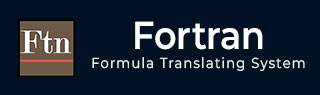
- Fortran 教程
- Fortran - 主页
- Fortran - 概述
- Fortran - 环境设置
- Fortran - 基本语法
- Fortran - 数据类型
- Fortran - 变量
- Fortran - 常量
- Fortran - 运算符
- Fortran - 决策
- Fortran - 循环
- Fortran - 数字
- Fortran - 字符
- Fortran - 字符串
- Fortran - 数组
- Fortran - 动态数组
- Fortran - 派生数据类型
- Fortran - 指针
- Fortran - 基本输入输出
- Fortran - 文件输入输出
- Fortran - 过程
- Fortran - 模块
- Fortran - 内在函数
- Fortran - 数值精度
- Fortran - 程序库
- Fortran - 编程风格
- Fortran - 调试程序
- Fortran 资源
- Fortran - 快速指南
- Fortran - 有用的资源
- Fortran - 讨论
Fortran - 字符串
Fortran 语言可以将字符视为单个字符或连续的字符串。
字符串的长度可能只有一个字符,甚至可能为零。在 Fortran 中,字符常量在一对双引号或单引号之间给出。
内在数据类型字符存储字符和字符串。字符串的长度可以通过len 说明符指定。如果没有指定长度,则为 1。您可以通过位置引用字符串中的单个字符;最左边的字符位于位置 1。
字符串声明
声明字符串与其他变量相同 -
type-specifier :: variable_name
例如,
Character(len = 20) :: firstname, surname
您可以分配一个值,例如
character (len = 40) :: name name = “Zara Ali”
以下示例演示了字符数据类型的声明和使用 -
program hello implicit none character(len = 15) :: surname, firstname character(len = 6) :: title character(len = 25)::greetings title = 'Mr.' firstname = 'Rowan' surname = 'Atkinson' greetings = 'A big hello from Mr. Beans' print *, 'Here is', title, firstname, surname print *, greetings end program hello
当您编译并执行上述程序时,它会产生以下结果 -
Here isMr. Rowan Atkinson A big hello from Mr. Bean
字符串连接
连接运算符//,连接字符串。
以下示例演示了这一点 -
program hello implicit none character(len = 15) :: surname, firstname character(len = 6) :: title character(len = 40):: name character(len = 25)::greetings title = 'Mr.' firstname = 'Rowan' surname = 'Atkinson' name = title//firstname//surname greetings = 'A big hello from Mr. Beans' print *, 'Here is', name print *, greetings end program hello
当您编译并执行上述程序时,它会产生以下结果 -
Here is Mr. Rowan Atkinson A big hello from Mr. Bean
提取子串
在 Fortran 中,您可以通过对字符串进行索引来从字符串中提取子字符串,并在一对括号中给出子字符串的开始和结束索引。这称为范围说明符。
以下示例显示如何从字符串“hello world”中提取子字符串“world” -
program subString character(len = 11)::hello hello = "Hello World" print*, hello(7:11) end program subString
当您编译并执行上述程序时,它会产生以下结果 -
World
例子
以下示例使用date_and_time函数给出日期和时间字符串。我们使用范围说明符来分别提取年、日、月、小时、分钟和秒信息。
program datetime implicit none character(len = 8) :: dateinfo ! ccyymmdd character(len = 4) :: year, month*2, day*2 character(len = 10) :: timeinfo ! hhmmss.sss character(len = 2) :: hour, minute, second*6 call date_and_time(dateinfo, timeinfo) ! let’s break dateinfo into year, month and day. ! dateinfo has a form of ccyymmdd, where cc = century, yy = year ! mm = month and dd = day year = dateinfo(1:4) month = dateinfo(5:6) day = dateinfo(7:8) print*, 'Date String:', dateinfo print*, 'Year:', year print *,'Month:', month print *,'Day:', day ! let’s break timeinfo into hour, minute and second. ! timeinfo has a form of hhmmss.sss, where h = hour, m = minute ! and s = second hour = timeinfo(1:2) minute = timeinfo(3:4) second = timeinfo(5:10) print*, 'Time String:', timeinfo print*, 'Hour:', hour print*, 'Minute:', minute print*, 'Second:', second end program datetime
当您编译并执行上述程序时,它会给出详细的日期和时间信息 -
Date String: 20140803 Year: 2014 Month: 08 Day: 03 Time String: 075835.466 Hour: 07 Minute: 58 Second: 35.466
修剪琴弦
修剪函数接受一个字符串,并在删除所有尾随空格后返回输入字符串。
例子
program trimString implicit none character (len = *), parameter :: fname="Susanne", sname="Rizwan" character (len = 20) :: fullname fullname = fname//" "//sname !concatenating the strings print*,fullname,", the beautiful dancer from the east!" print*,trim(fullname),", the beautiful dancer from the east!" end program trimString
当您编译并执行上述程序时,它会产生以下结果 -
Susanne Rizwan , the beautiful dancer from the east! Susanne Rizwan, the beautiful dancer from the east!
琴弦的左右调整
函数adjustmentl接受一个字符串并通过删除前导空格并将其附加为尾随空格来返回它。
函数调整器接受一个字符串并通过删除尾随空格并将其附加为前导空格来返回它。
例子
program hello implicit none character(len = 15) :: surname, firstname character(len = 6) :: title character(len = 40):: name character(len = 25):: greetings title = 'Mr. ' firstname = 'Rowan' surname = 'Atkinson' greetings = 'A big hello from Mr. Beans' name = adjustl(title)//adjustl(firstname)//adjustl(surname) print *, 'Here is', name print *, greetings name = adjustr(title)//adjustr(firstname)//adjustr(surname) print *, 'Here is', name print *, greetings name = trim(title)//trim(firstname)//trim(surname) print *, 'Here is', name print *, greetings end program hello
当您编译并执行上述程序时,它会产生以下结果 -
Here is Mr. Rowan Atkinson A big hello from Mr. Bean Here is Mr. Rowan Atkinson A big hello from Mr. Bean Here is Mr.RowanAtkinson A big hello from Mr. Bean
在字符串中搜索子字符串
索引函数接受两个字符串并检查第二个字符串是否是第一个字符串的子字符串。如果第二个参数是第一个参数的子字符串,则返回一个整数,该整数是第一个字符串中第二个字符串的起始索引,否则返回零。
例子
program hello implicit none character(len=30) :: myString character(len=10) :: testString myString = 'This is a test' testString = 'test' if(index(myString, testString) == 0)then print *, 'test is not found' else print *, 'test is found at index: ', index(myString, testString) end if end program hello
当您编译并执行上述程序时,它会产生以下结果 -
test is found at index: 11