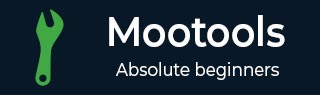
- MooTools教程
- MooTools - 主页
- MooTools - 简介
- MooTools - 安装
- MooTools - 程序结构
- MooTools - 选择器
- MooTools - 使用数组
- MooTools - 函数
- MooTools - 事件处理
- MooTools - DOM 操作
- MooTools - 样式属性
- MooTools - 输入过滤
- MooTools - 拖放
- MooTools - 正则表达式
- MooTools - 期刊
- MooTools - 滑块
- MooTools - 可排序
- MooTools - 手风琴
- MooTools - 工具提示
- MooTools - 选项卡式内容
- MooTools - 类
- MooTools - Fx.Element
- MooTools - Fx.Slide
- MooTools - Fx.Tween
- MooTools - Fx.Morph
- MooTools - Fx.Options
- MooTools - Fx.Events
- MooTools 有用资源
- MooTools - 快速指南
- MooTools - 有用的资源
- MooTools - 讨论
MooTools - 选项卡式内容
选项卡式内容是指选项卡式区域中存在的内容,并且该内容与列表项相关。每当我们对列表项应用任何操作(例如悬停或单击)时,立即反应都会对选项卡式内容产生效果。
让我们更多地讨论选项卡。
创建简单的选项卡
创建简单的菜单选项卡可帮助您将鼠标悬停在列表项上时探索其他信息。首先,创建一个包含项目的无序列表,然后创建 div,每个 div 对应一个列表项。让我们看一下下面的 HTML 代码。
脚本
<!-- here is our menu --> <ul id = "tabs"> <li id = "one">One</li> <li id = "two">Two</li> <li id = "three">Three</li> <li id = "four">Four</li> </ul> <!-- and here are our content divs --> <div id = "contentone" class = "hidden">content for one</div> <div id = "contenttwo" class = "hidden">content for two</div> <div id = "contentthree" class = "hidden">content for three</div> <div id = "contentfour" class = "hidden">content for four</div>
让我们使用 CSS 为上述 HTML 代码提供一些基本支持,以帮助隐藏数据。看看下面的代码。
.hidden { display: none; }
现在让我们编写一段 MooTools 代码来展示选项卡功能。看看下面的代码。
示例片段
//here are our functions to change the styles var showFunction = function() { this.setStyle('display', 'block'); } var hideFunction = function() { this.setStyle('display', 'none'); } window.addEvent('domready', function() { //here we turn our content elements into vars var elOne = $('contentone'); var elTwo = $('contenttwo'); var elThree = $('contentthree'); var elFour = $('contentfour'); //add the events to the tabs $('one').addEvents({ //set up the events types //and bind the function with the variable to pass 'mouseenter': showFunction.bind(elOne), 'mouseleave': hideFunction.bind(elOne) }); $('two').addEvents({ 'mouseenter': showFunction.bind(elTwo), 'mouseleave': hideFunction.bind(elTwo) }); $('three').addEvents({ 'mouseenter': showFunction.bind(elThree), 'mouseleave': hideFunction.bind(elThree) }); $('four').addEvents({ 'mouseenter': showFunction.bind(elFour), 'mouseleave': hideFunction.bind(elFour) }); });
结合上述代码,您将获得正确的功能。
例子
<!DOCTYPE html> <html> <head> <style> .hidden { display: none; } </style> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript"> //here are our functions to change the styles var showFunction = function() { this.setStyle('display', 'block'); } var hideFunction = function() { this.setStyle('display', 'none'); } window.addEvent('domready', function() { //here we turn our content elements into vars var elOne = $('contentone'); var elTwo = $('contenttwo'); var elThree = $('contentthree'); var elFour = $('contentfour'); //add the events to the tabs $('one').addEvents({ //set up the events types //and bind the function with the variable to pass 'mouseenter': showFunction.bind(elOne), 'mouseleave': hideFunction.bind(elOne) }); $('two').addEvents({ 'mouseenter': showFunction.bind(elTwo), 'mouseleave': hideFunction.bind(elTwo) }); $('three').addEvents({ 'mouseenter': showFunction.bind(elThree), 'mouseleave': hideFunction.bind(elThree) }); $('four').addEvents({ 'mouseenter': showFunction.bind(elFour), 'mouseleave': hideFunction.bind(elFour) }); }); </script> </head> <body> <!-- here is our menu --> <ul id = "tabs"> <li id = "one">One</li> <li id = "two">Two</li> <li id = "three">Three</li> <li id = "four">Four</li> </ul> <!-- and here are our content divs --> <div id = "contentone" class = "hidden">content for one</div> <div id = "contenttwo" class = "hidden">content for two</div> <div id = "contentthree" class = "hidden">content for three</div> <div id = "contentfour" class = "hidden">content for four</div> </body> </html>
输出
将鼠标指针放在列表项上,您将获得相应项目的附加信息。
Marph 内容选项卡
通过扩展代码,我们可以在显示隐藏内容时添加一些变形功能。我们可以通过使用 Fx.Morph 效果而不是样式来实现这一点。
看看下面的代码。
例子
<!DOCTYPE html> <html> <head> <style> .hiddenM { display: none; } </style> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript"> var showFunction = function() { //resets all the styles before it morphs the current one $$('.hiddenM').setStyles({ 'display': 'none', 'opacity': 0, 'background-color': '#fff', 'font-size': '16px' }); //here we start the morph and set the styles to morph to this.start({ 'display': 'block', 'opacity': 1, 'background-color': '#d3715c', 'font-size': '31px' }); } window.addEvent('domready', function() { var elOneM = $('contentoneM'); var elTwoM = $('contenttwoM'); var elThreeM = $('contentthreeM'); var elFourM = $('contentfourM'); //creat morph object elOneM = new Fx.Morph(elOneM, { link: 'cancel' }); elTwoM = new Fx.Morph(elTwoM, { link: 'cancel' }); elThreeM = new Fx.Morph(elThreeM, { link: 'cancel' }); elFourM = new Fx.Morph(elFourM, { link: 'cancel' }); $('oneM').addEvent('click', showFunction.bind(elOneM)); $('twoM').addEvent('click', showFunction.bind(elTwoM)); $('threeM').addEvent('click', showFunction.bind(elThreeM)); $('fourM').addEvent('click', showFunction.bind(elFourM)); }); </script> </head> <body> <!-- here is our menu --> <ul id = "tabs"> <li id = "oneM">One</li> <li id = "twoM">Two</li> <li id = "threeM">Three</li> <li id = "fourM">Four</li> </ul> <!-- and here are our content divs --> <div id = "contentoneM" class = "hiddenM">content for one</div> <div id = "contenttwoM" class = "hiddenM">content for two</div> <div id = "contentthreeM" class = "hiddenM">content for three</div> <div id = "contentfourM" class = "hiddenM">content for four</div> </body> </html>
输出
单击列表中的任意一项,您将在选项卡上获得更多信息。