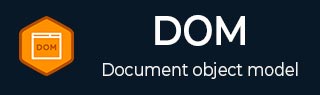
- XML DOM 基础知识
- XML DOM - 主页
- XML DOM - 概述
- XML DOM - 模型
- XML DOM - 节点
- XML DOM - 节点树
- XML DOM - 方法
- XML DOM - 加载
- XML DOM - 遍历
- XML DOM - 导航
- XML DOM - 访问
- XML DOM 操作
- XML DOM - 获取节点
- XML DOM - 设置节点
- XML DOM - 创建节点
- XML DOM - 添加节点
- XML DOM - 替换节点
- XML DOM - 删除节点
- XML DOM - 克隆节点
- XML DOM 对象
- DOM - 节点对象
- DOM - 节点列表对象
- DOM - 命名节点映射对象
- DOM - DOMI 实现
- DOM - 文档类型对象
- DOM - 处理指令
- DOM-实体对象
- DOM - 实体引用对象
- DOM - 表示法对象
- DOM - 元素对象
- DOM - 属性对象
- DOM - CDATASection 对象
- DOM - 评论对象
- DOM - XMLHttpRequest 对象
- DOM - DOMException 对象
- XML DOM 有用的资源
- XML DOM - 快速指南
- XML DOM - 有用的资源
- XML DOM - 讨论
XML DOM - 导航
到目前为止我们学习了 DOM 结构,如何加载和解析 XML DOM 对象以及遍历 DOM 对象。在这里,我们将了解如何在 DOM 对象中的节点之间导航。XML DOM 由节点的各种属性组成,这些属性帮助我们在节点中导航,例如 -
- 父节点
- 子节点
- 第一个孩子
- 最后一个孩子
- 下一个兄弟姐妹
- 上一个兄弟姐妹
下图是节点树图,显示了它与其他节点的关系。
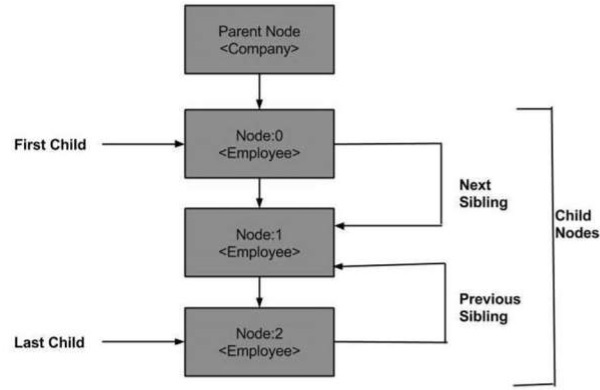
DOM - 父节点
该属性将父节点指定为节点对象。
例子
以下示例 (navigate_example.htm) 将 XML 文档 ( node.xml ) 解析为 XML DOM 对象。然后 DOM 对象通过子节点导航到父节点 -
<!DOCTYPE html> <html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; var y = xmlDoc.getElementsByTagName("Employee")[0]; document.write(y.parentNode.nodeName); </script> </body> </html>
正如您在上面的示例中看到的,子节点Employee导航到其父节点。
执行
在服务器路径上将此文件保存为navigate_example.html (此文件和node.xml应位于服务器中的同一路径上)。在输出中,我们得到Employee的父节点,即Company。
第一个孩子
该属性属于Node类型,表示 NodeList 中存在的第一个子名称。
例子
以下示例 (first_node_example.htm) 将 XML 文档 ( node.xml ) 解析为 XML DOM 对象,然后导航到 DOM 对象中存在的第一个子节点。
<!DOCTYPE html> <html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; function get_firstChild(p) { a = p.firstChild; while (a.nodeType != 1) { a = a.nextSibling; } return a; } var firstchild = get_firstChild(xmlDoc.getElementsByTagName("Employee")[0]); document.write(firstchild.nodeName); </script> </body> </html>
函数get_firstChild(p)用于避免空节点。它有助于从节点列表中获取第一个子元素。
x = get_firstChild(xmlDoc.getElementsByTagName("Employee")[0])获取标签名称Employee的第一个子节点。
执行
将此文件保存为服务器路径上的first_node_example.htm (此文件和node.xml应位于服务器中的同一路径上)。在输出中,我们得到Employee的第一个子节点, 即FirstName。
最后一个孩子
此属性属于Node类型,表示 NodeList 中存在的最后一个子名称。
例子
以下示例 (last_node_example.htm) 将 XML 文档 ( node.xml ) 解析为 XML DOM 对象,然后导航到 xml DOM 对象中存在的最后一个子节点。
<!DOCTYPE html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; function get_lastChild(p) { a = p.lastChild; while (a.nodeType != 1){ a = a.previousSibling; } return a; } var lastchild = get_lastChild(xmlDoc.getElementsByTagName("Employee")[0]); document.write(lastchild.nodeName); </script> </body> </html>
执行
将此文件保存为服务器路径上的last_node_example.htm (此文件和node.xml 应位于服务器中的同一路径上)。在输出中,我们得到Employee 的最后一个子节点,即Email。
下一个兄弟姐妹
该属性的类型为Node,代表下一个子元素,即 NodeList 中存在的指定子元素的下一个兄弟元素。
例子
以下示例 (nextSibling_example.htm) 将 XML 文档 ( node.xml ) 解析为 XML DOM 对象,该对象立即导航到 xml 文档中存在的下一个节点。
<!DOCTYPE html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; function get_nextSibling(p) { a = p.nextSibling; while (a.nodeType != 1) { a = a.nextSibling; } return a; } var nextsibling = get_nextSibling(xmlDoc.getElementsByTagName("FirstName")[0]); document.write(nextsibling.nodeName); </script> </body> </html>
执行
将此文件保存为服务器路径上的nextSibling_example.htm (此文件和 node.xml 应位于服务器中的同一路径上)。在输出中,我们得到FirstName 的下一个兄弟节点,即LastName。
以前的兄弟姐妹
该属性的类型为Node,表示前一个子元素,即 NodeList 中存在的指定子元素的前一个同级元素。
例子
以下示例 (previoussibling_example.htm) 将 XML 文档 ( node.xml ) 解析为 XML DOM 对象,然后导航 xml 文档中存在的最后一个子节点的 before 节点。
<!DOCTYPE html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; function get_previousSibling(p) { a = p.previousSibling; while (a.nodeType != 1) { a = a.previousSibling; } return a; } prevsibling = get_previousSibling(xmlDoc.getElementsByTagName("Email")[0]); document.write(prevsibling.nodeName); </script> </body> </html>
执行
将此文件保存为服务器路径上的previoussibling_example.htm (此文件和node.xml应位于服务器中的同一路径上)。在输出中,我们得到了Email的前一个同级节点,即ContactNo。