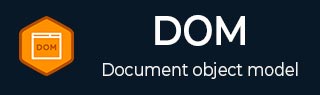
- XML DOM 基础知识
- XML DOM - 主页
- XML DOM - 概述
- XML DOM - 模型
- XML DOM - 节点
- XML DOM - 节点树
- XML DOM - 方法
- XML DOM - 加载
- XML DOM - 遍历
- XML DOM - 导航
- XML DOM - 访问
- XML DOM 操作
- XML DOM - 获取节点
- XML DOM - 设置节点
- XML DOM - 创建节点
- XML DOM - 添加节点
- XML DOM - 替换节点
- XML DOM - 删除节点
- XML DOM - 克隆节点
- XML DOM 对象
- DOM - 节点对象
- DOM - 节点列表对象
- DOM - 命名节点映射对象
- DOM - DOMI 实现
- DOM - 文档类型对象
- DOM - 处理指令
- DOM-实体对象
- DOM - 实体引用对象
- DOM - 表示法对象
- DOM - 元素对象
- DOM - 属性对象
- DOM - CDATASection 对象
- DOM - 评论对象
- DOM - XMLHttpRequest 对象
- DOM - DOMException 对象
- XML DOM 有用的资源
- XML DOM - 快速指南
- XML DOM - 有用的资源
- XML DOM - 讨论
DOM - XMLHttpRequest 对象
XMLHttpRequest 对象在网页的客户端和服务器端之间建立了一种媒介,许多脚本语言(如 JavaScript、JScript、VBScript 和其他 Web 浏览器)可以使用它来传输和操作 XML 数据。
使用 XMLHttpRequest 对象,可以更新网页的一部分,而无需重新加载整个页面,在页面加载后从服务器请求和接收数据,并将数据发送到服务器。
句法
XMLHttpRequest 对象可以按如下方式初始化 -
xmlhttp = new XMLHttpRequest();
要处理所有浏览器,包括 IE5 和 IE6,请检查浏览器是否支持 XMLHttpRequest 对象,如下所示 -
if(window.XMLHttpRequest) // for Firefox, IE7+, Opera, Safari, ... { xmlHttp = new XMLHttpRequest(); } else if(window.ActiveXObject) // for Internet Explorer 5 or 6 { xmlHttp = new ActiveXObject("Microsoft.XMLHTTP"); }
使用 XMLHttpRequest 对象加载 XML 文件的示例可以参考这里
方法
下表列出了 XMLHttpRequest 对象的方法 -
编号 | 方法及说明 |
---|---|
1 | 中止() 终止当前发出的请求。 |
2 | 获取所有响应头() 以字符串形式返回所有响应标头,如果未收到响应则返回 null。 |
3 | 获取响应头() 返回包含指定标头文本的字符串,如果尚未收到响应或响应中不存在标头,则返回 null。 |
4 | 打开(方法,url,异步,uname,pswd) 它与 Send 方法结合使用,将请求发送到服务器。open 方法指定以下参数 -
|
5 | 发送(字符串) 它用于与 Open 方法结合使用来发送请求。 |
6 | 设置请求头() 标头包含请求发送到的标签/值对。 |
属性
下表列出了 XMLHttpRequest 对象的属性 -
编号 | 属性及描述 |
---|---|
1 | 就绪状态改变 它是一个基于事件的属性,在每次状态更改时都会设置。 |
2 | 就绪状态 这描述了 XMLHttpRequest 对象的当前状态。ReadyState 属性有五种可能的状态 -
|
3 | 响应文本 当服务器的响应是文本文件时使用此属性。 |
4 | 响应XML 当服务器的响应是 XML 文件时,使用此属性。 |
5 | 地位 以数字形式给出 Http 请求对象的状态。例如,“404”或“200”。 |
6 | 状态文本 以字符串形式给出 Http 请求对象的状态。例如,“未找到”或“确定”。 |
例子
node.xml内容如下 -
<?xml version = "1.0"?> <Company> <Employee category = "Technical"> <FirstName>Tanmay</FirstName> <LastName>Patil</LastName> <ContactNo>1234567890</ContactNo> <Email>tanmaypatil@xyz.com</Email> </Employee> <Employee category = "Non-Technical"> <FirstName>Taniya</FirstName> <LastName>Mishra</LastName> <ContactNo>1234667898</ContactNo> <Email>taniyamishra@xyz.com</Email> </Employee> <Employee category = "Management"> <FirstName>Tanisha</FirstName> <LastName>Sharma</LastName> <ContactNo>1234562350</ContactNo> <Email>tanishasharma@xyz.com</Email> </Employee> </Company>
检索资源文件的具体信息
以下示例演示如何使用方法getResponseHeader()和属性readState检索资源文件的特定信息。
<!DOCTYPE html> <html> <head> <meta http-equiv = "content-type" content = "text/html; charset = iso-8859-2" /> <script> function loadXMLDoc() { var xmlHttp = null; if(window.XMLHttpRequest) // for Firefox, IE7+, Opera, Safari, ... { xmlHttp = new XMLHttpRequest(); } else if(window.ActiveXObject) // for Internet Explorer 5 or 6 { xmlHttp = new ActiveXObject("Microsoft.XMLHTTP"); } return xmlHttp; } function makerequest(serverPage, myDiv) { var request = loadXMLDoc(); request.open("GET", serverPage); request.send(null); request.onreadystatechange = function() { if (request.readyState == 4) { document.getElementById(myDiv).innerHTML = request.getResponseHeader("Content-length"); } } } </script> </head> <body> <button type = "button" onclick="makerequest('/dom/node.xml', 'ID')">Click me to get the specific ResponseHeader</button> <div id = "ID">Specific header information is returned.</div> </body> </html>
执行
将此文件保存为服务器路径上的elementattribute_removeAttributeNS.htm (此文件和 node_ns.xml 应位于服务器中的同一路径上)。我们将得到如下所示的输出 -
Before removing the attributeNS: en After removing the attributeNS: null
检索资源文件的标头信息
以下示例演示如何使用属性readState并使用方法getAllResponseHeaders()检索资源文件的标头信息。
<!DOCTYPE html> <html> <head> <meta http-equiv="content-type" content="text/html; charset=iso-8859-2" /> <script> function loadXMLDoc() { var xmlHttp = null; if(window.XMLHttpRequest) // for Firefox, IE7+, Opera, Safari, ... { xmlHttp = new XMLHttpRequest(); } else if(window.ActiveXObject) // for Internet Explorer 5 or 6 { xmlHttp = new ActiveXObject("Microsoft.XMLHTTP"); } return xmlHttp; } function makerequest(serverPage, myDiv) { var request = loadXMLDoc(); request.open("GET", serverPage); request.send(null); request.onreadystatechange = function() { if (request.readyState == 4) { document.getElementById(myDiv).innerHTML = request.getAllResponseHeaders(); } } } </script> </head> <body> <button type = "button" onclick = "makerequest('/dom/node.xml', 'ID')"> Click me to load the AllResponseHeaders</button> <div id = "ID"></div> </body> </html>
执行
将此文件保存为服务器路径上的http_allheader.html (此文件和 node.xml 应位于服务器中的同一路径上)。我们将得到如下所示的输出(取决于浏览器) -
Date: Sat, 27 Sep 2014 07:48:07 GMT Server: Apache Last-Modified: Wed, 03 Sep 2014 06:35:30 GMT Etag: "464bf9-2af-50223713b8a60" Accept-Ranges: bytes Vary: Accept-Encoding,User-Agent Content-Encoding: gzip Content-Length: 256 Content-Type: text/xml