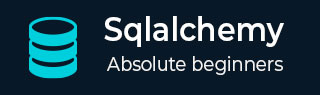
- SQLAlchemy 教程
- SQLAlchemy - 主页
- SQLAlchemy - 简介
- SQLAlchemy 核心
- 表达语言
- 连接到数据库
- 创建表
- SQL 表达式
- 执行表达式
- 选择行
- 使用文本 SQL
- 使用别名
- 使用 UPDATE 表达式
- 使用 DELETE 表达式
- 使用多个表
- 使用多个表更新
- 按参数顺序更新
- 多表删除
- 使用连接
- 使用连词
- 使用函数
- 使用集合运算
- SQLAlchemy ORM
- 声明映射
- 创建会话
- 添加对象
- 使用查询
- 更新对象
- 应用过滤器
- 过滤器运算符
- 返回列表和标量
- 文本SQL
- 建立关系
- 使用相关对象
- 使用连接
- 常见关系运算符
- 急切加载
- 删除相关对象
- 多对多关系
- 方言
- SQLAlchemy 有用资源
- SQLAlchemy - 快速指南
- SQLAlchemy - 有用的资源
- SQLAlchemy - 讨论
SQLAlchemy 核心 - 使用文本 SQL
SQLAlchemy 允许您仅使用字符串,适用于 SQL 已知且不强烈需要语句支持动态功能的情况。text() 构造用于编写基本不变地传递到数据库的文本语句。
它构造一个新的TextClause,直接表示文本 SQL 字符串,如下面的代码所示 -
from sqlalchemy import text t = text("SELECT * FROM students") result = connection.execute(t)
text()相对于普通字符串的优点是 -
- 对绑定参数的后端中立支持
- 每条语句的执行选项
- 结果列键入Behave
text() 函数需要指定冒号格式的绑定参数。无论数据库后端如何,它们都是一致的。为了发送参数值,我们将它们作为附加参数传递到execute()方法中。
以下示例在文本 SQL 中使用绑定参数 -
from sqlalchemy.sql import text s = text("select students.name, students.lastname from students where students.name between :x and :y") conn.execute(s, x = 'A', y = 'L').fetchall()
text() 函数构造 SQL 表达式如下 -
select students.name, students.lastname from students where students.name between ? and ?
x = 'A' 和 y = 'L' 的值作为参数传递。结果是名称介于“A”和“L”之间的行列表 -
[('Komal', 'Bhandari'), ('Abdul', 'Sattar')]
text() 构造支持使用 TextClause.bindparams() 方法预先建立的绑定值。参数也可以明确输入如下 -
stmt = text("SELECT * FROM students WHERE students.name BETWEEN :x AND :y") stmt = stmt.bindparams( bindparam("x", type_= String), bindparam("y", type_= String) ) result = conn.execute(stmt, {"x": "A", "y": "L"}) The text() function also be produces fragments of SQL within a select() object that accepts text() objects as an arguments. The “geometry” of the statement is provided by select() construct , and the textual content by text() construct. We can build a statement without the need to refer to any pre-established Table metadata. from sqlalchemy.sql import select s = select([text("students.name, students.lastname from students")]).where(text("students.name between :x and :y")) conn.execute(s, x = 'A', y = 'L').fetchall()
您还可以使用and_()函数组合在 text() 函数的帮助下创建的 WHERE 子句中的多个条件。
from sqlalchemy import and_ from sqlalchemy.sql import select s = select([text("* from students")]) \ .where( and_( text("students.name between :x and :y"), text("students.id>2") ) ) conn.execute(s, x = 'A', y = 'L').fetchall()
上面的代码获取名称在“A”和“L”之间且 id 大于 2 的行。代码的输出如下 -
[(3, 'Komal', 'Bhandari'), (4, 'Abdul', 'Sattar')]