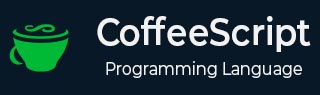
- CoffeeScript 教程
- CoffeeScript - 主页
- CoffeeScript - 概述
- CoffeeScript - 环境
- CoffeeScript - 命令行实用程序
- CoffeeScript - 语法
- CoffeeScript - 数据类型
- CoffeeScript - 变量
- CoffeeScript - 运算符和别名
- CoffeeScript - 条件
- CoffeeScript - 循环
- CoffeeScript - 理解
- CoffeeScript - 函数
- CoffeeScript 面向对象
- CoffeeScript - 字符串
- CoffeeScript - 数组
- CoffeeScript - 对象
- CoffeeScript - 范围
- CoffeeScript - Splat
- CoffeeScript - 日期
- CoffeeScript - 数学
- CoffeeScript - 异常处理
- CoffeeScript - 正则表达式
- CoffeeScript - 类和继承
- CoffeeScript 高级版
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript-MongoDB
- CoffeeScript-SQLite
- CoffeeScript 有用资源
- CoffeeScript - 快速指南
- CoffeeScript - 有用的资源
- CoffeeScript - 讨论
CoffeeScript - 异常处理
异常(或异常事件)是程序执行过程中出现的问题。当异常发生时,程序的正常流程被打乱,程序/应用程序异常终止,这是不推荐的,因此需要对这些异常进行处理。
异常的发生可能有多种原因。以下是一些发生异常的场景。
- 用户输入了无效数据。
- 找不到需要打开的文件。
CoffeeScript 中的异常
CoffeeScripts 支持使用try catch 和 finally块进行异常/错误处理。这些块的功能与 JavaScript 中相同,try块保存异常语句,catch块具有异常发生时要执行的操作,finally块用于无条件执行语句。
以下是CoffeeScript 中try catch和finally块的语法。
try // Code to run catch ( e ) // Code to run if an exception occurs finally // Code that is always executed regardless of // an exception occurring
try块后面必须紧跟一个catch块或一个finally块(或两者之一)。当try块中发生异常时,异常被放置在e中并执行catch块。可选的finally块在try/catch 之后无条件执行。
例子
以下示例演示了在 CoffeeScript 中使用 try 和 catch 块进行异常处理。在这里,我们尝试在 CoffeeScript 操作中使用未定义的符号,并使用try和catch块处理发生的错误。将此代码保存在名为Exception_handling.coffee的文件中
try x = y+20 console.log "The value of x is :" +x catch e console.log "exception/error occurred" console.log "The STACKTRACE for the exception/error occurred is ::" console.log e.stack
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c Exception_handling.coffee
编译时,它会给出以下 JavaScript。
// Generated by CoffeeScript 1.10.0 (function() { var e, error, x; try { x = y + 20; console.log("The value of x is :" + x); } catch (error) { e = error; console.log("exception/error occurred"); console.log("The STACKTRACE for the exception/error occurred is ::"); console.log(e.stack); } }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:\> coffee Exception_handling.coffee
执行时,CoffeeScript 文件会产生以下输出。
exception/error occurred The STACKTRACE for the exception/error occurred is :: ReferenceError: y is not defined at Object.<anonymous> (C:\Examples\strings_exceptions\Exception_handling.coffee:3:7) at Object.<anonymous> (C:\Examples\strings_exceptions\Exception_handling.coffee:2:1) at Module._compile (module.js:413:34) at Object.exports.run (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\lib\coffee-script\coffee-script.js:134:23) at compileScript (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\lib\coffee-script\command.js:224:29) at compilePath (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\lib\coffee-script\command.js:174:14) at Object.exports.run (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\lib\coffee-script\command.js:98:20) at Object.<anonymous> (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\bin\coffee:7:41) at Module._compile (module.js:413:34) at Object.Module._extensions..js (module.js:422:10) at Module.load (module.js:357:32) at Function.Module._load (module.js:314:12) at Function.Module.runMain (module.js:447:10) at startup (node.js:139:18) at node.js:999:3
最后块
我们还可以使用finally块重写上面的例子。如果我们这样做,该块的内容将在try和catch之后无条件执行。将此代码保存在名为Exception_handling_finally.coffee的文件中
try x = y+20 console.log "The value of x is :" +x catch e console.log "exception/error occurred" console.log "The STACKTRACE for the exception/error occurred is ::" console.log e.stack finally console.log "This is the statement of finally block"
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c Exception_handling_finally.coffee
编译时,它会给出以下 JavaScript。
// Generated by CoffeeScript 1.10.0 (function() { var e, error, x; try { x = y + 20; console.log("The value of x is :" + x); } catch (error) { e = error; console.log("exception/error occurred"); console.log("The STACKTRACE for the exception/error occurred is ::"); console.log(e.stack); } finally { console.log("This is the statement of finally block"); } }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:\> coffee Exception_handling_finally.coffee
执行时,CoffeeScript 文件会产生以下输出。
exception/error occurred The STACKTRACE for the exception/error occurred is :: ReferenceError: y is not defined at Object.<anonymous> (C:\Examples\strings_exceptions\Exception_handling.coffee:3:7) at Object.<anonymous> (C:\Examples\strings_exceptions\Exception_handling.coffee:2:1) at Module._compile (module.js:413:34) at Object.exports.run (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\lib\coffee-script\coffee-script.js:134:23) at compileScript (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\lib\coffee-script\command.js:224:29) at compilePath (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\lib\coffee-script\command.js:174:14) at Object.exports.run (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\lib\coffee-script\command.js:98:20) at Object.<anonymous> (C:\Users\Tutorialspoint\AppData\Roaming\npm\node_modules\coffee-script\bin\coffee:7:41) at Module._compile (module.js:413:34) at Object.Module._extensions..js (module.js:422:10) at Module.load (module.js:357:32) at Function.Module._load (module.js:314:12) at Function.Module.runMain (module.js:447:10) at startup (node.js:139:18) at node.js:999:3 This is the statement of finally block
抛出语句
CoffeeScript 还支持throw语句。您可以使用 throw 语句引发内置异常或自定义异常。稍后可以捕获这些异常,您可以采取适当的操作。
例子
以下示例演示了CoffeeScript 中throw语句的用法。将此代码保存在名为throw_example.coffee的文件中
myFunc = -> a = 100 b = 0 try if b == 0 throw ("Divided by zero error.") else c = a / b catch e console.log "Error: " + e myFunc()
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c throw_example.coffee
编译时,它会给出以下 JavaScript。
// Generated by CoffeeScript 1.10.0 (function() { var myFunc; myFunc = function() { var a, b, c, e, error; a = 100; b = 0; try { if (b === 0) { throw "Divided by zero error."; } else { return c = a / b; } } catch (error) { e = error; return console.log("Error: " + e); } }; myFunc(); }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:\> coffee throw_example.coffee
执行时,CoffeeScript 文件会产生以下输出。
Divided by zero error.