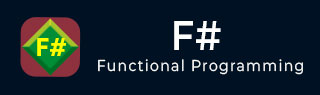
- F# 基础教程
- F# - 主页
- F# - 概述
- F# - 环境设置
- F# - 程序结构
- F# - 基本语法
- F# - 数据类型
- F# - 变量
- F# - 运算符
- F# - 决策
- F# - 循环
- F# - 函数
- F# - 字符串
- F# - 选项
- F# - 元组
- F# - 记录
- F# - 列表
- F# - 序列
- F# - 集
- F# - 地图
- F# - 受歧视的工会
- F# - 可变数据
- F# - 数组
- F# - 可变列表
- F# - 可变字典
- F# - 基本 I/O
- F# - 泛型
- F# - 代表
- F# - 枚举
- F# - 模式匹配
- F# - 异常处理
- F# - 类
- F# - 结构
- F# - 运算符重载
- F# - 继承
- F# - 接口
- F# - 事件
- F# - 模块
- F# - 命名空间
- F# 有用资源
- F# - 快速指南
- F# - 有用的资源
- F# - 讨论
F# - 运算符重载
您可以重新定义或重载 F# 中可用的大多数内置运算符。因此,程序员也可以将运算符与用户定义的类型一起使用。
运算符是具有特殊名称的函数,用括号括起来。它们必须被定义为静态类成员。与任何其他函数一样,重载运算符具有返回类型和参数列表。
以下示例显示了复数上的 + 运算符 -
//overloading + operator static member (+) (a : Complex, b: Complex) = Complex(a.x + b.x, a.y + b.y)
上面的函数为用户定义的类 Complex 实现了加法运算符 (+)。它将两个对象的属性相加并返回结果 Complex 对象。
运算符重载的实现
以下程序显示了完整的实现 -
//implementing a complex class with +, and - operators //overloaded type Complex(x: float, y : float) = member this.x = x member this.y = y //overloading + operator static member (+) (a : Complex, b: Complex) = Complex(a.x + b.x, a.y + b.y) //overloading - operator static member (-) (a : Complex, b: Complex) = Complex(a.x - b.x, a.y - b.y) // overriding the ToString method override this.ToString() = this.x.ToString() + " " + this.y.ToString() //Creating two complex numbers let c1 = Complex(7.0, 5.0) let c2 = Complex(4.2, 3.1) // addition and subtraction using the //overloaded operators let c3 = c1 + c2 let c4 = c1 - c2 //printing the complex numbers printfn "%s" (c1.ToString()) printfn "%s" (c2.ToString()) printfn "%s" (c3.ToString()) printfn "%s" (c4.ToString())
当您编译并执行该程序时,它会产生以下输出 -
7 5 4.2 3.1 11.2 8.1 2.8 1.9