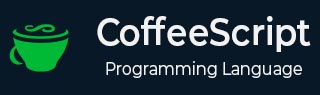
- CoffeeScript 教程
- CoffeeScript - 主页
- CoffeeScript - 概述
- CoffeeScript - 环境
- CoffeeScript - 命令行实用程序
- CoffeeScript - 语法
- CoffeeScript - 数据类型
- CoffeeScript - 变量
- CoffeeScript - 运算符和别名
- CoffeeScript - 条件
- CoffeeScript - 循环
- CoffeeScript - 理解
- CoffeeScript - 函数
- CoffeeScript 面向对象
- CoffeeScript - 字符串
- CoffeeScript - 数组
- CoffeeScript - 对象
- CoffeeScript - 范围
- CoffeeScript - Splat
- CoffeeScript - 日期
- CoffeeScript - 数学
- CoffeeScript - 异常处理
- CoffeeScript - 正则表达式
- CoffeeScript - 类和继承
- CoffeeScript 高级版
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript-MongoDB
- CoffeeScript-SQLite
- CoffeeScript 有用资源
- CoffeeScript - 快速指南
- CoffeeScript - 有用的资源
- CoffeeScript - 讨论
CoffeeScript - 理解
在上一章中,我们学习了 CoffeeScript 提供的各种循环、while及其变体。除此之外,CoffeeScript 还提供了称为推导式的附加循环结构。
如果我们显式添加可选的保护子句和当前数组索引的值,这些推导式将取代其他编程语言中的for循环。使用推导式,我们可以迭代数组和对象,迭代数组的推导式是表达式,我们可以在函数中返回它们或分配给变量。
编号 | 声明及说明 |
---|---|
1 | for..in 推导式
for..in理解是 CoffeeScript 中理解的基本形式,使用它我们可以迭代列表或数组的元素。 |
2 | for..of 理解
就像数组一样,CoffeeScriptScript 提供了一个容器来存储称为对象的键值对。我们可以使用CoffeeScript 提供的for..of推导式来迭代对象。 |
3 | 列表推导式
CoffeeScript 中的列表推导式用于将对象数组映射到另一个数组。 |
理解索引
元素列表/数组有一个可用于推导式的索引。您可以使用变量在推导式中使用它,如下所示。
for student,i in [element1, element2, element3]
例子
以下示例演示了CoffeeScript 中for...in理解式索引的用法。将此代码保存在名为for_in_index.coffee的文件中
for student,i in ['Ram', 'Mohammed', 'John'] console.log "The name of the student with id "+i+" is: "+student
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c for_in_index.coffee
编译时,它会给出以下 JavaScript。
// Generated by CoffeeScript 1.10.0 (function() { var i, j, len, ref, student; ref = ['Ram', 'Mohammed', 'John']; for (i = j = 0, len = ref.length; j < len; i = ++j) { student = ref[i]; console.log("The name of the student with id " + i + " is: " + student); } }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:\> coffee for_in_index.coffee
执行时,CoffeeScript 文件会产生以下输出。
The name of the student with id 0 is: Ram The name of the student with id 1 is: Mohammed The name of the student with id 2 is: John
推导式的后缀形式
就像后缀if和except 一样,CoffeeScript 提供了推导式的后缀形式,这在编写代码时非常方便。使用它,我们可以在一行中编写for..in理解,如下所示。
#Postfix for..in comprehension console.log student for student in ['Ram', 'Mohammed', 'John'] #postfix for..of comprehension console.log key+"::"+value for key,value of { name: "Mohammed", age: 24, phone: 9848022338}显示示例
分配给变量
我们用来迭代数组的推导式可以分配给一个变量,也可以由一个函数返回。
例子
考虑下面给出的例子。在这里您可以观察到我们已经使用for..in理解检索了数组的元素并将其分配给名为名称的变量。我们还有一个使用return关键字显式返回推导式的函数。将此代码保存在名为example.coffee的文件中
my_function =-> student = ['Ram', 'Mohammed', 'John'] #Assigning comprehension to a variable names = (x for x in student ) console.log "The contents of the variable names are ::"+names #Returning the comprehension return x for x in student console.log "The value returned by the function is "+my_function()
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c example.coffee
编译时,它会给出以下 JavaScript。
// Generated by CoffeeScript 1.10.0 (function() { var my_function; my_function = function() { var i, len, names, student, x; student = ['Ram', 'Mohammed', 'John']; names = (function() { var i, len, results; results = []; for (i = 0, len = student.length; i < len; i++) { x = student[i]; results.push(x); } return results; })(); console.log("The contents of the variable names are ::" + names); for (i = 0, len = student.length; i < len; i++) { x = student[i]; return x; } }; console.log("The value returned by the function is " + my_function()); }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:\> coffee example.coffee
执行时,CoffeeScript 文件会产生以下输出。
The contents of the variable names are ::Ram,Mohammed,John The value returned by the function is Ram
by 关键字
CoffeeScript 提供范围来定义元素列表。例如,范围 [1..10] 相当于 [1, 2, 3, 4, 5, 6, 7, 8, 9, 10],其中每个元素都递增 1。我们也可以更改此增量使用推导式的by关键字。
例子
以下示例演示了CoffeeScript 提供的for..in理解中by关键字的用法。将此代码保存在名为by_keyword_example.coffee的文件中
array = (num for num in [1..10] by 2) console.log array
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c by_keyword_example.coffee
编译时,它会给出以下 JavaScript。
// Generated by CoffeeScript 1.10.0 (function() { var array, num; array = (function() { var i, results; results = []; for (num = i = 1; i <= 10; num = i += 2) { results.push(num); } return results; })(); console.log(array); }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:\> coffee by_keyword_example.coffee
执行时,CoffeeScript 文件会产生以下输出。
[ 1, 3, 5, 7, 9 ]