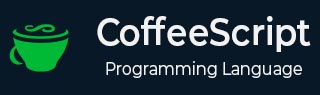
- CoffeeScript 教程
- CoffeeScript - 主页
- CoffeeScript - 概述
- CoffeeScript - 环境
- CoffeeScript - 命令行实用程序
- CoffeeScript - 语法
- CoffeeScript - 数据类型
- CoffeeScript - 变量
- CoffeeScript - 运算符和别名
- CoffeeScript - 条件
- CoffeeScript - 循环
- CoffeeScript - 理解
- CoffeeScript - 函数
- CoffeeScript 面向对象
- CoffeeScript - 字符串
- CoffeeScript - 数组
- CoffeeScript - 对象
- CoffeeScript - 范围
- CoffeeScript - Splat
- CoffeeScript - 日期
- CoffeeScript - 数学
- CoffeeScript - 异常处理
- CoffeeScript - 正则表达式
- CoffeeScript - 类和继承
- CoffeeScript 高级版
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript-MongoDB
- CoffeeScript-SQLite
- CoffeeScript 有用资源
- CoffeeScript - 快速指南
- CoffeeScript - 有用的资源
- CoffeeScript - 讨论
CoffeeScript - 数学
JavaScript 的Math对象为您提供数学常量和函数的属性和方法。与其他全局对象不同,Math不是构造函数。Math的所有属性和方法都是静态的,可以将Math作为对象来调用,而无需创建它。
因此,您将常量pi称为Math.PI,并将正弦函数称为Math.sin(x),其中 x 是该方法的参数。我们可以在 CoffeeScript 代码中使用 JavaScript 的 Math 对象来执行数学运算。
数学常数
如果我们想使用任何常见的数学常量,例如 pi 或 e,我们可以使用 JavaScript 的Math对象来使用它们。
以下是 JavaScript Math 对象提供的 Math 常量列表
编号 | 属性及描述 |
---|---|
1 | 乙 欧拉常数和自然对数的底,大约为 2.718。 |
2 | 液氮2 2 的自然对数,约为 0.693。 |
3 | 液氮10 10 的自然对数,约为 2.302。 |
4 | 对数2E E 以 2 为底的对数,约为 1.442。 |
5 | 日志10E E 以 10 为底的对数,约为 0.434。 |
6 | PI 圆的周长与其直径的比值,大约为 3.14159。 |
7 | SQRT1_2 1/2 的平方根;等价地,1 除以 2 的平方根,大约为 0.707。 |
8 | 平方根RT2
2 的平方根,约为 1.414。 |
例子
以下示例演示了 JavaScript 提供的数学常量在 CoffeeScript 中的用法。将此代码保存在名为math_example.coffee的文件中
e_value = Math.E console.log "The value of the constant E is: " + e_value LN2_value = Math.LN2 console.log "The value of the constant LN2 is: " + LN2_value LN10_value = Math.LN10 console.log "The value of the constant LN10 is: " + LN10_value LOG2E_value = Math.LOG2E console.log "The value of the constant LOG2E is: " + LOG2E_value LOG10E_value = Math.LOG10E console.log "The value of the constant LOG10E is: " + LOG10E_value PI_value = Math.PI console.log "The value of the constant PI is: " + PI_value SQRT1_2_value = Math.SQRT1_2 console.log "The value of the constant SQRT1_2 is: " + SQRT1_2_value SQRT2_value = Math.SQRT2 console.log "The value of the constant SQRT2 is: " + SQRT2_value
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c math_example.coffee
编译时,它会给出以下 JavaScript。
// Generated by CoffeeScript 1.10.0 (function() { var LN10_value, LN2_value, LOG10E_value, LOG2E_value, PI_value, SQRT1_2_value, SQRT2_value, e_value; e_value = Math.E; console.log("The value of the constant E is: " + e_value); LN2_value = Math.LN2; console.log("The value of the constant LN2 is: " + LN2_value); LN10_value = Math.LN10; console.log("The value of the constant LN10 is: " + LN10_value); LOG2E_value = Math.LOG2E; console.log("The value of the constant LOG2E is: " + LOG2E_value); LOG10E_value = Math.LOG10E; console.log("The value of the constant LOG10E is: " + LOG10E_value); PI_value = Math.PI; console.log("The value of the constant PI is: " + PI_value); SQRT1_2_value = Math.SQRT1_2; console.log("The value of the constant SQRT1_2 is: " + SQRT1_2_value); SQRT2_value = Math.SQRT2; console.log("The value of the constant SQRT2 is: " + SQRT2_value); }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:\> coffee math_example.coffee
执行时,CoffeeScript 文件会产生以下输出。
The value of the constant E is: 2.718281828459045 The value of the constant LN2 is: 0.6931471805599453 The value of the constant LN10 is: 2.302585092994046 The value of the constant LOG2E is: 1.4426950408889634 The value of the constant LOG10E is: 0.4342944819032518 The value of the constant PI is: 3.141592653589793 The value of the constant SQRT1_2 is: 0.7071067811865476 The value of the constant SQRT2 is: 1.4142135623730951
数学方法
除了属性之外,Math 对象还提供方法。以下是 JavaScript Math对象的方法列表。单击这些方法的名称即可获取演示它们在 CoffeeScript 中用法的示例。
编号 | 方法及说明 |
---|---|
1 | 绝对值()
返回数字的绝对值。 |
2 | acos()
返回数字的反余弦(以弧度为单位)。 |
3 | 阿辛()
返回数字的反正弦(以弧度为单位)。 |
4 | 晒黑()
返回数字的反正切(以弧度为单位)。 |
5 | 阿坦2()
返回其参数商的反正切值。 |
6 | 天花板()
返回大于或等于数字的最小整数。 |
7 | 余弦()
返回数字的余弦。 |
8 | 指数()
返回 E N,其中 N 是参数,E 是欧拉常数,即自然对数的底数。 |
9 | 地面()
返回小于或等于数字的最大整数。 |
10 | 日志()
返回数字的自然对数(以 E 为底)。 |
11 | 最大限度()
返回零个或多个数字中最大的一个。 |
12 | 分钟()
返回零个或多个数字中的最小值。 |
13 | 战俘()
返回指数幂的底数,即底指数。 |
14 | 随机的()
返回 0 到 1 之间的伪随机数。 |
15 | 圆形的()
返回四舍五入到最接近的整数的数字值。 |
16 | 罪()
返回数字的正弦值。 |
17 号 | 开方()
返回数字的平方根。 |
18 | 正切()
返回数字的正切值。 |