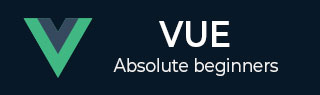
- VueJS Tutorial
- VueJS - Home
- VueJS - Overview
- VueJS - Environment Setup
- VueJS - Introduction
- VueJS - Instances
- VueJS - Template
- VueJS - Components
- VueJS - Computed Properties
- VueJS - Watch Property
- VueJS - Binding
- VueJS - Events
- VueJS - Rendering
- VueJS - Transition & Animation
- VueJS - Directives
- VueJS - Routing
- VueJS - Mixins
- VueJS - Render Function
- VueJS - Reactive Interface
- VueJS - Examples
- VueJS Useful Resources
- VueJS - Quick Guide
- VueJS - Useful Resources
- VueJS - Discussion
VueJS - 过渡和动画
在本章中,我们将讨论 VueJS 中可用的过渡和动画功能。
过渡
VueJS 提供了多种方法来在 DOM 中添加/更新 HTML 元素时应用转换。VueJS 有一个内置的转换组件,需要包裹在需要转换的元素周围。
句法
<transition name = "nameoftransition"> <div></div> </transition>
让我们考虑一个例子来理解转换的工作原理。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <style> .fade-enter-active, .fade-leave-active { transition: opacity 2s } .fade-enter, .fade-leave-to /* .fade-leave-active below version 2.1.8 */ { opacity: 0 } </style> <div id = "databinding"> <button v-on:click = "show = !show">Click Me</button> <transition name = "fade"> <p v-show = "show" v-bind:style = "styleobj">Animation Example</p> </transition> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { show:true, styleobj :{ fontSize:'30px', color:'red' } }, methods : { } }); </script> </body> </html>
创建了一个名为 clickme 的按钮,使用它我们可以将变量 show 的值更改为 true 到 false,反之亦然。有一个p 标签,仅当变量为 true 时才显示文本元素。我们用过渡元素包装了 p 标签,如下面的代码所示。
<transition name = "fade"> <p v-show = "show" v-bind:style = "styleobj">Animation Example</p> </transition>
过渡的名称是fade。VueJS 提供了一些用于转换的标准类,这些类以转换的名称为前缀。
以下是一些过渡的标准类 -
v-enter - 在更新/添加元素之前最初调用此类。它的起始状态。
v-enter-active - 此类用于定义进入过渡阶段的延迟、持续时间和缓动曲线。这是整个活动的状态,并且该类在整个进入阶段都可用。
v-leave - 当触发离开转换时添加,删除。
v-leave-active - 在离开阶段应用。转换完成后它将被删除。此类用于在离开阶段应用延迟、持续时间和缓动曲线。
上述每个类都将以转换名称为前缀。我们将过渡的名称指定为 fade,因此类的名称变为.fade_enter、.fade_enter_active、.fade_leave、.fade_leave_active。
它们在以下代码中定义。
<style> .fade-enter-active, .fade-leave-active { transition: opacity 2s } .fade-enter, .fade-leave-to /* .fade-leave-active below version 2.1.8 */ { opacity: 0 } </style>
.fade_enter_active 和 .fade_leave_active 一起定义,并在开始和离开阶段应用过渡。不透明度属性在 2 秒内更改为 0。
持续时间在 .fade_enter_active 和 .fade_leave_active 中定义。最后阶段在.fade_enter、.fade_leave_to中定义。
浏览器中的显示如下。

单击按钮后,文本将在两秒钟内消失。

两秒钟后,文字将完全消失。
让我们考虑另一个例子,其中有一个图像,当单击按钮时它会在 x 轴上移动。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <style> .shiftx-enter-active, .shiftx-leave-active { transition: all 2s ease-in-out; } .shiftx-enter, .shiftx-leave-to /* .fade-leave-active below version 2.1.8 */ { transform : translateX(100px); } </style> <div id = "databinding"> <button v-on:click = "show = !show">Click Me</button> <transition name = "shiftx"> <p v-show = "show"> <img src = "images/img.jpg" style = "width:100px;height:100px;" /> </p> </transition> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { show:true }, methods : { } }); </script> </body> </html>
转换的名称是shiftx。变换属性用于使用以下代码将图像在 x 轴上移动 100px。
<style> .shiftx-enter-active, .shiftx-leave-active { transition: all 2s ease-in-out; } .shiftx-enter, .shiftx-leave-to /* .fade-leave-active below version 2.1.8 */ { transform : translateX(100px); } </style>
以下是输出。

单击该按钮后,图像将向右移动 100px,如下图所示。

动画片
动画的应用方式与过渡的完成方式相同。动画还具有需要声明才能发生效果的类。
让我们考虑一个例子来看看动画是如何工作的。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <style> .shiftx-enter-active { animation: shift-in 2s; } .shiftx-leave-active { animation: shift-in 2s reverse; } @keyframes shift-in { 0% {transform:rotateX(0deg);} 25% {transform:rotateX(90deg);} 50% {transform:rotateX(120deg);} 75% {transform:rotateX(180deg);} 100% {transform:rotateX(360deg);} } </style> <div id = "databinding"> <button v-on:click = "show = !show">Click Me</button> <transition name = "shiftx"> <p v-show = "show"> <img src = "images/img.jpg" style = "width:100px;height:100px;" /> </p> </transition> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { show:true }, methods : { } }); </script> </body> </html>
要应用动画,有与过渡相同的类。在上面的代码中,我们有一个包含在 p 标签中的图像,如下面的代码所示。
<transition name = "shiftx"> <p v-show = "show"><img src = "images/img.jpg" style = "width:100px;height:100px;" /></p> </transition>
转换的名称是shiftx。应用的类如下 -
<style> .shiftx-enter-active { animation: shift-in 2s; } .shiftx-leave-active { animation: shift-in 2s reverse; } @keyframes shift-in { 0% {transform:rotateX(0deg);} 25% {transform:rotateX(90deg);} 50% {transform:rotateX(120deg);} 75% {transform:rotateX(180deg);} 100% {transform:rotateX(360deg);} } </style>
该类以转换名称为前缀,即shiftx-enter-active 和.shiftx-leave-active。动画由 0% 到 100% 的关键帧定义。每个关键帧都定义了一个变换,如下面的代码所示。
@keyframes shift-in { 0% {transform:rotateX(0deg);} 25% {transform:rotateX(90deg);} 50% {transform:rotateX(120deg);} 75% {transform:rotateX(180deg);} 100% {transform:rotateX(360deg);} }
以下是输出。

单击按钮后,它会从 0 度旋转到 360 度并消失。

自定义转换类
VueJS 提供了自定义类的列表,可以将其作为属性添加到过渡元素。
- 进入班级
- 进入活跃班级
- 下课
- 离开活动班级
当我们想要使用外部 CSS 库(例如 animate.css)时,自定义类基本上就会发挥作用。
例子
<html> <head> <link href = "https://cdn.jsdelivr.net/npm/animate.css@3.5.1" rel = "stylesheet" type = "text/css"> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "animate" style = "text-align:center"> <button @click = "show = !show"><span style = "font-size:25px;">Animate</span></button> <transition name = "custom-classes-transition" enter-active-class = "animated swing" leave-active-class = "animated bounceIn"> <p v-if = "show"><span style = "font-size:25px;">Example</span></p> </transition> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#animate', data: { show: true } }); </script> </body> </html>
输出

输出

输出

上面的代码中应用了两个动画。一个进入活动类=“动画摆动”,另一个离开活动类=“动画弹跳”。我们正在使用自定义动画类来应用来自第三方库的动画。
明确的过渡持续时间
我们可以使用 VueJS 在元素上应用过渡和动画。Vue 等待 transionend 和animationend 事件来检测动画或转换是否完成。
有时,转换可能会导致延迟。在这种情况下,我们可以明确应用持续时间,如下所示。
<transition :duration = "1000"></transition> <transition :duration = "{ enter: 500, leave: 800 }">...</transition>
我们可以在过渡元素上使用带有 : 的持续时间属性,如上所示。如果需要分别指定进入和离开的时长,可以按照上面的代码来完成。
JavaScript 钩子
可以使用 JavaScript 事件将转换类作为方法进行调用。让我们考虑一个例子以便更好地理解。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <script src = "https://cdnjs.cloudflare.com/ajax/libs/velocity/1.2.3/velocity.min.js"></script> <div id = "example-4"> <button @click = "show = !show"> <span style = "font-size:25px;">Toggle</span> </button> <transition v-on:before-enter = "beforeEnter" v-on:enter = "enter" v-on:leave = "leave" v-bind:css = "false"> <p v-if = "show" style = "font-size:25px;">Animation Example with velocity</p> </transition> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#example-4', data: { show: false }, methods: { beforeEnter: function (el) { el.style.opacity = 0 }, enter: function (el, done) { Velocity(el, { opacity: 1, fontSize: '25px' }, { duration: 1000 }) Velocity(el, { fontSize: '10px' }, { complete: done }) }, leave: function (el, done) { Velocity(el, { translateX: '15px', rotateZ: '50deg' }, { duration: 1500 }) Velocity(el, { rotateZ: '100deg' }, { loop: 2 }) Velocity(el, { rotateZ: '45deg', translateY: '30px', translateX: '30px', opacity: 0 }, { complete: done }) } } }); </script> </body> </html>
输出


在上面的示例中,我们在过渡元素上使用 js 方法执行动画。
过渡方法的应用如下 -
<transition v-on:before-enter = "beforeEnter" v-on:enter = "enter" v-on:leave = "leave" v-bind:css = "false"> <p v-if = "show" style = "font-size:25px;">Animation Example with velocity</p> </transition>
添加了前缀v-on和调用该方法的事件的名称。这些方法在 Vue 实例中定义如下 -
methods: { beforeEnter: function (el) { el.style.opacity = 0 }, enter: function (el, done) { Velocity(el, { opacity: 1, fontSize: '25px' }, { duration: 1000 }) Velocity(el, { fontSize: '10px' }, { complete: done }) }, leave: function (el, done) { Velocity(el, { translateX: '15px', rotateZ: '50deg' }, { duration: 1500 }) Velocity(el, { rotateZ: '100deg' }, { loop: 2 }) Velocity(el, { rotateZ: '45deg', translateY: '30px', translateX: '30px', opacity: 0 }, { complete: done }) } }
这些方法中的每一种都应用了所需的转换。单击按钮时以及动画完成时都会应用不透明动画。第三方库用于动画。
在转换 v-bind:css = "false" 上添加了一个属性,这样做是为了让 Vue 理解它是一个 JavaScript 转换。
初始渲染时的过渡
为了在开始时添加动画,我们需要向过渡元素添加“appear”属性。
让我们看一个例子来更好地理解它。
例子
<html> <head> <link href = "https://cdn.jsdelivr.net/npm/animate.css@3.5.1" rel = "stylesheet" type = "text/css"> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "animate" style = "text-align:center"> <transition appear appear-class = "custom-appear-class" appear-active-class = "animated bounceIn"> <h1>BounceIn - Animation Example</h1> </transition> <transition appear appear-class = "custom-appear-class" appear-active-class = "animated swing"> <h1>Swing - Animation Example</h1> </transition> <transition appear appear-class = "custom-appear-class" appear-active-class = "animated rubberBand"> <h1>RubberBand - Animation Example</h1> </transition> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#animate', data: { show: true } }); </script> </body> </html>
在上面的示例中,我们使用了 animate.css 库中的三种不同的动画。我们已将外观添加到过渡元素中。
执行上述代码后,浏览器中的输出如下。

组件上的动画
我们可以使用以下代码包装组件的转换。我们在这里使用了动态组件。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> <link href = "https://cdn.jsdelivr.net/npm/animate.css@3.5.1" rel = "stylesheet" type = "text/css"> </head> <body> <div id = "databinding" style = "text-align:center;"> <transition appear appear-class = "custom-appear-class" appear-active-class = "animated wobble"> <component v-bind:is = "view"></component> </transition> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { view: 'component1' }, components: { 'component1': { template: '<div><span style = "font- size:25;color:red;">Animation on Components</span></div>' } } }); </script> </body> </html>
输出
