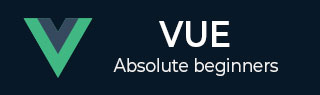
- VueJS Tutorial
- VueJS - Home
- VueJS - Overview
- VueJS - Environment Setup
- VueJS - Introduction
- VueJS - Instances
- VueJS - Template
- VueJS - Components
- VueJS - Computed Properties
- VueJS - Watch Property
- VueJS - Binding
- VueJS - Events
- VueJS - Rendering
- VueJS - Transition & Animation
- VueJS - Directives
- VueJS - Routing
- VueJS - Mixins
- VueJS - Render Function
- VueJS - Reactive Interface
- VueJS - Examples
- VueJS Useful Resources
- VueJS - Quick Guide
- VueJS - Useful Resources
- VueJS - Discussion
VueJS - 组件
Vue 组件是 VueJS 的重要功能之一,它可以创建自定义元素,可以在 HTML 中重用。
让我们使用一个示例并创建一个组件,这将更好地理解组件如何与 VueJS 一起工作。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "component_test"> <testcomponent></testcomponent> </div> <div id = "component_test1"> <testcomponent></testcomponent> </div> <script type = "text/javascript" src = "js/vue_component.js"></script> </body> </html>
vue_component.js
Vue.component('testcomponent',{ template : '<div><h1>This is coming from component</h1></div>' }); var vm = new Vue({ el: '#component_test' }); var vm1 = new Vue({ el: '#component_test1' });
在 .html 文件中,我们创建了两个 ID 为component_test和component_test1的 div 。在上面显示的.js文件中,使用 div id 创建了两个 Vue 实例。我们创建了一个与两个视图实例一起使用的通用组件。
要创建组件,语法如下。
Vue.component('nameofthecomponent',{ // options});
创建组件后,组件的名称将成为自定义元素,并且可以在创建的 Vue 实例元素中使用相同的名称,即在带有 ids component_test和component_test1的 div 内。
在.js文件中,我们使用测试组件作为组件的名称,并使用相同的名称作为 div 内的自定义元素。
例子
<div id = "component_test"> <testcomponent></testcomponent> </div> <div id = "component_test1"> <testcomponent></testcomponent> </div>
在.js文件中创建的组件中,我们添加了一个模板,并为其分配了 HTML 代码。这是注册全局组件的一种方法,可以将其作为任何 vue 实例的一部分,如以下脚本所示。
Vue.component('testcomponent',{ template : '<div><h1>This is coming from component</h1></div>' });
执行时,浏览器中也会反映出来。

这些组件被赋予自定义元素标签,即<testcomponent></testcomponent>。但是,当我们在浏览器中检查相同内容时,我们不会注意到模板中存在纯 HTML 格式的自定义标记,如以下屏幕截图所示。

我们还直接将组件作为 vue 实例的一部分,如以下脚本所示。
var vm = new Vue({ el: '#component_test', components:{ 'testcomponent': { template : '<div><h1>This is coming from component</h1></div>' } } });
这称为本地注册,组件将仅是创建的 vue 实例的一部分。
到目前为止,我们已经看到了具有基本选项的基本组件。现在,让我们向其中添加更多选项,例如数据和方法。正如 Vue 实例有数据和方法一样,组件也有相同的数据和方法。因此,我们将扩展我们已经在数据和方法中看到的代码。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "component_test"> <testcomponent></testcomponent> </div> <div id = "component_test1"> <testcomponent></testcomponent> </div> <script type = "text/javascript" src = "js/vue_component.js"></script> </body> </html>
vue_component.js
Vue.component('testcomponent',{ template : '<div v-on:mouseover = "changename()" v-on:mouseout = "originalname();"><h1>Custom Component created by <span id = "name">{{name}}</span></h1></div>', data: function() { return { name : "Ria" } }, methods:{ changename : function() { this.name = "Ben"; }, originalname: function() { this.name = "Ria"; } } }); var vm = new Vue({ el: '#component_test' }); var vm1 = new Vue({ el: '#component_test1' });
在上面的.js文件中,我们添加了作为函数的数据,该函数返回一个对象。该对象有一个名称属性,该属性被赋予值“Ria”。这在以下模板中使用。
template : '<div v-on:mouseover = "changename()" v-on:mouseout = "originalname();"><h1>Custom Component created by <span id = "name">{{name}}</span></h1></div>',
尽管数据作为组件中的函数,我们可以像使用直接 Vue 实例一样使用它的属性。此外,还添加了两个方法:changename 和originalname。在changename中,我们正在更改name属性,在originalname中,我们将其重置回原始名称。
我们还在 div 上添加了两个事件:mouseover 和 mouseout。事件的详细信息将在事件章节中讨论。所以现在,mouseover 调用changename方法,mouseout 调用originalname方法。
其显示内容如以下浏览器所示。

在上面的浏览器中可以看到,它显示的是在 data 属性中分配的名称,这是相同的名称。我们还在 div 上分配了一个 mouseover 事件和一个 mouseout 事件。让我们看看当鼠标悬停和鼠标移出时会发生什么。

鼠标悬停时,我们看到第一个组件的名称更改为 Ben,但是第二个组件保持原样。这是因为数据组件是一个函数并且它返回一个对象。因此,当在一处更改时,在其他情况下不会覆盖该更改。
动态组件
动态组件是使用关键字<component></component>创建的,并使用属性进行绑定,如以下示例所示。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"> <component v-bind:is = "view"></component> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { view: 'component1' }, components: { 'component1': { template: '<div><span style = "font-size:25;color:red;">Dynamic Component</span></div>' } } }); </script> </body> </html>
输出

动态组件是使用以下语法创建的。
<component v-bind:is = "view"></component>
它有 v-bind:is = ”view”,并为其分配了一个值 view。View 在 Vue 实例中定义如下。
var vm = new Vue({ el: '#databinding', data: { view: 'component1' }, components: { 'component1': { template: '<div><span style = "font-size:25;color:red;">Dynamic Component</span></div>' } } });
执行时,模板动态组件将显示在浏览器中。