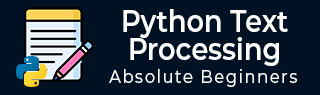
- Python-文本处理
- Python-文本处理简介
- Python - 文本处理环境
- Python - 字符串不变性
- Python - 排序行
- Python - 重新格式化段落
- Python - 计算段落中的标记
- Python - 二进制 ASCII 转换
- Python - 字符串作为文件
- Python-向后读取文件
- Python - 过滤重复单词
- Python - 从文本中提取电子邮件
- Python - 从文本中提取 URL
- Python - 漂亮的打印
- Python - 文本处理状态机
- Python - 大写和翻译
- Python - 标记化
- Python - 删除停用词
- Python - 同义词和反义词
- Python - 文本翻译
- Python-单词替换
- Python-拼写检查
- Python - WordNet 接口
- Python - 语料库访问
- Python - 标记单词
- Python - 块和缝隙
- Python - 块分类
- Python-文本分类
- Python-二元组
- Python - 处理 PDF
- Python-处理Word文档
- Python - 读取 RSS 提要
- Python-情感分析
- Python - 搜索和匹配
- Python - 文本修改
- Python-文本换行
- Python-频率分布
- Python-文本摘要
- Python - 词干算法
- Python - 约束搜索
Python-情感分析
语义分析是分析受众的总体意见。这可能是对一条新闻、电影或任何有关正在讨论的问题的推文的反应。一般来说,此类反应是从社交媒体中获取的,并合并到一个文件中,以便通过 NLP 进行分析。我们先看一个简单的例子,定义积极和消极的词。然后采取一种方法来分析这些单词作为使用这些单词的句子的一部分。我们使用 nltk 的情感分析器模块。我们首先使用一个单词进行分析,然后使用配对单词(也称为二元组)进行分析。最后,我们按照mark_negation函数中的定义标记具有负面情绪的单词。
import nltk import nltk.sentiment.sentiment_analyzer # Analysing for single words def OneWord(): positive_words = ['good', 'progress', 'luck'] text = 'Hard Work brings progress and good luck.'.split() analysis = nltk.sentiment.util.extract_unigram_feats(text, positive_words) print(' ** Sentiment with one word **\n') print(analysis) # Analysing for a pair of words def WithBigrams(): word_sets = [('Regular', 'fit'), ('fit', 'fine')] text = 'Regular excercise makes you fit and fine'.split() analysis = nltk.sentiment.util.extract_bigram_feats(text, word_sets) print('\n*** Sentiment with bigrams ***\n') print analysis # Analysing the negation words. def NegativeWord(): text = 'Lack of good health can not bring success to students'.split() analysis = nltk.sentiment.util.mark_negation(text) print('\n**Sentiment with Negative words**\n') print(analysis) OneWord() WithBigrams() NegativeWord()
当我们运行上面的程序时,我们得到以下输出 -
** Sentiment with one word ** {'contains(luck)': False, 'contains(good)': True, 'contains(progress)': True} *** Sentiment with bigrams *** {'contains(fit - fine)': False, 'contains(Regular - fit)': False} **Sentiment with Negative words** ['Lack', 'of', 'good', 'health', 'can', 'not', 'bring_NEG', 'success_NEG', 'to_NEG', 'students_NEG']