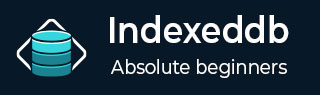
- IndexedDB Tutorial
- IndexedDB - Home
- IndexedDB - Introduction
- IndexedDB - Installation
- IndexedDB - Connection
- IndexedDB - Object Stores
- IndexedDB - Creating Data
- IndexedDB - Reading Data
- IndexedDB - Updating Data
- IndexedDB - Deleting Data
- Using getAll() Functions
- IndexedDB - Indexes
- IndexedDB - Ranges
- IndexedDB - Transactions
- IndexedDB - Error Handling
- IndexedDB - Searching
- IndexedDB - Cursors
- IndexedDB - Promise Wrapper
- IndexedDB - Ecmascript Binding
- IndexedDB Useful Resources
- IndexedDB - Quick Guide
- IndexedDB - Useful Resources
- IndexedDB - Discussion
IndexedDB - 创建数据
在创建数据之前,我们需要知道数据是如何传输的。IndexedDB开启事务,它的每一个数据操作都是在每一个事务内部进行的。每个操作有四个步骤 -
- 获取数据库对象
- 在数据库上打开事务
- 在事务上打开对象存储
- 对对象存储进行操作
IndexedDB 中的操作是 -
- 创造
- 读
- 更新
- 删除
首先,要在数据库中执行任何操作,我们需要打开一个事务。交易打开后,我们需要获取我们需要的对象存储。这些对象存储仅根据创建事务时提到的要求提供。然后可以稍后添加所需的任何数据。
函数用于执行给定的操作(如果有)。例如,我们使用 add() 函数将数据添加到数据库中或添加新条目。
句法
以下是将数据创建到数据库的语法 -
ar request = objectStore.add(data);
我们可以使用add()或put()函数将数据添加到对象存储中。
例子
在下面的示例中,我们使用 JavaScript 中的 add() 方法将数据插入到对象存储中 -
<!DOCTYPE html> <html lang="en"> <head> <title>creating data</title> </head> <body> <script> const dbName = "Database"; var request = indexedDB.open("Database", 2); request.onupgradeneeded = event => { var db = event.target.result; var objectStore = db.createObjectStore("student",{ keyPath :"rollno" } ); }; request.onsuccess = event => { document.write("Database opened successfully"); var db = event.target.result; var transaction = db.transaction("student", "readwrite"); var objectStore = transaction.objectStore("student"); objectStore.add({ rollno: 160218737028, name: "jason", branch: "IT" }); objectStore.add({ rollno: 160218733028, name: "tarun", branch: "EEE" }); objectStore.add({ rollno: 160218732028, name: "lokesh", branch: "CSE" }); objectStore.add({ rollno: 160218737025, name: "abdul", branch: "IT" }); objectStore.add({ rollno: 160218736055, name: "palli", branch: "MECH" }); } transaction.oncomplete = function () { db.close(); }; </script> </body> </html>
输出
0 160218732028 {rollno: 160218732028, name: 'lokesh', branch: 'CSE'} 1 160218733028 {rollno: 160218733028, name: 'tarun', branch: 'EEE'} 2 160218736055 {rollno: 160218736055, name: 'palli', branch: 'CSE'} 3 160218737025 {rollno: 160218737025, name: 'abdul', branch: 'IT'} 4 160218737028 {rollno: 160218737028, name: 'jason', branch: 'IT'}