lname; this.age = age; this.address = address; } get fullname() { return this.fname + "-" + this.lname; } } var a = new Person("Siya", "Kapoor", "15", "Mumbai"); var persondet = a.fullname;
我们将得到原样的代码。现在让我们将预设添加到.babelrc文件中。
注意-在项目的根文件夹中创建.babelrc文件。
.babelrc 用于 babel 6

.babelrc 为 babel 7
{ "presets":["@babel/env"] }
我们已经安装了预设;现在让我们再次运行命令 -
npx babel main.js --out-file main_out.js
main_out.js
"use strict"; var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }(); function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } var Person = function () { function Person(fname, lname, age, address) { _classCallCheck(this, Person); this.fname = fname; this.lname = lname; this.age = age; this.address = address; } _createClass(Person, [{ key: "fullname", get: function get() { return this.fname + "-" + this.lname; } }]); return Person; }(); var a = new Person("Siya", "Kapoor", "15", "Mumbai"); var persondet = a.fullname;
在ES6中,类语法如下
class Person { constructor(fname, lname, age, address) { this.fname = fname; this.lname = lname; this.age = age; this.address = address; } get fullname() { return this.fname + "-" + this.lname; } }
有构造函数,类的所有属性都在其中定义。如果我们需要在类之外定义类属性,我们就不能这样做。
例子
class Person { name = "Siya Kapoor"; fullname = () => { return this.name; } } var a = new Person(); var persondet = a.fullname(); console.log("%c"+persondet, "font-size:25px;color:red;");
如果我们碰巧编译上面的代码,它会在 babel 中抛出错误。这会导致代码无法编译。
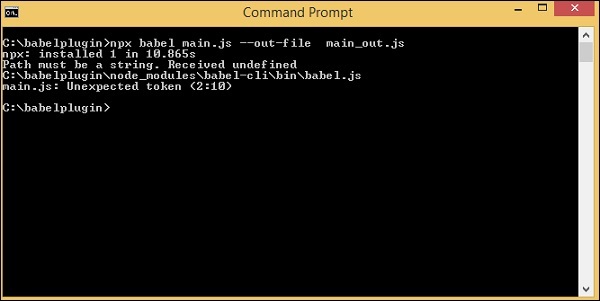
为了按照我们想要的方式工作,我们可以使用名为 babel-plugin-transform-class-properties 的 babel 插件。为了使其工作,我们需要首先安装它,如下所示 -
babel 6 的软件包
npm install --save-dev babel-plugin-transform-class-properties
babel 7 包
npm install --save-dev @babel/plugin-proposal-class-properties
将插件添加到 babel 6 的 .babelrc 文件中-
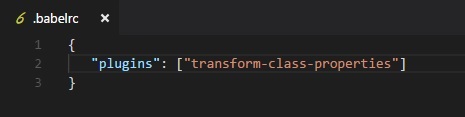
.babelrc 为 babel 7
{ "plugins": ["@babel/plugin-proposal-class-properties"] }
现在,我们将再次运行该命令。
命令
npx babel main.js --out-file main_out.js
main.js
class Person { name = "Siya Kapoor"; fullname = () => { return this.name; } } var a = new Person(); var persondet = a.fullname(); console.log("%c"+persondet, "font-size:25px;color:red;");
编译为main_out.js
class Person { constructor() { this.name = "Siya Kapoor"; this.fullname = () => { return this.name; }; } } var a = new Person(); var persondet = a.fullname(); console.log("%c"+persondet, "font-size:25px;color:red;");
输出
以下是我们在浏览器中使用时得到的输出 -

求幂运算符 - 变换求幂运算符
** 是 ES7 中用于求幂的运算符。以下示例显示了 ES7 中的相同工作原理。它还展示了如何使用 babeljs 转译代码。
例子
let sqr = 9 ** 2; console.log("%c"+sqr, "font-size:25px;color:red;");
要转译幂运算符,我们需要安装一个插件,如下所示 -
babel 6 的软件包
npm install --save-dev babel-plugin-transform-exponentiation-operator
babel 7 的软件包
npm install --save-dev @babel/plugin-transform-exponentiation-operator
对于 babel 6 ,将插件详细信息添加到.babelrc文件中,如下所示 -
{ "plugins": ["transform-exponentiation-operator"] }
.babelrc 为 babel 7
{ "plugins": ["@babel/plugin-transform-exponentiation-operator"] }
命令
npx babel exponeniation.js --out-file exponeniation_out.js
exponiation_out.js
let sqr = Math.pow(9, 2); console.log("%c" + sqr, "font-size:25px;color:red;");
输出
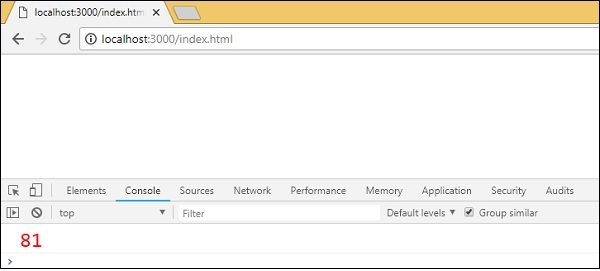
for-of
babel6 和 7 中的插件所需的包如下 -
通天塔6
npm install --save-dev babel-plugin-transform-es2015-for-of
巴别塔 7
npm install --save-dev @babel/plugin-transform-for-of
.babelrc 用于 babel6
{ "plugins": ["transform-es2015-for-of"] }
babel7 的 .babelrc
{ "plugins": ["@babel/plugin-transform-for-of"] }
forof.js
let foo = ["PHP", "C++", "Mysql", "JAVA"]; for (var i of foo) { console.log(i); }
命令
npx babel forof.js --out-file forof_es5.js
Forof_es5.js
let foo = ["PHP", "C++", "Mysql", "JAVA"]; var _iteratorNormalCompletion = true; var _didIteratorError = false; var _iteratorError = undefined; try { for (var _iterator = foo[Symbol.iterator](), _step; !(_iteratorNormalCompletion = (_step = _iterator.next()).done); _iteratorNormalCompletion = true) { var i = _step.value; console.log(i); } } catch (err) { _didIteratorError = true; _iteratorError = err; } finally { try { if (!_iteratorNormalCompletion && _iterator.return) { _iterator.return(); } } finally { if (_didIteratorError) { throw _iteratorError; } } }
输出

物体静止传播
babel6 和 7 中的插件所需的包如下 -
通天塔 6
npm install --save-dev babel-plugin-transform-object-rest-spread
巴别塔 7
npm install --save-dev @babel/plugin-proposal-object-rest-spread
.babelrc 用于 babel6
{ "plugins": ["transform-object-rest-spread"] }
babel7 的 .babelrc
{ "plugins": ["@babel/plugin-proposal-object-rest-spread"] }
js
let { x1, y1, ...z1 } = { x1: 11, y1: 12, a: 23, b: 24 }; console.log(x1); console.log(y1); console.log(z1); let n = { x1, y1, ...z1}; console.log(n);
命令
npx babel o.js --out-file o_es5.js
o_es5.js
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; }; function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; } let _x1$y1$a$b = { x1: 11, y1: 12, a: 23, b: 24 }, { x1, y1 } = _x1$y1$a$b, z1 = _objectWithoutProperties(_x1$y1$a$b, ["x1", "y1"]); console.log(x1); console.log(y1); console.log(z1); let n = _extends({ x1, y1 }, z1); console.log(n);
输出
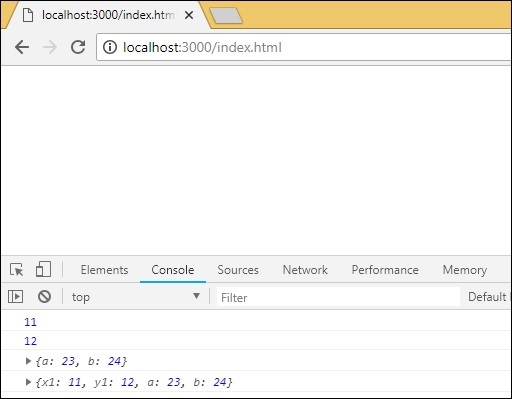
异步/等待
我们需要为 babel 6 安装以下软件包 -
npm install --save-dev babel-plugin-transform-async-to-generator
babel 7 的软件包
npm install --save-dev @babel/plugin-transform-async-to-generator
.babelrc 用于 babel 6
{ "plugins": ["transform-async-to-generator"] }
.babelrc 为 babel 7
{ "plugins": ["@babel/plugin-transform-async-to-generator"] }
异步js
let timer = () => { return new Promise(resolve => { setTimeout(() => { resolve("Promise resolved after 5 seconds"); }, 5000); }); }; let out = async () => { let msg = await timer(); console.log(msg); console.log("hello after await"); }; out();
命令
npx babel async.js --out-file async_es5.js
async_es5.js
function _asyncToGenerator(fn) { return function () { var gen = fn.apply(this, arguments); return new Promise(function (resolve, reject) { function step(key, arg) { try { var info = gen[key](arg); var value = info.value; } catch (error) { reject(error); return; } if (info.done) { resolve(value); } else { return Promise.resolve(value).then(function (value) { step("next", value); }, function (err) { step("throw", err); }); } } return step("next"); }); }; } let timer = () => { return new Promise(resolve => { setTimeout(() => { resolve("Promise resolved after 5 seconds"); }, 5000); }); }; let out = (() => { var _ref = _asyncToGenerator(function* () { let msg = yield timer(); console.log(msg); console.log("hello after await"); }); return function out() { return _ref.apply(this, arguments); }; })(); out();
我们必须使用polyfill来达到同样的目的,因为它在不支持promise的浏览器中不起作用。
输出
