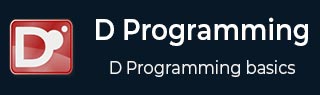
- D 编程基础
- D 编程 - 主页
- D 编程 - 概述
- D 编程 - 环境
- D 编程 - 基本语法
- D 编程 - 变量
- D 编程 - 数据类型
- D 编程 - 枚举
- D 编程 - 文字
- D 编程 - 运算符
- D 编程 - 循环
- D 编程 - 决策
- D 编程 - 函数
- D 编程 - 字符
- D 编程 - 字符串
- D 编程 - 数组
- D 编程 - 关联数组
- D 编程 - 指针
- D 编程 - 元组
- D 编程 - 结构
- D 编程 - 联合
- D 编程 - 范围
- D 编程 - 别名
- D 编程 - Mixins
- D 编程 - 模块
- D 编程 - 模板
- D 编程 - 不可变
- D 编程 - 文件 I/O
- D 编程 - 并发
- D 编程 - 异常处理
- D 编程 - 合同
- D - 条件编译
- D 编程 - 面向对象
- D 编程 - 类和对象
- D 编程 - 继承
- D 编程 - 重载
- D 编程 - 封装
- D 编程 - 接口
- D 编程 - 抽象类
- D 编程 - 有用的资源
- D 编程 - 快速指南
- D 编程 - 有用的资源
- D 编程 - 讨论
D 编程 - 重载
D 允许在同一作用域内为一个函数名或一个运算符指定多个定义,这分别称为函数重载和运算符重载。
重载声明是与同一作用域中的前一个声明同名声明的声明,只不过这两个声明具有不同的参数和明显不同的定义(实现)。
当您调用重载函数或运算符时,编译器通过将用于调用函数或运算符的参数类型与定义中指定的参数类型进行比较来确定要使用的最合适的定义。选择最合适的重载函数或运算符的过程称为重载决策。。
函数重载
您可以在同一范围内对同一函数名称有多个定义。函数的定义必须在参数列表中的参数类型和/或数量上有所不同。您不能重载仅返回类型不同的函数声明。
例子
以下示例使用相同的函数print()来打印不同的数据类型 -
import std.stdio; import std.string; class printData { public: void print(int i) { writeln("Printing int: ",i); } void print(double f) { writeln("Printing float: ",f ); } void print(string s) { writeln("Printing string: ",s); } }; void main() { printData pd = new printData(); // Call print to print integer pd.print(5); // Call print to print float pd.print(500.263); // Call print to print character pd.print("Hello D"); }
当上面的代码被编译并执行时,它会产生以下结果 -
Printing int: 5 Printing float: 500.263 Printing string: Hello D
运算符重载
您可以重新定义或重载 D 中可用的大多数内置运算符。因此,程序员也可以将运算符与用户定义的类型一起使用。
可以根据要重载的运算符使用字符串 op 后跟 Add、Sub 等来重载运算符。我们可以重载运算符 + 以添加两个框,如下所示。
Box opAdd(Box b) { Box box = new Box(); box.length = this.length + b.length; box.breadth = this.breadth + b.breadth; box.height = this.height + b.height; return box; }
以下示例显示使用成员函数的运算符重载的概念。这里一个对象作为参数传递,使用该对象访问其属性。可以使用此运算符访问调用此运算符的对象,如下所述 -
import std.stdio; class Box { public: double getVolume() { return length * breadth * height; } void setLength( double len ) { length = len; } void setBreadth( double bre ) { breadth = bre; } void setHeight( double hei ) { height = hei; } Box opAdd(Box b) { Box box = new Box(); box.length = this.length + b.length; box.breadth = this.breadth + b.breadth; box.height = this.height + b.height; return box; } private: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box }; // Main function for the program void main( ) { Box box1 = new Box(); // Declare box1 of type Box Box box2 = new Box(); // Declare box2 of type Box Box box3 = new Box(); // Declare box3 of type Box double volume = 0.0; // Store the volume of a box here // box 1 specification box1.setLength(6.0); box1.setBreadth(7.0); box1.setHeight(5.0); // box 2 specification box2.setLength(12.0); box2.setBreadth(13.0); box2.setHeight(10.0); // volume of box 1 volume = box1.getVolume(); writeln("Volume of Box1 : ", volume); // volume of box 2 volume = box2.getVolume(); writeln("Volume of Box2 : ", volume); // Add two object as follows: box3 = box1 + box2; // volume of box 3 volume = box3.getVolume(); writeln("Volume of Box3 : ", volume); }
当上面的代码被编译并执行时,它会产生以下结果 -
Volume of Box1 : 210 Volume of Box2 : 1560 Volume of Box3 : 5400
运算符重载类型
基本上,存在以下三种类型的运算符重载。