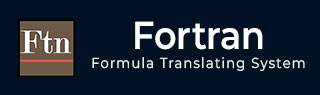
- Fortran 教程
- Fortran - 主页
- Fortran - 概述
- Fortran - 环境设置
- Fortran - 基本语法
- Fortran - 数据类型
- Fortran - 变量
- Fortran - 常量
- Fortran - 运算符
- Fortran - 决策
- Fortran - 循环
- Fortran - 数字
- Fortran - 字符
- Fortran - 字符串
- Fortran - 数组
- Fortran - 动态数组
- Fortran - 派生数据类型
- Fortran - 指针
- Fortran - 基本输入输出
- Fortran - 文件输入输出
- Fortran - 过程
- Fortran - 模块
- Fortran - 内在函数
- Fortran - 数值精度
- Fortran - 程序库
- Fortran - 编程风格
- Fortran - 调试程序
- Fortran 资源
- Fortran - 快速指南
- Fortran - 有用的资源
- Fortran - 讨论
Fortran - 过程
过程是一组执行明确定义的任务并且可以从程序中调用的语句。信息(或数据)作为参数传递给调用程序,传递给过程。
有两种类型的程序 -
- 功能
- 子程序
功能
函数是返回单个数量的过程。函数不应修改其参数。
返回的数量称为函数值,由函数名称表示。
句法
函数的语法如下 -
function name(arg1, arg2, ....) [declarations, including those for the arguments] [executable statements] end function [name]
以下示例演示了一个名为area_of_circle 的函数。它计算半径为 r 的圆的面积。
program calling_func real :: a a = area_of_circle(2.0) Print *, "The area of a circle with radius 2.0 is" Print *, a end program calling_func ! this function computes the area of a circle with radius r function area_of_circle (r) ! function result implicit none ! dummy arguments real :: area_of_circle ! local variables real :: r real :: pi pi = 4 * atan (1.0) area_of_circle = pi * r**2 end function area_of_circle
当您编译并执行上述程序时,它会产生以下结果 -
The area of a circle with radius 2.0 is 12.5663710
请注意 -
您必须在主程序和过程中指定隐式 none 。
被调用函数中的参数 r 称为虚拟参数。
结果选项
如果您希望返回值以函数名称以外的其他名称存储,则可以使用result选项。
您可以将返回变量名称指定为 -
function name(arg1, arg2, ....) result (return_var_name) [declarations, including those for the arguments] [executable statements] end function [name]
子程序
子例程不返回值,但可以修改其参数。
句法
subroutine name(arg1, arg2, ....) [declarations, including those for the arguments] [executable statements] end subroutine [name]
调用子程序
您需要使用call语句调用子例程。
以下示例演示了子例程交换的定义和使用,该子例程交换更改了其参数的值。
program calling_func implicit none real :: a, b a = 2.0 b = 3.0 Print *, "Before calling swap" Print *, "a = ", a Print *, "b = ", b call swap(a, b) Print *, "After calling swap" Print *, "a = ", a Print *, "b = ", b end program calling_func subroutine swap(x, y) implicit none real :: x, y, temp temp = x x = y y = temp end subroutine swap
当您编译并执行上述程序时,它会产生以下结果 -
Before calling swap a = 2.00000000 b = 3.00000000 After calling swap a = 3.00000000 b = 2.00000000
指定参数的意图
Intent 属性允许您指定在过程中使用参数的意图。下表提供了意图属性的值 -
价值 | 用作 | 解释 |
---|---|---|
在 | 意图(中) | 用作输入值,在函数中不改变 |
出去 | 意图(输出) | 用作输出值,它们被覆盖 |
进出 | 意图(输入输出) | 参数被使用和覆盖 |
下面的例子演示了这个概念 -
program calling_func implicit none real :: x, y, z, disc x = 1.0 y = 5.0 z = 2.0 call intent_example(x, y, z, disc) Print *, "The value of the discriminant is" Print *, disc end program calling_func subroutine intent_example (a, b, c, d) implicit none ! dummy arguments real, intent (in) :: a real, intent (in) :: b real, intent (in) :: c real, intent (out) :: d d = b * b - 4.0 * a * c end subroutine intent_example
当您编译并执行上述程序时,它会产生以下结果 -
The value of the discriminant is 17.0000000
递归过程
当编程语言允许您在同一函数内调用函数时,就会发生递归。这称为函数的递归调用。
当过程直接或间接调用自身时,称为递归过程。您应该在声明之前添加“recursive”一词来声明此类过程。
当递归使用函数时,必须使用结果选项。
以下是一个示例,它使用递归过程计算给定数字的阶乘 -
program calling_func implicit none integer :: i, f i = 15 Print *, "The value of factorial 15 is" f = myfactorial(15) Print *, f end program calling_func ! computes the factorial of n (n!) recursive function myfactorial (n) result (fac) ! function result implicit none ! dummy arguments integer :: fac integer, intent (in) :: n select case (n) case (0:1) fac = 1 case default fac = n * myfactorial (n-1) end select end function myfactorial
内部程序
当一个过程包含在一个程序中时,它被称为该程序的内部过程。包含内部过程的语法如下 -
program program_name implicit none ! type declaration statements ! executable statements . . . contains ! internal procedures . . . end program program_name
下面的例子演示了这个概念 -
program mainprog implicit none real :: a, b a = 2.0 b = 3.0 Print *, "Before calling swap" Print *, "a = ", a Print *, "b = ", b call swap(a, b) Print *, "After calling swap" Print *, "a = ", a Print *, "b = ", b contains subroutine swap(x, y) real :: x, y, temp temp = x x = y y = temp end subroutine swap end program mainprog
当您编译并执行上述程序时,它会产生以下结果 -
Before calling swap a = 2.00000000 b = 3.00000000 After calling swap a = 3.00000000 b = 2.00000000