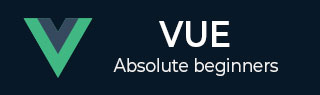
- VueJS Tutorial
- VueJS - Home
- VueJS - Overview
- VueJS - Environment Setup
- VueJS - Introduction
- VueJS - Instances
- VueJS - Template
- VueJS - Components
- VueJS - Computed Properties
- VueJS - Watch Property
- VueJS - Binding
- VueJS - Events
- VueJS - Rendering
- VueJS - Transition & Animation
- VueJS - Directives
- VueJS - Routing
- VueJS - Mixins
- VueJS - Render Function
- VueJS - Reactive Interface
- VueJS - Examples
- VueJS Useful Resources
- VueJS - Quick Guide
- VueJS - Useful Resources
- VueJS - Discussion
VueJS - 反应式界面
VueJS 提供了向属性添加反应性的选项,这些属性是动态添加的。考虑到我们已经创建了 vue 实例,需要添加 watch 属性。可以按如下方式完成 -
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "app"> <p style = "font-size:25px;">Counter: {{ counter }}</p> <button @click = "counter++" style = "font-size:25px;">Click Me</button> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#app', data: { counter: 1 } }); vm.$watch('counter', function(nval, oval) { alert('Counter is incremented :' + oval + ' to ' + nval + '!'); }); setTimeout( function(){ vm.counter = 20; },2000 ); </script> </body> </html>
数据对象中有一个属性计数器定义为1。当我们单击按钮时,计数器就会增加。
Vue 实例已经创建。要向其添加监视,我们需要执行以下操作 -
vm.$watch('counter', function(nval, oval) { alert('Counter is incremented :' + oval + ' to ' + nval + '!'); });
我们需要使用 $watch 在 vue 实例之外添加 watch。添加了一个警报,显示计数器属性的值更改。另外还添加了一个定时器函数,即setTimeout,将计数器值设置为20。
setTimeout( function(){ vm.counter = 20; },2000 );
每当计数器发生变化时,来自 watch 方法的警报就会被触发,如下面的屏幕截图所示。

VueJS 无法检测属性添加和删除。最好的方法是始终声明属性,这些属性需要在 Vue 实例中预先进行响应。如果我们需要在运行时添加属性,我们可以使用 Vue global、Vue.set 和 Vue.delete 方法。
Vue.set
此方法有助于设置对象的属性。它用于绕过 Vue 无法检测属性添加的限制。
句法
Vue.set( target, key, value )
在哪里,
target:可以是对象或数组
key : 可以是字符串或数字
值:可以是任何类型
让我们看一个例子。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "app"> <p style = "font-size:25px;">Counter: {{ products.id }}</p> <button @click = "products.id++" style = "font-size:25px;">Click Me</button> </div> <script type = "text/javascript"> var myproduct = {"id":1, name:"book", "price":"20.00"}; var vm = new Vue({ el: '#app', data: { counter: 1, products: myproduct } }); vm.products.qty = "1"; console.log(vm); vm.$watch('counter', function(nval, oval) { alert('Counter is incremented :' + oval + ' to ' + nval + '!'); }); </script> </body> </html>
在上面的示例中,使用以下代码在开始时创建了一个变量 myproduct。
var myproduct = {"id":1, name:"book", "price":"20.00"};
它被赋予 Vue 实例中的数据对象,如下所示 -
var vm = new Vue({ el: '#app', data: { counter: 1, products: myproduct } });
考虑一下,在创建 Vue 实例后,我们想要向 myproduct 数组添加一个属性。可以按如下方式完成 -
vm.products.qty = "1";
让我们看看控制台中的输出。

如上所示,在产品中添加了数量。get/set 方法基本上增加了反应性,可用于 id、名称和价格,但不可用于数量。
我们无法仅通过添加 vue 对象来实现反应性。VueJS 主要希望在开始时创建它的所有属性。但是,如果我们稍后需要添加它,我们可以使用 Vue.set。为此,我们需要使用vue全局来设置它,即Vue.set。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "app"> <p style = "font-size:25px;">Counter: {{ products.id }}</p> <button @click = "products.id++" style = "font-size:25px;">Click Me</button> </div> <script type = "text/javascript"> var myproduct = {"id":1, name:"book", "price":"20.00"}; var vm = new Vue({ el: '#app', data: { counter: 1, products: myproduct } }); Vue.set(myproduct, 'qty', 1); console.log(vm); vm.$watch('counter', function(nval, oval) { alert('Counter is incremented :' + oval + ' to ' + nval + '!'); }); </script> </body> </html>
我们使用 Vue.set 使用以下代码将数量添加到数组中。
Vue.set(myproduct, 'qty', 1);
我们已经对 vue 对象进行了控制台操作,以下是输出。

现在,我们可以看到使用 Vue.set 添加的数量的获取/设置。
Vue.删除
该函数用于动态删除属性。
例子
Vue.delete( target, key )
在哪里,
target:可以是对象或数组
key:可以是字符串或数字
要删除任何属性,我们可以使用 Vue.delete,如以下代码所示。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "app"> <p style = "font-size:25px;">Counter: {{ products.id }}</p> <button @click = "products.id++" style = "font-size:25px;">Click Me</button> </div> <script type = "text/javascript"> var myproduct = {"id":1, name:"book", "price":"20.00"}; var vm = new Vue({ el: '#app', data: { counter: 1, products: myproduct } }); Vue.delete(myproduct, 'price'); console.log(vm); vm.$watch('counter', function(nval, oval) { alert('Counter is incremented :' + oval + ' to ' + nval + '!'); }); </script> </body> </html>
在上面的示例中,我们使用 Vue.delete 使用以下代码从数组中删除价格。
Vue.delete(myproduct, 'price');
以下是我们在控制台中看到的输出。

删除后,我们只能看到id和name,价格也被删除了。我们还可以注意到 get/set 方法被删除了。