
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX - Environment
- JavaFX - Architecture
- JavaFX - Application
- JavaFX - 2D Shapes
- JavaFX - Text
- JavaFX - Effects
- JavaFX - Transformations
- JavaFX - Animations
- JavaFX - Colors
- JavaFX - Images
- JavaFX - 3D Shapes
- JavaFX - Event Handling
- JavaFX - UI Controls
- JavaFX - Charts
- JavaFX - Layout Panes
- JavaFX - CSS
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - 3D 形状
在前面的章节中,我们已经了解了如何在 XY 平面上绘制 2D 形状。除了这些 2D 形状之外,我们还可以使用 JavaFX 绘制其他几种 3D 形状。
3D形状
一般来说,3D 形状是可以在 XYZ 平面上绘制的几何图形。其中包括圆柱体、球体和盒子。
上述每个 3D 形状都由一个类表示,所有这些类都属于javafx.scene.shape包。名为Shape3D的类是 JavaFX 中所有 3 维形状的基类。
创建 3D 形状
要创建 3 维形状,您需要 -
实例化所需 3D 形状的相应类。
设置 3D 形状的属性。
将 3D 形状对象添加到组中。
实例化相应的类
要创建 3 维形状,首先需要实例化其各自的类。例如,如果要创建 3D 盒子,则需要实例化名为 Box 的类,如下所示 -
Box box = new Box();
设置形状的属性
实例化该类后,您需要使用 setter 方法设置形状的属性。
例如,要绘制 3D 盒子,您需要传递其宽度、高度、深度。您可以使用各自的 setter 方法指定这些值,如下所示 -
//Setting the properties of the Box box.setWidth(200.0); box.setHeight(400.0); box.setDepth(200.0);
将形状对象添加到组中
最后,您需要将形状的对象添加到组中,方法是将其作为构造函数的参数传递,如下所示。
//Creating a Group object Group root = new Group(box);
下表列出了 JavaFX 提供的各种 3D 形状。
序列号 | 形状及描述 |
---|---|
1 |
盒子
长方体是具有长度(深度)、宽度和高度的三维形状。 在 JavaFX 中,三维盒子由名为Box的类表示。该类属于包javafx.scene.shape。 通过实例化此类,您可以在 JavaFX 中创建 Box 节点。 这个类有 3 个 double 数据类型的属性,即 -
|
2 | 圆柱
圆柱体是一个封闭的实体,具有两个通过曲面连接的平行(大部分是圆形)底座。 它由两个参数来描述,即圆形底面的半径和圆柱体的高度。 在 JavaFX 中,圆柱体由名为Cylinder的类表示。该类属于包javafx.scene.shape。 通过实例化此类,您可以在 JavaFX 中创建圆柱体节点。这个类有 2 个 double 数据类型的属性,即 -
|
3 | 领域
球体被定义为距 3D 空间中给定点均具有相同距离 r 的点集。该距离 r 是球体的半径,给定点是球体的中心。 在 JavaFX 中,球体由名为Sphere的类表示。该类属于包javafx.scene.shape。 通过实例化此类,您可以在 JavaFX 中创建球体节点。 该类有一个名为radius的 double 数据类型属性。它代表球体的半径。 |
3D 对象的属性
对于所有 3 维对象,您可以设置各种属性,例如剔除面、绘图模式、材质。
以下部分讨论 3D 对象的属性。
剔除面
一般来说,剔除是指移除形状中方向不正确的部分(在视图区域中不可见)。
Cull Face 属性的类型为CullFace,它表示 3D 形状的 Cull Face。您可以使用setCullFace()方法设置形状的剔除面,如下所示 -
box.setCullFace(CullFace.NONE);
形状的笔划类型可以是 -
None - 不执行剔除(CullFace.NONE)。
Front - 所有正面的多边形都被剔除。(CullFace.FRONT)。
背面- 所有背面的多边形都被剔除。(笔划类型.BACK)。
默认情况下,3 维形状的剔除面为“背面”。
例子
以下程序是演示球体的各种剔除面的示例。将此代码保存在名为SphereCullFace.java的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.CullFace; import javafx.stage.Stage; import javafx.scene.shape.Sphere; public class SphereCullFace extends Application { @Override public void start(Stage stage) { //Drawing Sphere1 Sphere sphere1 = new Sphere(); //Setting the radius of the Sphere sphere1.setRadius(50.0); //Setting the position of the sphere sphere1.setTranslateX(100); sphere1.setTranslateY(150); //setting the cull face of the sphere to front sphere1.setCullFace(CullFace.FRONT); //Drawing Sphere2 Sphere sphere2 = new Sphere(); //Setting the radius of the Sphere sphere2.setRadius(50.0); //Setting the position of the sphere sphere2.setTranslateX(300); sphere2.setTranslateY(150); //Setting the cull face of the sphere to back sphere2.setCullFace(CullFace.BACK); //Drawing Sphere3 Sphere sphere3 = new Sphere(); //Setting the radius of the Sphere sphere3.setRadius(50.0); //Setting the position of the sphere sphere3.setTranslateX(500); sphere3.setTranslateY(150); //Setting the cull face of the sphere to none sphere2.setCullFace(CullFace.NONE); //Creating a Group object Group root = new Group(sphere1, sphere2, sphere3); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a Sphere"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac SphereCullFace.java java SphereCullFace
执行时,上述程序生成一个 JavaFX 窗口,显示三个球体,其剔除面值分别为FRONT、BACK和NONE,如下所示 -

绘图模式
该属性的类型为DrawMode,表示用于绘制当前 3D 形状的绘制模式。您可以使用 setDrawMode () 方法选择绘制模式来绘制 3D 形状,如下所示 -
box.setDrawMode(DrawMode.FILL);
在 JavaFX 中,您可以选择两种绘制模式来绘制 3D 形状,它们是 -
填充- 此模式绘制并填充 2D 形状 (DrawMode.FILL)。
Line - 此模式使用线绘制 3D 形状 (DrawMode.LINE)。
默认情况下,3D 形状的绘制模式为填充。
例子
以下程序是演示 3D 框的各种绘制模式的示例。将此代码保存在名为BoxDrawMode.java的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.PerspectiveCamera; import javafx.scene.Scene; import javafx.scene.shape.Box; import javafx.scene.shape.DrawMode; import javafx.stage.Stage; public class BoxDrawMode extends Application { @Override public void start(Stage stage) { //Drawing a Box Box box1 = new Box(); //Setting the properties of the Box box1.setWidth(100.0); box1.setHeight(100.0); box1.setDepth(100.0); //Setting the position of the box box1.setTranslateX(200); box1.setTranslateY(150); box1.setTranslateZ(0); //Setting the drawing mode of the box box1.setDrawMode(DrawMode.LINE); //Drawing a Box Box box2 = new Box(); //Setting the properties of the Box box2.setWidth(100.0); box2.setHeight(100.0); box2.setDepth(100.0); //Setting the position of the box box2.setTranslateX(450); //450 box2.setTranslateY(150);//150 box2.setTranslateZ(300); //Setting the drawing mode of the box box2.setDrawMode(DrawMode.FILL); //Creating a Group object Group root = new Group(box1, box2); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting camera PerspectiveCamera camera = new PerspectiveCamera(false); camera.setTranslateX(0); camera.setTranslateY(0); camera.setTranslateZ(0); scene.setCamera(camera); //Setting title to the Stage stage.setTitle("Drawing a Box"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac BoxDrawMode.java java BoxDrawMode
执行时,上述程序生成一个 JavaFX 窗口,显示两个框,分别具有绘制模式值 LINE 和 FILL,如下所示 -

材料
cull Face 属性属于Material类型,用于选择 3D 形状材质的表面。您可以使用setCullFace()方法设置 3D 形状的材质,如下所示 -
cylinder.setMaterial(material);
如上所述,对于此方法,您需要传递一个 Material 类型的对象。javafx.scene.paint包中的 PhongMaterial 类是该类的子类,它提供了 7 个表示 Phong 着色材质的属性。您可以使用这些属性的 setter 方法将所有这些类型的材质应用到 3D 形状的表面。
以下是 JavaFX 中可用的材料类型 -
凹凸贴图- 这表示存储为 RGB 图像的法线贴图。
diffemap--这代表一个漫反射贴图。
selfIlluminationMap - 这表示此 PhongMaterial 的自发光贴图。
SpecularMap - 这表示此 PhongMaterial 的镜面贴图。
iffuseColor - 这表示该 PhongMaterial 的漫反射颜色。
SpecularColor - 这表示此 PhongMaterial 的镜面颜色。
SpecularPower - 这代表了该 PhongMaterial 的镜面反射强度。
默认情况下,3 维形状的材质是具有浅灰色漫反射颜色的 PhongMaterial。
例子
以下是显示圆柱体上各种材料的示例。将此代码保存在名为CylinderMaterials.java的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.PerspectiveCamera; import javafx.scene.Scene; import javafx.scene.image.Image; import javafx.scene.paint.Color; import javafx.scene.paint.PhongMaterial; import javafx.scene.shape.Cylinder; import javafx.stage.Stage; public class CylinderMaterials extends Application { @Override public void start(Stage stage) { //Drawing Cylinder1 Cylinder cylinder1 = new Cylinder(); //Setting the properties of the Cylinder cylinder1.setHeight(130.0f); cylinder1.setRadius(30.0f); //Setting the position of the Cylinder cylinder1.setTranslateX(100); cylinder1.setTranslateY(75); //Preparing the phong material of type bump map PhongMaterial material1 = new PhongMaterial(); material1.setBumpMap(new Image ("http://www.tutorialspoint.com/images/tplogo.gif")); //Setting the bump map material to Cylinder1 cylinder1.setMaterial(material1); //Drawing Cylinder2 Cylinder cylinder2 = new Cylinder(); //Setting the properties of the Cylinder cylinder2.setHeight(130.0f); cylinder2.setRadius(30.0f); //Setting the position of the Cylinder cylinder2.setTranslateX(200); cylinder2.setTranslateY(75); //Preparing the phong material of type diffuse map PhongMaterial material2 = new PhongMaterial(); material2.setDiffuseMap(new Image ("http://www.tutorialspoint.com/images/tp-logo.gif")); //Setting the diffuse map material to Cylinder2 cylinder2.setMaterial(material2); //Drawing Cylinder3 Cylinder cylinder3 = new Cylinder(); //Setting the properties of the Cylinder cylinder3.setHeight(130.0f); cylinder3.setRadius(30.0f); //Setting the position of the Cylinder cylinder3.setTranslateX(300); cylinder3.setTranslateY(75); //Preparing the phong material of type Self Illumination Map PhongMaterial material3 = new PhongMaterial(); material3.setSelfIlluminationMap(new Image ("http://www.tutorialspoint.com/images/tp-logo.gif")); //Setting the Self Illumination Map material to Cylinder3 cylinder3.setMaterial(material3); //Drawing Cylinder4 Cylinder cylinder4 = new Cylinder(); //Setting the properties of the Cylinder cylinder4.setHeight(130.0f); cylinder4.setRadius(30.0f); //Setting the position of the Cylinder cylinder4.setTranslateX(400); cylinder4.setTranslateY(75); //Preparing the phong material of type Specular Map PhongMaterial material4 = new PhongMaterial(); material4.setSpecularMap(new Image ("http://www.tutorialspoint.com/images/tp-logo.gif")); //Setting the Specular Map material to Cylinder4 cylinder4.setMaterial(material4); //Drawing Cylinder5 Cylinder cylinder5 = new Cylinder(); //Setting the properties of the Cylinder cylinder5.setHeight(130.0f); cylinder5.setRadius(30.0f); //Setting the position of the Cylinder cylinder5.setTranslateX(100); cylinder5.setTranslateY(300); //Preparing the phong material of type diffuse color PhongMaterial material5 = new PhongMaterial(); material5.setDiffuseColor(Color.BLANCHEDALMOND); //Setting the diffuse color material to Cylinder5 cylinder5.setMaterial(material5); //Drawing Cylinder6 Cylinder cylinder6 = new Cylinder(); //Setting the properties of the Cylinder cylinder6.setHeight(130.0f); cylinder6.setRadius(30.0f); //Setting the position of the Cylinder cylinder6.setTranslateX(200); cylinder6.setTranslateY(300); //Preparing the phong material of type specular color PhongMaterial material6 = new PhongMaterial(); //setting the specular color map to the material material6.setSpecularColor(Color.BLANCHEDALMOND); //Setting the specular color material to Cylinder6 cylinder6.setMaterial(material6); //Drawing Cylinder7 Cylinder cylinder7 = new Cylinder(); //Setting the properties of the Cylinder cylinder7.setHeight(130.0f); cylinder7.setRadius(30.0f); //Setting the position of the Cylinder cylinder7.setTranslateX(300); cylinder7.setTranslateY(300); //Preparing the phong material of type Specular Power PhongMaterial material7 = new PhongMaterial(); material7.setSpecularPower(0.1); //Setting the Specular Power material to the Cylinder cylinder7.setMaterial(material7); //Creating a Group object Group root = new Group(cylinder1 ,cylinder2, cylinder3, cylinder4, cylinder5, cylinder6, cylinder7); //Creating a scene object Scene scene = new Scene(root, 600, 400); //Setting camera PerspectiveCamera camera = new PerspectiveCamera(false); camera.setTranslateX(0); camera.setTranslateY(0); camera.setTranslateZ(-10); scene.setCamera(camera); //Setting title to the Stage stage.setTitle("Drawing a cylinder"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
Javac CylinderMaterials.java java CylinderMaterials
执行时,上述程序生成一个 JavaFX 窗口,显示 7 个圆柱体,分别包含材质、凹凸贴图、漫反射贴图、自发光贴图、镜面反射贴图、漫反射颜色、镜面反射颜色、(BLANCHEDALMOND) 镜面反射强度,如下图所示-
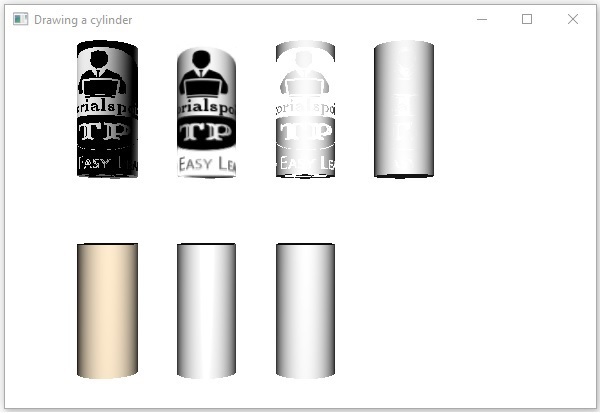