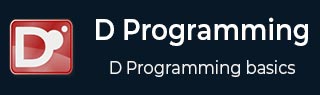
- D 编程基础
- D 编程 - 主页
- D 编程 - 概述
- D 编程 - 环境
- D 编程 - 基本语法
- D 编程 - 变量
- D 编程 - 数据类型
- D 编程 - 枚举
- D 编程 - 文字
- D 编程 - 运算符
- D 编程 - 循环
- D 编程 - 决策
- D 编程 - 函数
- D 编程 - 字符
- D 编程 - 字符串
- D 编程 - 数组
- D 编程 - 关联数组
- D 编程 - 指针
- D 编程 - 元组
- D 编程 - 结构
- D 编程 - 联合
- D 编程 - 范围
- D 编程 - 别名
- D 编程 - Mixins
- D 编程 - 模块
- D 编程 - 模板
- D 编程 - 不可变
- D 编程 - 文件 I/O
- D 编程 - 并发
- D 编程 - 异常处理
- D 编程 - 合同
- D - 条件编译
- D 编程 - 面向对象
- D 编程 - 类和对象
- D 编程 - 继承
- D 编程 - 重载
- D 编程 - 封装
- D 编程 - 接口
- D 编程 - 抽象类
- D 编程 - 有用的资源
- D 编程 - 快速指南
- D 编程 - 有用的资源
- D 编程 - 讨论
D 编程 - 接口
接口是一种强制从它继承的类必须实现某些函数或变量的方法。函数不能在接口中实现,因为它们始终在从接口继承的类中实现。
接口是使用interface关键字而不是class关键字创建的,尽管两者在很多方面都很相似。当你想从一个接口继承并且该类已经从另一个类继承时,你需要用逗号分隔类的名称和接口的名称。
让我们看一个解释接口使用的简单示例。
例子
import std.stdio; // Base class interface Shape { public: void setWidth(int w); void setHeight(int h); } // Derived class class Rectangle: Shape { int width; int height; public: void setWidth(int w) { width = w; } void setHeight(int h) { height = h; } int getArea() { return (width * height); } } void main() { Rectangle Rect = new Rectangle(); Rect.setWidth(5); Rect.setHeight(7); // Print the area of the object. writeln("Total area: ", Rect.getArea()); }
当上面的代码被编译并执行时,它会产生以下结果 -
Total area: 35
与 D 中的 Final 和 Static 函数的接口
接口可以具有最终方法和静态方法,其定义应包含在接口本身中。这些函数不能被派生类重写。下面显示了一个简单的示例。
例子
import std.stdio; // Base class interface Shape { public: void setWidth(int w); void setHeight(int h); static void myfunction1() { writeln("This is a static method"); } final void myfunction2() { writeln("This is a final method"); } } // Derived class class Rectangle: Shape { int width; int height; public: void setWidth(int w) { width = w; } void setHeight(int h) { height = h; } int getArea() { return (width * height); } } void main() { Rectangle rect = new Rectangle(); rect.setWidth(5); rect.setHeight(7); // Print the area of the object. writeln("Total area: ", rect.getArea()); rect.myfunction1(); rect.myfunction2(); }
当上面的代码被编译并执行时,它会产生以下结果 -
Total area: 35 This is a static method This is a final method