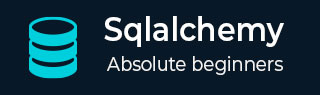
- SQLAlchemy 教程
- SQLAlchemy - 主页
- SQLAlchemy - 简介
- SQLAlchemy 核心
- 表达语言
- 连接到数据库
- 创建表
- SQL 表达式
- 执行表达式
- 选择行
- 使用文本 SQL
- 使用别名
- 使用 UPDATE 表达式
- 使用 DELETE 表达式
- 使用多个表
- 使用多个表更新
- 按参数顺序更新
- 多表删除
- 使用连接
- 使用连词
- 使用函数
- 使用集合运算
- SQLAlchemy ORM
- 声明映射
- 创建会话
- 添加对象
- 使用查询
- 更新对象
- 应用过滤器
- 过滤器运算符
- 返回列表和标量
- 文本SQL
- 建立关系
- 使用相关对象
- 使用连接
- 常见关系运算符
- 急切加载
- 删除相关对象
- 多对多关系
- 方言
- SQLAlchemy 有用资源
- SQLAlchemy - 快速指南
- SQLAlchemy - 有用的资源
- SQLAlchemy - 讨论
SQLAlchemy 核心 - 使用函数
本章讨论 SQLAlchemy 中使用的一些重要函数。
标准 SQL 推荐了许多函数,大多数方言都实现了这些函数。它们根据传递给它的参数返回一个值。有些 SQL 函数将列作为参数,而有些则是通用的。SQLAlchemy API 中的func 关键字用于生成这些函数。
在 SQL 中,now() 是一个通用函数。以下语句使用 func 呈现 now() 函数 -
from sqlalchemy.sql import func result = conn.execute(select([func.now()])) print (result.fetchone())
上述代码的示例结果可能如下所示 -
(datetime.datetime(2018, 6, 16, 6, 4, 40),)
另一方面,返回从表中选择的行数的 count() 函数是通过以下 func 用法呈现的:
from sqlalchemy.sql import func result = conn.execute(select([func.count(students.c.id)])) print (result.fetchone())
从上面的代码中,将获取学生表中的行数。
使用 Employee 表和以下数据演示了一些内置 SQL 函数 -
ID | 姓名 | 分数 |
---|---|---|
1 | 卡迈勒 | 56 |
2 | 费尔南德斯 | 85 |
3 | 苏尼尔 | 62 |
4 | 巴斯卡 | 76 |
max() 函数是通过使用 SQLAlchemy 中的 func 来实现的,这将得到 85,即获得的总最大分数 -
from sqlalchemy.sql import func result = conn.execute(select([func.max(employee.c.marks)])) print (result.fetchone())
同样,将返回 56(最小分数)的 min() 函数将通过以下代码呈现 -
from sqlalchemy.sql import func result = conn.execute(select([func.min(employee.c.marks)])) print (result.fetchone())
因此,AVG() 函数也可以通过使用以下代码来实现 -
from sqlalchemy.sql import func result = conn.execute(select([func.avg(employee.c.marks)])) print (result.fetchone()) Functions are normally used in the columns clause of a select statement. They can also be given label as well as a type. A label to function allows the result to be targeted in a result row based on a string name, and a type is required when you need result-set processing to occur.from sqlalchemy.sql import func result = conn.execute(select([func.max(students.c.lastname).label('Name')])) print (result.fetchone())