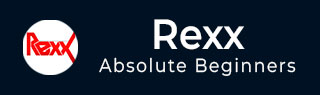
- 雷克斯教程
- 雷克斯 - 主页
- Rexx - 概述
- Rexx - 环境
- Rexx - 安装
- Rexx - 插件安装
- Rexx - 基本语法
- Rexx - 数据类型
- Rexx - 变量
- Rexx - 操作员
- Rexx - 数组
- Rexx - 循环
- Rexx - 决策
- Rexx - 数字
- Rexx - 弦乐
- Rexx - 功能
- Rexx - 堆栈
- Rexx - 文件 I/O
- Rexx - 文件函数
- Rexx - 子程序
- Rexx - 内置函数
- Rexx - 系统命令
- 雷克斯-XML
- 雷克斯 - 里贾纳
- Rexx - 解析
- Rexx - 信号
- Rexx - 调试
- Rexx - 错误处理
- Rexx - 面向对象
- Rexx - 便携性
- Rexx - 扩展功能
- Rexx - 说明
- Rexx - 实施
- 雷克斯 - Netrexx
- 雷克斯 - Brexx
- Rexx - 数据库
- 手持式和嵌入式
- Rexx - 性能
- Rexx - 最佳编程实践
- Rexx - 图形用户界面
- 雷克斯 - 雷金纳德
- Rexx - 网络编程
- 雷克斯有用资源
- Rexx - 快速指南
- Rexx - 有用的资源
- Rexx - 讨论
Rexx - 面向对象
当您按照环境章节安装 ooRexx 时,您还可以使用类和对象。请注意,以下所有代码都需要在 ooRexx 解释器中运行。普通的 Rexx 解释器将无法运行此面向对象的代码。
类和方法声明
类是使用以下语法声明定义的。
句法
::class classname
其中classname是为类指定的名称。
类中的方法是使用以下语法声明定义的。
句法
::method methodname
其中methodname是为方法指定的名称。
类中的属性是使用以下语法声明定义的。
句法
::attribute propertyname
其中propertyname是属性的名称。
例子
以下是 Rexx 中的类的示例。
::class student ::attribute StudentID ::attribute StudentName
上述程序需要注意以下几点。
- 班级名称是学生。
- 该类有 2 个属性:StudentID 和 StudentName。
Getter 和 Setter 方法
Getter 和Setter 方法用于自动设置和获取属性的值。在 Rexx 中,当您使用 attribute 关键字声明属性时,getter 和 setter 方法就已经就位。
例子
::class student ::attribute StudentID ::attribute StudentName
在上面的示例中,StudentId 和 StudentName 都有 Getter 和 Setter 方法。
以下程序显示了如何使用它们的示例。
/* Main program */ value = .student~new value~StudentID = 1 value~StudentName = 'Joe' say value~StudentID say value~StudentName exit 0 ::class student ::attribute StudentID ::attribute StudentName
上述程序的输出如下所示。
1 Joe
实例方法
可以通过~new 运算符从类创建对象。可以通过以下方式调用该类的方法。
Object~methodname
其中methodname是需要从类中调用的方法。
例子
以下示例显示如何从类创建对象并调用相应的方法。
/* Main program */ value = .student~new value~StudentID = 1 value~StudentName = 'Joe' value~Marks1 = 10 value~Marks2 = 20 value~Marks3 = 30 total = value~Total(value~Marks1,value~Marks2,value~Marks3) say total exit 0 ::class student ::attribute StudentID ::attribute StudentName ::attribute Marks1 ::attribute Marks2 ::attribute Marks3 ::method 'Total' use arg a,b,c return (ABS(a) + ABS(b) + ABS(c))
上述程序的输出如下所示。
60
创建多个对象
还可以创建一个类的多个对象。以下示例将展示如何实现这一点。
在这里,我们创建 3 个对象(st、st1 和 st2)并相应地调用它们的实例成员和实例方法。
让我们看一个如何创建多个对象的示例。
例子
/* Main program */ st = .student~new st~StudentID = 1 st~StudentName = 'Joe' st~Marks1 = 10 st~Marks2 = 20 st~Marks3 = 30 total = st~Total(st~Marks1,st~Marks2,st~Marks3) say total st1 = .student~new st1~StudentID = 2 st1~StudentName = 'John' st1~Marks1 = 10 st1~Marks2 = 20 st1~Marks3 = 40 total = st1~Total(st1~Marks1,st1~Marks2,st1~Marks3) say total st2 = .student~new st2~StudentID = 3 st2~StudentName = 'Mark' st2~Marks1 = 10 st2~Marks2 = 20 st2~Marks3 = 30 total = st2~Total(st2~Marks1,st2~Marks2,st2~Marks3) say total exit 0 ::class student ::attribute StudentID ::attribute StudentName ::attribute Marks1 ::attribute Marks2 ::attribute Marks3 ::method 'Total' use arg a,b,c return (ABS(a) + ABS(b) + ABS(c))
上述程序的输出如下所示。
60 70 60
遗产
继承可以定义为一个类获取另一个类的属性(方法和字段)的过程。通过使用继承,可以按层次结构顺序管理信息。
继承其他类的属性的类称为子类(派生类、子类),继承其他类的属性的类称为超类(基类、父类)。
让我们看一下 Rexx 中的继承示例。在下面的示例中,我们将创建一个名为Person的类。从那里我们使用 subclass 关键字创建Student 类作为Person 的子类。
例子
/* Main program */ st = .student~new st~StudentID = 1 st~StudentName = 'Joe' st~Marks1 = 10 st~Marks2 = 20 st~Marks3 = 30 say st~Total(st~Marks1,st~Marks2,st~Marks3) exit 0 ::class Person ::class student subclass Person ::attribute StudentID ::attribute StudentName ::attribute Marks1 ::attribute Marks2 ::attribute Marks3 ::method 'Total' use arg a,b,c return (ABS(a) + ABS(b) + ABS(c))
上述程序的输出如下所示。
60