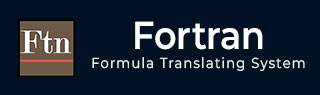
- Fortran 教程
- Fortran - 主页
- Fortran - 概述
- Fortran - 环境设置
- Fortran - 基本语法
- Fortran - 数据类型
- Fortran - 变量
- Fortran - 常量
- Fortran - 运算符
- Fortran - 决策
- Fortran - 循环
- Fortran - 数字
- Fortran - 字符
- Fortran - 字符串
- Fortran - 数组
- Fortran - 动态数组
- Fortran - 派生数据类型
- Fortran - 指针
- Fortran - 基本输入输出
- Fortran - 文件输入输出
- Fortran - 过程
- Fortran - 模块
- Fortran - 内在函数
- Fortran - 数值精度
- Fortran - 程序库
- Fortran - 编程风格
- Fortran - 调试程序
- Fortran 资源
- Fortran - 快速指南
- Fortran - 有用的资源
- Fortran - 讨论
Fortran - 操作函数
操纵函数是移位函数。移位函数返回数组的形状不变,但移动元素。
先生编号 | 功能说明 |
---|---|
1 | cshift(数组、移位、暗淡) 如果shift为正,则通过向左移动位置,如果为负,则通过向右移动位置来执行循环移位。如果数组是一个向量,则移位将以自然的方式完成,如果它是一个更高等级的数组,则移位将发生在沿维度 dim 的所有部分中。如果缺少 dim ,则将其视为 1,在其他情况下,它必须是 1 和 n 之间的标量整数(其中 n 等于 array 的秩)。参数 shift 是标量整数或阶为 n-1 的整数数组,并且与数组的形状相同,除了沿着维度 dim(由于阶较低而被删除)。因此,不同的部分可以沿不同的方向并以不同数量的位置移动。 |
2 | eoshift(数组,移位,边界,暗淡) 这是结束班次。如果shift为正,则执行左移;如果为负,则执行右移。从边界中取出新元素,而不是移出元素。如果数组是一个向量,则移位是以自然的方式完成的,如果它是一个更高阶的数组,则所有部分上的移位都是沿着维度 dim 进行的。如果缺少 dim,则将其视为 1,在其他情况下,它必须具有 1 和 n 之间的标量整数值(其中 n 等于数组的秩)。如果数组的秩为 1,则参数 shift 是一个标量整数,在其他情况下,它可以是一个标量整数或一个秩为 n-1 的整数数组,并且与数组 array 具有相同的形状,除了沿维度 dim(已删除)因为级别较低)。 |
3 | 转置(矩阵) 它转置一个矩阵,该矩阵是一个 2 阶数组。它替换矩阵中的行和列。 |
例子
下面的例子演示了这个概念 -
program arrayShift implicit none real, dimension(1:6) :: a = (/ 21.0, 22.0, 23.0, 24.0, 25.0, 26.0 /) real, dimension(1:6) :: x, y write(*,10) a x = cshift ( a, shift = 2) write(*,10) x y = cshift (a, shift = -2) write(*,10) y x = eoshift ( a, shift = 2) write(*,10) x y = eoshift ( a, shift = -2) write(*,10) y 10 format(1x,6f6.1) end program arrayShift
当上面的代码被编译并执行时,它会产生以下结果 -
21.0 22.0 23.0 24.0 25.0 26.0 23.0 24.0 25.0 26.0 21.0 22.0 25.0 26.0 21.0 22.0 23.0 24.0 23.0 24.0 25.0 26.0 0.0 0.0 0.0 0.0 21.0 22.0 23.0 24.0
例子
以下示例演示了矩阵的转置 -
program matrixTranspose implicit none interface subroutine write_matrix(a) integer, dimension(:,:) :: a end subroutine write_matrix end interface integer, dimension(3,3) :: a, b integer :: i, j do i = 1, 3 do j = 1, 3 a(i, j) = i end do end do print *, 'Matrix Transpose: A Matrix' call write_matrix(a) b = transpose(a) print *, 'Transposed Matrix:' call write_matrix(b) end program matrixTranspose subroutine write_matrix(a) integer, dimension(:,:) :: a write(*,*) do i = lbound(a,1), ubound(a,1) write(*,*) (a(i,j), j = lbound(a,2), ubound(a,2)) end do end subroutine write_matrix
当上面的代码被编译并执行时,它会产生以下结果 -
Matrix Transpose: A Matrix 1 1 1 2 2 2 3 3 3 Transposed Matrix: 1 2 3 1 2 3 1 2 3
fortran_arrays.htm