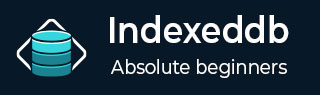
- IndexedDB Tutorial
- IndexedDB - Home
- IndexedDB - Introduction
- IndexedDB - Installation
- IndexedDB - Connection
- IndexedDB - Object Stores
- IndexedDB - Creating Data
- IndexedDB - Reading Data
- IndexedDB - Updating Data
- IndexedDB - Deleting Data
- Using getAll() Functions
- IndexedDB - Indexes
- IndexedDB - Ranges
- IndexedDB - Transactions
- IndexedDB - Error Handling
- IndexedDB - Searching
- IndexedDB - Cursors
- IndexedDB - Promise Wrapper
- IndexedDB - Ecmascript Binding
- IndexedDB Useful Resources
- IndexedDB - Quick Guide
- IndexedDB - Useful Resources
- IndexedDB - Discussion
IndexedDB - 索引
索引是一种对象存储,用于从指定属性存储的引用对象中检索数据。尽管索引位于引用对象存储内部并且包含相同的数据,但它使用指定的属性作为其键路径,而不是引用存储的主键。
索引用于定义数据的唯一约束,它们是在创建对象存储时创建的。要创建索引,请在对象存储实例上调用 createIndex 方法 -
句法
var myIDBIndex = objectStore.createIndex(indexName, keyPath); var myIDBIndex = objectStore.createIndex(indexName, keyPath, objectParameters);
该方法创建并返回一个索引对象。该方法创建一个采用以下参数的索引 -
索引名称- 索引的名称。
Keypath - 我们在这里提到主键。
对象参数- 有两个对象参数。
唯一- 无法添加重复值。
多条目- 如果为 true,则当 keyPath 解析为数组时,索引将为每个数组元素在索引中添加一个条目。如果为 false,它将添加一个包含数组的单个条目。
例子
以下示例显示了对象存储中索引的实现 -
<!DOCTYPE html> <html lang="en"> <head> <title>Document</title> </head> <body> <script> const request = indexedDB.open("botdatabase",1); request.onupgradeneeded = function(){ const db = request.result; const store = db.createObjectStore("bots",{ keyPath: "id"}); store.createIndex("branch_db",["branch"],{unique: false}); } request.onsuccess = function(){ document.write("database opened successfully"); const db = request.result; const transaction=db.transaction("bots","readwrite"); const store = transaction.objectStore("bots"); const branchIndex = store.index("branch_db"); store.add({id: 1, name: "jason",branch: "IT"}); store.add({id: 2, name: "praneeth",branch: "CSE"}); store.add({id: 3, name: "palli",branch: "EEE"}); store.add({id: 4, name: "abdul",branch: "IT"}); store.put({id: 4, name: "deevana",branch: "CSE"}); transaction.oncomplete = function(){ db.close; } } </script> </body> </html>
输出
branchIndex: 1 ['CSE'] 0: "CSE" length: 1 4 {id: 4, name: 'deevana', branch: 'CSE'} branch: "CSE" id: 4 name: "deevana" 2 ['EEE'] 0: "EEE" length: 1 3 {id: 3, name: 'palli', branch: 'EEE'} branch: "EEE" id: 3 name: "palli" 3 ['IT'] 0: "IT" length: 1 1 {id: 1, name: 'jason', branch: 'IT'} branch: "IT" id: 1 name: "jason"