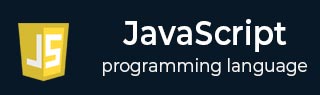
- JavaScript 基础教程
- JavaScript - 主页
- JavaScript - 概述
- JavaScript - 语法
- JavaScript - 启用
- JavaScript - 放置
- JavaScript - 变量
- JavaScript - 运算符
- JavaScript - 如果...否则
- Javascript - 切换大小写
- JavaScript - While 循环
- JavaScript - For 循环
- Javascript - 对于...in
- Javascript - 循环控制
- JavaScript - 函数
- Javascript - 事件
- JavaScript - Cookie
- JavaScript - 页面重定向
- JavaScript - 对话框
- Javascript - 无效关键字
- Javascript - 页面打印
- JavaScript 对象
- JavaScript - 对象
- JavaScript - 数字
- JavaScript - 布尔值
- JavaScript - 字符串
- JavaScript - 数组
- JavaScript - 日期
- JavaScript - 数学
- JavaScript - 正则表达式
- JavaScript - HTML DOM
- JavaScript 高级
- JavaScript - 错误处理
- Javascript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 调试
- Javascript - 图像映射
- JavaScript - 浏览器
- JavaScript 有用资源
- JavaScript - 问题与解答
- JavaScript - 快速指南
- JavaScript - 函数
- JavaScript - 资源
JavaScript - 运算符
什么是操作员?
让我们看一个简单的表达式4 + 5 等于 9。这里 4 和 5 称为操作数,“+”称为运算符。JavaScript 支持以下类型的运算符。
- 算术运算符
- 比较运算符
- 逻辑(或关系)运算符
- 赋值运算符
- 条件(或三元)运算符
让我们一一看看所有的运营商。
算术运算符
JavaScript 支持以下算术运算符 -
假设变量 A 为 10,变量 B 为 20,则 -
先生。 | 运算符及描述 |
---|---|
1 | +(加法) 添加两个操作数 例如: A + B 将给出 30 |
2 | -(减法) 从第一个操作数中减去第二个操作数 例如: A - B 将给出 -10 |
3 | *(乘法) 将两个操作数相乘 例如: A * B 将给出 200 |
4 | / (分配) 将分子除以分母 例如: B / A 将给出 2 |
5 | %(模数) 输出整数除法的余数 例如: B % A 将给出 0 |
6 | ++(增量) 将整数值加一 例如: A++ 会给出 11 |
7 | --(递减) 将整数值减一 例如: A-- 将给出 9 |
注意- 加法运算符 (+) 适用于数字和字符串。例如“a”+10 将得到“a10”。
例子
以下代码展示了如何在 JavaScript 中使用算术运算符。
<html> <body> <script type = "text/javascript"> <!-- var a = 33; var b = 10; var c = "Test"; var linebreak = "<br />"; document.write("a + b = "); result = a + b; document.write(result); document.write(linebreak); document.write("a - b = "); result = a - b; document.write(result); document.write(linebreak); document.write("a / b = "); result = a / b; document.write(result); document.write(linebreak); document.write("a % b = "); result = a % b; document.write(result); document.write(linebreak); document.write("a + b + c = "); result = a + b + c; document.write(result); document.write(linebreak); a = ++a; document.write("++a = "); result = ++a; document.write(result); document.write(linebreak); b = --b; document.write("--b = "); result = --b; document.write(result); document.write(linebreak); //--> </script> Set the variables to different values and then try... </body> </html>
输出
a + b = 43 a - b = 23 a / b = 3.3 a % b = 3 a + b + c = 43Test ++a = 35 --b = 8 Set the variables to different values and then try...
比较运算符
JavaScript 支持以下比较运算符 -
假设变量 A 为 10,变量 B 为 20,则 -
先生。 | 运算符及描述 |
---|---|
1 |
= =(等于) 检查两个操作数的值是否相等,如果相等,则条件成立。 例如: (A == B) 不正确。 |
2 |
!=(不等于) 检查两个操作数的值是否相等,如果值不相等,则条件成立。 例如: (A != B) 为真。 |
3 |
>(大于) 检查左操作数的值是否大于右操作数的值,如果是,则条件成立。 例如: (A > B) 不正确。 |
4 |
<(小于) 检查左操作数的值是否小于右操作数的值,如果是,则条件成立。 例如: (A < B) 为真。 |
5 |
>=(大于或等于) 检查左操作数的值是否大于或等于右操作数的值,如果是,则条件成立。 例如: (A >= B) 不正确。 |
6 |
<=(小于或等于) 检查左操作数的值是否小于或等于右操作数的值,如果是,则条件成立。 例如: (A <= B) 为真。 |
例子
以下代码展示了如何在 JavaScript 中使用比较运算符。
<html> <body> <script type = "text/javascript"> <!-- var a = 10; var b = 20; var linebreak = "<br />"; document.write("(a == b) => "); result = (a == b); document.write(result); document.write(linebreak); document.write("(a < b) => "); result = (a < b); document.write(result); document.write(linebreak); document.write("(a > b) => "); result = (a > b); document.write(result); document.write(linebreak); document.write("(a != b) => "); result = (a != b); document.write(result); document.write(linebreak); document.write("(a >= b) => "); result = (a >= b); document.write(result); document.write(linebreak); document.write("(a <= b) => "); result = (a <= b); document.write(result); document.write(linebreak); //--> </script> Set the variables to different values and different operators and then try... </body> </html>
输出
(a == b) => false (a < b) => true (a > b) => false (a != b) => true (a >= b) => false a <= b) => true Set the variables to different values and different operators and then try...
逻辑运算符
JavaScript 支持以下逻辑运算符 -
假设变量 A 为 10,变量 B 为 20,则 -
先生。 | 运算符及描述 |
---|---|
1 | &&(逻辑与) 如果两个操作数均非零,则条件为真。 例如: (A && B) 是正确的。 |
2 | || (逻辑或) 如果两个操作数中的任何一个非零,则条件为真。 例如: (A || B) 为真。 |
3 | !(逻辑非) 反转其操作数的逻辑状态。如果条件为真,则逻辑 NOT 运算符会将其设为假。 例如:!(A && B) 是错误的。 |
例子
尝试以下代码来了解如何在 JavaScript 中实现逻辑运算符。
<html> <body> <script type = "text/javascript"> <!-- var a = true; var b = false; var linebreak = "<br />"; document.write("(a && b) => "); result = (a && b); document.write(result); document.write(linebreak); document.write("(a || b) => "); result = (a || b); document.write(result); document.write(linebreak); document.write("!(a && b) => "); result = (!(a && b)); document.write(result); document.write(linebreak); //--> </script> <p>Set the variables to different values and different operators and then try...</p> </body> </html>
输出
(a && b) => false (a || b) => true !(a && b) => true Set the variables to different values and different operators and then try...
按位运算符
JavaScript 支持以下按位运算符 -
假设变量 A 为 2,变量 B 为 3,则 -
先生。 | 运算符及描述 |
---|---|
1 | &(按位与) 它对其整数参数的每一位执行布尔 AND 运算。 例如: (A & B) 是 2。 |
2 | | (按位或) 它对其整数参数的每一位执行布尔 OR 运算。 例如: (A | B) 为 3。 |
3 | ^(按位异或) 它对其整数参数的每一位执行布尔异或运算。异或意味着操作数一为真或操作数二为真,但不能两者都为真。 例如: (A ^ B) 为 1。 |
4 | ~(按位非) 它是一个一元运算符,通过反转操作数中的所有位来进行运算。 例如: (~B) 为 -4。 |
5 | <<(左移) 它将第一个操作数中的所有位向左移动第二个操作数中指定的位数。新的位用零填充。将值左移一位相当于乘以 2,左移两位相当于乘以 4,依此类推。 例如: (A << 1) 是 4。 |
6 | >>(右移) 二进制右移运算符。左操作数的值向右移动右操作数指定的位数。 例如: (A >> 1) 是 1。 |
7 | >>>(右移零) 该运算符与 >> 运算符类似,只是左侧移入的位始终为零。 例如: (A >>> 1) 是 1。 |
例子
尝试使用以下代码在 JavaScript 中实现按位运算符。
<html> <body> <script type = "text/javascript"> <!-- var a = 2; // Bit presentation 10 var b = 3; // Bit presentation 11 var linebreak = "<br />"; document.write("(a & b) => "); result = (a & b); document.write(result); document.write(linebreak); document.write("(a | b) => "); result = (a | b); document.write(result); document.write(linebreak); document.write("(a ^ b) => "); result = (a ^ b); document.write(result); document.write(linebreak); document.write("(~b) => "); result = (~b); document.write(result); document.write(linebreak); document.write("(a << b) => "); result = (a << b); document.write(result); document.write(linebreak); document.write("(a >> b) => "); result = (a >> b); document.write(result); document.write(linebreak); //--> </script> <p>Set the variables to different values and different operators and then try...</p> </body> </html>
(a & b) => 2 (a | b) => 3 (a ^ b) => 1 (~b) => -4 (a << b) => 16 (a >> b) => 0 Set the variables to different values and different operators and then try...
赋值运算符
JavaScript 支持以下赋值运算符 -
先生。 | 运算符及描述 |
---|---|
1 | =(简单赋值) 将右侧操作数中的值分配给左侧操作数 例如: C = A + B 将把 A + B 的值赋给 C |
2 | +=(添加和赋值) 它将右操作数添加到左操作数,并将结果分配给左操作数。 例如: C += A 相当于 C = C + A |
3 | −=(减法和赋值) 它从左操作数中减去右操作数,并将结果赋给左操作数。 例如: C -= A 相当于 C = C - A |
4 | *=(乘法和赋值) 它将右操作数与左操作数相乘,并将结果赋给左操作数。 例如: C *= A 相当于 C = C * A |
5 | /=(除法和赋值) 它将左操作数与右操作数相除,并将结果赋给左操作数。 例如: C /= A 相当于 C = C / A |
6 | %=(模块和分配) 它使用两个操作数取模并将结果分配给左侧操作数。 例如: C %= A 相当于 C = C % A |
注意- 相同的逻辑适用于按位运算符,因此它们将变得像 <<=、>>=、>>=、&=、|= 和 ^=。
例子
尝试使用以下代码在 JavaScript 中实现赋值运算符。
<html> <body> <script type = "text/javascript"> <!-- var a = 33; var b = 10; var linebreak = "<br />"; document.write("Value of a => (a = b) => "); result = (a = b); document.write(result); document.write(linebreak); document.write("Value of a => (a += b) => "); result = (a += b); document.write(result); document.write(linebreak); document.write("Value of a => (a -= b) => "); result = (a -= b); document.write(result); document.write(linebreak); document.write("Value of a => (a *= b) => "); result = (a *= b); document.write(result); document.write(linebreak); document.write("Value of a => (a /= b) => "); result = (a /= b); document.write(result); document.write(linebreak); document.write("Value of a => (a %= b) => "); result = (a %= b); document.write(result); document.write(linebreak); //--> </script> <p>Set the variables to different values and different operators and then try...</p> </body> </html>
输出
Value of a => (a = b) => 10 Value of a => (a += b) => 20 Value of a => (a -= b) => 10 Value of a => (a *= b) => 100 Value of a => (a /= b) => 10 Value of a => (a %= b) => 0 Set the variables to different values and different operators and then try...
杂项操作员
我们将在这里讨论 JavaScript 中非常有用的两个运算符:条件运算符(?:) 和typeof 运算符。
条件运算符 (?:)
条件运算符首先计算表达式的真值或假值,然后根据计算结果执行两个给定语句之一。
先生。 | 操作符及说明 |
---|---|
1 | ?:(有条件) 如果条件为真?然后值 X :否则值 Y |
例子
尝试以下代码来了解条件运算符在 JavaScript 中的工作原理。
<html> <body> <script type = "text/javascript"> <!-- var a = 10; var b = 20; var linebreak = "<br />"; document.write ("((a > b) ? 100 : 200) => "); result = (a > b) ? 100 : 200; document.write(result); document.write(linebreak); document.write ("((a < b) ? 100 : 200) => "); result = (a < b) ? 100 : 200; document.write(result); document.write(linebreak); //--> </script> <p>Set the variables to different values and different operators and then try...</p> </body> </html>
输出
((a > b) ? 100 : 200) => 200 ((a < b) ? 100 : 200) => 100 Set the variables to different values and different operators and then try...
运算符类型
typeof运算符是一个一元运算符,位于其单个操作数之前,该操作数可以是任何类型。它的值是一个字符串,指示操作数的数据类型。
如果typeof运算符的操作数是数字、字符串或布尔值,则其计算结果为“数字”、“字符串”或“布尔值”,并根据计算结果返回 true 或 false。
以下是typeof运算符的返回值列表。
类型 | typeof 返回的字符串 |
---|---|
数字 | “数字” |
细绳 | “细绳” |
布尔值 | “布尔值” |
目的 | “目的” |
功能 | “功能” |
不明确的 | “不明确的” |
无效的 | “目的” |
例子
以下代码展示了如何实现typeof运算符。
<html> <body> <script type = "text/javascript"> <!-- var a = 10; var b = "String"; var linebreak = "<br />"; result = (typeof b == "string" ? "B is String" : "B is Numeric"); document.write("Result => "); document.write(result); document.write(linebreak); result = (typeof a == "string" ? "A is String" : "A is Numeric"); document.write("Result => "); document.write(result); document.write(linebreak); //--> </script> <p>Set the variables to different values and different operators and then try...</p> </body> </html>
输出
Result => B is String Result => A is Numeric Set the variables to different values and different operators and then try...