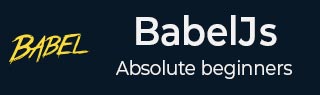
- BabelJs 教程
- BabelJs - 主页
- BabelJs - 概述
- BabelJs - 环境设置
- BabelJs-CLI
- BabelJs - ES6 代码执行
- BabelJs - 使用 Babel 6 进行项目设置
- BabelJs - 使用 Babel 7 进行项目设置
- 将 ES6 功能转换为 ES5
- 将 ES6 模块转译为 ES5
- 将 ES7 功能转换为 ES5
- 将 ES8 功能转译为 ES5
- BabelJs - Babel 插件
- BabelJs - Babel Polyfill
- BabelJs - Babel CLI
- BabelJs - Babel 预设
- 使用 Babel 和 Webpack
- 使用 Babel 和 JSX
- 使用 Babel 和 Flow
- 使用 BabelJS 和 Gulp
- BabelJs - 示例
- BabelJs 有用的资源
- BabelJs - 快速指南
- BabelJs - 有用的资源
- BabelJs - 讨论
BabelJS - 将 ES7 功能转换为 ES5
在本章中,我们将学习如何将 ES7 功能转换为 ES5。
ECMA Script 7 添加了以下新功能 -
- 异步等待
- 求幂运算符
- Array.prototype.includes()
我们将使用 babeljs 将它们编译为 ES5。根据您的项目要求,还可以编译任何 ecma 版本的代码,即 ES7 到 ES6 或 ES7 到 ES5。由于 ES5 版本是最稳定的并且在所有现代和旧浏览器上都可以正常工作,因此我们将代码编译为 ES5。
异步等待
Async 是一个异步函数,它返回一个隐式的 Promise。该承诺要么得到解决,要么被拒绝。异步函数与普通标准函数相同。该函数可以有await表达式,它会暂停执行直到它返回一个promise,一旦它得到它,执行就会继续。仅当函数是异步的时,Await 才会起作用。
这是一个关于 async 和 wait 的工作示例。
例子
let timer = () => { return new Promise(resolve => { setTimeout(() => { resolve("Promise resolved after 5 seconds"); }, 5000); }); }; let out = async () => { let msg = await timer(); console.log(msg); console.log("hello after await"); }; out();
输出
Promise resolved after 5 seconds hello after await
在调用计时器函数之前添加await 表达式。计时器函数将在 5 秒后返回承诺。因此,await 将停止执行,直到计时器函数上的承诺得到解决或拒绝,然后继续。
现在让我们使用 babel 将上面的代码转译为 ES5。
ES7 - 异步等待
let timer = () => { return new Promise(resolve => { setTimeout(() => { resolve("Promise resolved after 5 seconds"); }, 5000); }); }; let out = async () => { let msg = await timer(); console.log(msg); console.log("hello after await"); }; out();
命令
npx babel asyncawait.js --out-file asyncawait_es5.js
BabelJS-ES5
"use strict"; var timer = function timer() { return new Promise(function (resolve) { setTimeout(function () { resolve("Promise resolved after 5 seconds"); }, 5000); }); }; var out = async function out() { var msg = await timer(); console.log(msg); console.log("hello after await"); }; out();
Babeljs 不编译对象或方法;所以这里使用的承诺不会被转译,并会按原样显示。为了在旧浏览器上支持 Promise,我们需要添加代码来支持 Promise。现在,让我们安装 babel-polyfill,如下所示 -
npm install --save babel-polyfill
它应该保存为依赖项而不是开发依赖项。
为了在浏览器中运行代码,我们将使用来自node_modules\babel-polyfill\dist\polyfill.min.js的polyfill文件,并使用脚本标签调用它,如下所示 -
<!DOCTYPE html> <html> <head> <title>BabelJs Testing</title> </head> <body> <script src="node_modules\babel-polyfill\dist\polyfill.min.js" type="text/javascript"></script> <script type="text/javascript" src="aynscawait_es5.js"></script> </body> </html>
当您运行上面的测试页面时,您将在控制台中看到如下所示的输出

求幂运算符
** 是 ES7 中用于求幂的运算符。以下示例显示了 ES7 中的相同工作原理,并且代码使用 babeljs 进行了转译。
例子
let sqr = 9 ** 2; console.log(sqr);
输出
81
ES6 - 求幂
let sqr = 9 ** 2; console.log(sqr);
要转译幂运算符,我们需要安装一个插件,安装如下 -
命令
npm install --save-dev babel-plugin-transform-exponentiation-operator
将插件详细信息添加到.babelrc文件中,如下所示 -
{ "presets":[ "es2015" ], "plugins": ["transform-exponentiation-operator"] }
命令
npx babel exponeniation.js --out-file exponeniation_es5.js
BabelJS-ES5
"use strict"; var sqr = Math.pow(9, 2); console.log(sqr);
Array.prototype.includes()
如果传递给它的元素存在于数组中,则此功能给出 true,否则给出 false。
例子
let arr1 = [10, 6, 3, 9, 17]; console.log(arr1.includes(9)); let names = ['Siya', 'Tom', 'Jerry', 'Bean', 'Ben']; console.log(names.includes('Tom')); console.log(names.includes('Be'));
输出
true true false
我们必须在这里再次使用 babel-polyfill,因为includes是数组上的一个方法,并且它不会被转译。我们需要额外的步骤来包含polyfill,以使其在旧版浏览器中工作。
ES6 - 数组.includes
let arr1 = [10, 6, 3, 9, 17]; console.log(arr1.includes(9)); let names = ['Siya', 'Tom', 'Jerry', 'Bean', 'Ben']; console.log(names.includes('Tom')); console.log(names.includes('Be'));
命令
npx babel array_include.js --out-file array_include_es5.js
巴别塔-ES5
'use strict'; var arr1 = [10, 6, 3, 9, 17]; console.log(arr1.includes(9)); var names = ['Siya', 'Tom', 'Jerry', 'Bean', 'Ben']; console.log(names.includes('Tom')); console.log(names.includes('Be'));
为了在旧版浏览器中测试它,我们需要使用polyfill,如下所示 -
<!DOCTYPE html> <html> <head> <title>BabelJs Testing</title> </head> <body> <script src="node_modules\babel-polyfill\dist\polyfill.min.js" type="text/javascript"></script> <script type="text/javascript" src="array_include_es5.js"></script> </body> </html>
输出
