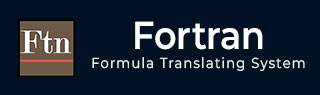
- Fortran 教程
- Fortran - 主页
- Fortran - 概述
- Fortran - 环境设置
- Fortran - 基本语法
- Fortran - 数据类型
- Fortran - 变量
- Fortran - 常量
- Fortran - 运算符
- Fortran - 决策
- Fortran - 循环
- Fortran - 数字
- Fortran - 字符
- Fortran - 字符串
- Fortran - 数组
- Fortran - 动态数组
- Fortran - 派生数据类型
- Fortran - 指针
- Fortran - 基本输入输出
- Fortran - 文件输入输出
- Fortran - 过程
- Fortran - 模块
- Fortran - 内在函数
- Fortran - 数值精度
- Fortran - 程序库
- Fortran - 编程风格
- Fortran - 调试程序
- Fortran 资源
- Fortran - 快速指南
- Fortran - 有用的资源
- Fortran - 讨论
Fortran - 模块
模块就像一个包,您可以在其中保存函数和子例程,以防您正在编写一个非常大的程序,或者您的函数或子例程可以在多个程序中使用。
模块为您提供了一种在多个文件之间拆分程序的方法。
模块用于 -
打包子程序、数据和接口块。
定义可供多个例程使用的全局数据。
声明可在您选择的任何例程中使用的变量。
将模块完全导入到另一个程序或子例程中以供使用。
模块的语法
模块由两部分组成 -
- 语句声明的规范部分
- a 包含子程序和函数定义的部分
模块的一般形式是 -
module name [statement declarations] [contains [subroutine and function definitions] ] end module [name]
在程序中使用模块
您可以通过 use 语句将模块合并到程序或子例程中 -
use name
请注意
您可以根据需要添加任意数量的模块,每个模块都位于单独的文件中并单独编译。
模块可以在各种不同的程序中使用。
一个模块可以在同一个程序中多次使用。
模块规范部分中声明的变量对于模块来说是全局的。
模块中声明的变量将成为使用该模块的任何程序或例程中的全局变量。
use 语句可以出现在主程序中,或者使用在特定模块中声明的例程或变量的任何其他子例程或模块中。
例子
下面的例子演示了这个概念 -
module constants implicit none real, parameter :: pi = 3.1415926536 real, parameter :: e = 2.7182818285 contains subroutine show_consts() print*, "Pi = ", pi print*, "e = ", e end subroutine show_consts end module constants program module_example use constants implicit none real :: x, ePowerx, area, radius x = 2.0 radius = 7.0 ePowerx = e ** x area = pi * radius**2 call show_consts() print*, "e raised to the power of 2.0 = ", ePowerx print*, "Area of a circle with radius 7.0 = ", area end program module_example
当您编译并执行上述程序时,它会产生以下结果 -
Pi = 3.14159274 e = 2.71828175 e raised to the power of 2.0 = 7.38905573 Area of a circle with radius 7.0 = 153.938049
模块中变量和子例程的可访问性
默认情况下,模块中的所有变量和子例程都可通过use语句供使用模块代码的程序使用。
但是,您可以使用私有和公共属性来控制模块代码的可访问性。当您将某些变量或子例程声明为私有时,它在模块外部不可用。
例子
下面的例子说明了这个概念 -
在前面的示例中,我们有两个模块变量,e和pi。让我们将它们设为私有并观察输出 -
module constants implicit none real, parameter,private :: pi = 3.1415926536 real, parameter, private :: e = 2.7182818285 contains subroutine show_consts() print*, "Pi = ", pi print*, "e = ", e end subroutine show_consts end module constants program module_example use constants implicit none real :: x, ePowerx, area, radius x = 2.0 radius = 7.0 ePowerx = e ** x area = pi * radius**2 call show_consts() print*, "e raised to the power of 2.0 = ", ePowerx print*, "Area of a circle with radius 7.0 = ", area end program module_example
当您编译并执行上述程序时,它会给出以下错误消息 -
ePowerx = e ** x 1 Error: Symbol 'e' at (1) has no IMPLICIT type main.f95:19.13: area = pi * radius**2 1 Error: Symbol 'pi' at (1) has no IMPLICIT type
由于e和pi都被声明为私有,因此程序 module_example 无法再访问这些变量。
但是,其他模块子例程可以访问它们 -
module constants implicit none real, parameter,private :: pi = 3.1415926536 real, parameter, private :: e = 2.7182818285 contains subroutine show_consts() print*, "Pi = ", pi print*, "e = ", e end subroutine show_consts function ePowerx(x)result(ePx) implicit none real::x real::ePx ePx = e ** x end function ePowerx function areaCircle(r)result(a) implicit none real::r real::a a = pi * r**2 end function areaCircle end module constants program module_example use constants implicit none call show_consts() Print*, "e raised to the power of 2.0 = ", ePowerx(2.0) print*, "Area of a circle with radius 7.0 = ", areaCircle(7.0) end program module_example
当您编译并执行上述程序时,它会产生以下结果 -
Pi = 3.14159274 e = 2.71828175 e raised to the power of 2.0 = 7.38905573 Area of a circle with radius 7.0 = 153.938049