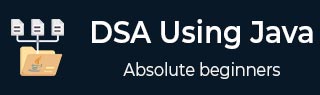
- DSA 使用 Java 教程
- 使用 Java 的 DSA - 主页
- 使用 Java 的 DSA - 概述
- 使用 Java 的 DSA - 环境设置
- 使用 Java 的 DSA - 算法
- 使用 Java 的 DSA - 数据结构
- 使用 Java 的 DSA - 数组
- 使用 Java 的 DSA - 链表
- 使用 Java 的 DSA - 双向链表
- 使用 Java 的 DSA - 循环链表
- 使用Java的DSA - 堆栈内存溢出
- DSA - 解析表达式
- 使用 Java 的 DSA - 队列
- 使用 Java 的 DSA - 优先级队列
- 使用 Java 的 DSA - 树
- 使用 Java 的 DSA - 哈希表
- 使用 Java 的 DSA - 堆
- 使用 Java 的 DSA - 图
- 使用 Java 的 DSA - 搜索技术
- 使用 Java 的 DSA - 排序技术
- 使用 Java 的 DSA - 递归
- 使用 Java 的 DSA 有用资源
- 使用 Java 的 DSA - 快速指南
- 使用 Java 的 DSA - 有用资源
- 使用 Java 的 DSA - 讨论
使用 Java 的 DSA - 图
概述
图是一种对数学图进行建模的数据结构。它由一组称为顶点边的连接对组成。我们可以使用顶点数组和边的二维数组来表示图。
重要条款
顶点- 图的每个节点都表示为一个顶点。在下面给出的示例中,标记的圆圈表示顶点。所以A到G是顶点。我们可以使用数组来表示它们,如下图所示。这里A可以通过索引0来标识。B可以通过索引1来标识,依此类推。
Edge - 边表示两个顶点之间的路径或两个顶点之间的线。在下面给出的示例中,从 A 到 B、B 到 C 等的线代表边缘。我们可以使用二维数组来表示数组,如下图所示。这里AB可以在第0行第1列表示为1,BC在第1行第2列表示为1,依此类推,其他组合保持为0。
邻接- 如果两个节点或顶点通过边相互连接,则它们是相邻的。在下面给出的示例中,B 与 A 相邻,C 与 B 相邻,依此类推。
路径- 路径表示两个顶点之间的边序列。在下面给出的示例中,ABCD 表示从 A 到 D 的路径。
基本操作
以下是图的基本主要操作。
添加顶点- 向图中添加一个顶点。
添加边- 在图的两个顶点之间添加边。
显示顶点- 显示图形的顶点。
添加顶点操作
//add vertex to the array of vertex public void addVertex(char label){ lstVertices[vertexCount++] = new Vertex(label); }
添加边缘操作
//add edge to edge array public void addEdge(int start,int end){ adjMatrix[start][end] = 1; adjMatrix[end][start] = 1; }
显示边缘操作
//display the vertex public void displayVertex(int vertexIndex){ System.out.print(lstVertices[vertexIndex].label+" "); }
遍历算法
以下是图上的重要遍历算法。
深度优先搜索- 以深度运动遍历图形。
广度优先搜索- 以广度运动遍历图。
深度优先搜索算法
深度优先搜索算法(DFS)以深度运动方式遍历图,并在任何迭代中出现死端时使用堆栈来记住获取下一个顶点以开始搜索。
正如上面给出的例子,DFS算法首先从A遍历到B,再到C,再到D,然后到E,然后到F,最后到G。它采用以下规则。
规则 1 - 访问相邻的未访问顶点。标记它已访问过。显示它。将其推入堆栈。
规则 2 - 如果没有找到相邻顶点,则从堆栈中弹出一个顶点。(它将从堆栈中弹出所有没有相邻顶点的顶点。)
规则 3 - 重复规则 1 和规则 2,直到堆栈为空。
public void depthFirstSearch(){ //mark first node as visited lstVertices[0].visited = true; //display the vertex displayVertex(0); //push vertex index in stack stack.push(0); while(!stack.isEmpty()){ //get the unvisited vertex of vertex which is at top of the stack int unvisitedVertex = getAdjUnvisitedVertex(stack.peek()); //no adjacent vertex found if(unvisitedVertex == -1){ stack.pop(); }else{ lstVertices[unvisitedVertex].visited = true; displayVertex(unvisitedVertex); stack.push(unvisitedVertex); } } //stack is empty, search is complete, reset the visited flag for(int i=0;i<vertexCount;i++){ lstVertices[i].visited = false; } }
广度优先搜索算法
广度优先搜索算法(BFS)以广度运动方式遍历图,并在任何迭代中出现死端时使用队列来记住获取下一个顶点以开始搜索。
如上面给出的示例,BFS 算法首先从 A 遍历到 B,再到 E,再到 F,然后再遍历到 C,最后遍历到 D。它采用以下规则。
规则 1 - 访问相邻的未访问顶点。标记它已访问过。显示它。将其插入队列中。
规则 2 - 如果没有找到相邻顶点,则从队列中删除第一个顶点。
规则 3 - 重复规则 1 和规则 2,直到队列为空。
public void breadthFirstSearch(){ //mark first node as visited lstVertices[0].visited = true; //display the vertex displayVertex(0); //insert vertex index in queue queue.insert(0); int unvisitedVertex; while(!queue.isEmpty()){ //get the unvisited vertex of vertex which is at front of the queue int tempVertex = queue.remove(); //no adjacent vertex found while((unvisitedVertex=getAdjUnvisitedVertex(tempVertex)) != -1){ lstVertices[unvisitedVertex].visited = true; displayVertex(unvisitedVertex); queue.insert(unvisitedVertex); } } //queue is empty, search is complete, reset the visited flag for(int i=0;i<vertexCount;i++){ lstVertices[i].visited = false; } }
图实现
堆栈.java
package com.tutorialspoint.datastructure; public class Stack { private int size; // size of the stack private int[] intArray; // stack storage private int top; // top of the stack // Constructor public Stack(int size){ this.size = size; intArray = new int[size]; //initialize array top = -1; //stack is initially empty } // Operation : Push // push item on the top of the stack public void push(int data) { if(!isFull()){ // increment top by 1 and insert data intArray[++top] = data; }else{ System.out.println("Cannot add data. Stack is full."); } } // Operation : Pop // pop item from the top of the stack public int pop() { //retrieve data and decrement the top by 1 return intArray[top--]; } // Operation : Peek // view the data at top of the stack public int peek() { //retrieve data from the top return intArray[top]; } // Operation : isFull // return true if stack is full public boolean isFull(){ return (top == size-1); } // Operation : isEmpty // return true if stack is empty public boolean isEmpty(){ return (top == -1); } }
队列.java
package com.tutorialspoint.datastructure; public class Queue { private final int MAX; private int[] intArray; private int front; private int rear; private int itemCount; public Queue(int size){ MAX = size; intArray = new int[MAX]; front = 0; rear = -1; itemCount = 0; } public void insert(int data){ if(!isFull()){ if(rear == MAX-1){ rear = -1; } intArray[++rear] = data; itemCount++; } } public int remove(){ int data = intArray[front++]; if(front == MAX){ front = 0; } itemCount--; return data; } public int peek(){ return intArray[front]; } public boolean isEmpty(){ return itemCount == 0; } public boolean isFull(){ return itemCount == MAX; } public int size(){ return itemCount; } }
顶点.java
package com.tutorialspoint.datastructure; public class Vertex { public char label; public boolean visited; public Vertex(char label){ this.label = label; visited = false; } }
图.java
package com.tutorialspoint.datastructure; public class Graph { private final int MAX = 20; //array of vertices private Vertex lstVertices[]; //adjacency matrix private int adjMatrix[][]; //vertex count private int vertexCount; private Stack stack; private Queue queue; public Graph(){ lstVertices = new Vertex[MAX]; adjMatrix = new int[MAX][MAX]; vertexCount = 0; stack = new Stack(MAX); queue = new Queue(MAX); for(int j=0; j<MAX; j++) // set adjacency for(int k=0; k<MAX; k++) // matrix to 0 adjMatrix[j][k] = 0; } //add vertex to the vertex list public void addVertex(char label){ lstVertices[vertexCount++] = new Vertex(label); } //add edge to edge array public void addEdge(int start,int end){ adjMatrix[start][end] = 1; adjMatrix[end][start] = 1; } //display the vertex public void displayVertex(int vertexIndex){ System.out.print(lstVertices[vertexIndex].label+" "); } //get the adjacent unvisited vertex public int getAdjUnvisitedVertex(int vertexIndex){ for(int i=0; i<vertexCount; i++) if(adjMatrix[vertexIndex][i]==1 && lstVertices[i].visited==false) return i; return -1; } public void depthFirstSearch(){ //mark first node as visited lstVertices[0].visited = true; //display the vertex displayVertex(0); //push vertex index in stack stack.push(0); while(!stack.isEmpty()){ //get the unvisited vertex of vertex which is at top of the stack int unvisitedVertex = getAdjUnvisitedVertex(stack.peek()); //no adjacent vertex found if(unvisitedVertex == -1){ stack.pop(); }else{ lstVertices[unvisitedVertex].visited = true; displayVertex(unvisitedVertex); stack.push(unvisitedVertex); } } //stack is empty, search is complete, reset the visited flag for(int i=0;i<vertexCount;i++){ lstVertices[i].visited = false; } } public void breadthFirstSearch(){ //mark first node as visited lstVertices[0].visited = true; //display the vertex displayVertex(0); //insert vertex index in queue queue.insert(0); int unvisitedVertex; while(!queue.isEmpty()){ //get the unvisited vertex of vertex which is at front of the queue int tempVertex = queue.remove(); //no adjacent vertex found while((unvisitedVertex=getAdjUnvisitedVertex(tempVertex)) != -1){ lstVertices[unvisitedVertex].visited = true; displayVertex(unvisitedVertex); queue.insert(unvisitedVertex); } } //queue is empty, search is complete, reset the visited flag for(int i=0;i<vertexCount;i++){ lstVertices[i].visited = false; } } }
演示程序
GraphDemo.java
package com.tutorialspoint.datastructure; public class GraphDemo { public static void main(String args[]){ Graph graph = new Graph(); graph.addVertex('A'); //0 graph.addVertex('B'); //1 graph.addVertex('C'); //2 graph.addVertex('D'); //3 graph.addVertex('E'); //4 graph.addVertex('F'); //5 graph.addVertex('G'); //6 /* 1 2 3 * 0 |--B--C--D * A--| * | * | 4 * |-----E * | 5 6 * | |--F--G * |--| */ graph.addEdge(0, 1); //AB graph.addEdge(1, 2); //BC graph.addEdge(2, 3); //CD graph.addEdge(0, 4); //AC graph.addEdge(0, 5); //AF graph.addEdge(5, 6); //FG System.out.print("Depth First Search: "); //A B C D E F G graph.depthFirstSearch(); System.out.println(""); System.out.print("Breadth First Search: "); //A B E F C G D graph.breadthFirstSearch(); } }
如果我们编译并运行上面的程序,那么它将产生以下结果 -
Depth First Search: A B C D E F G Breadth First Search: A B E F C G D