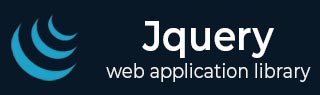
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 回调函数
jQuery 回调函数是一个仅在当前效果完成后才会执行的函数。本教程将向您解释什么是jQuery 回调函数以及为什么使用它们。
以下是任何 jQuery 效果方法的简单语法:
$(selector).effectName(speed, callback);
如果我们更详细一点,那么 jQuery 回调函数将编写如下:
$(selector).effectName(speed, function(){ <!-- function body --> });
没有回调函数的示例
首先,我们来看一个不使用回调函数的 jQuery 程序,因此即使在隐藏效果完成之前,也会显示警报消息。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("div").click(function(){ $(this).hide(1000); alert("I'm hidden now"); }); }); </script> <style> div{ margin:10px;padding:12px; border:2px solid #666; width:60px; cursor:pointer} </style> </head> <body> <p>Click on any of the squares to see the result:</p> <div>Hide Me</div> <div>Hide Me</div> <div>Hide Me</div> </body> </html>
jQuery 回调函数
由于 Javascript (jQuery) 代码执行的异步特性,需要 jQuery 回调函数。jQuery 效果可能需要一些时间才能完成,因此当效果仍在执行时,下一行代码可能会被执行。为了处理代码的异步执行,jQuery 允许在所有效果方法中传递回调函数,该回调函数的目的是仅在效果完成时执行。
例子
让我们再次重写上面的例子,这次我们使用了隐藏效果完成后执行的回调函数:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("div").click(function(){ $(this).hide(1000, function(){ alert("I'm hidden now"); }); }); }); </script> <style> div{ margin:10px;padding:12px; border:2px solid #666; width:60px; cursor:pointer} </style> </head> <body> <p>Click on any of the squares to see the result:</p> <div>Hide Me</div> <div>Hide Me</div> <div>Hide Me</div> </body> </html>
带动画的回调
jQuery animate()方法还提供了使用回调函数的功能。
例子
以下示例使用了动画效果完成后执行的回调函数:
<html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#right").click(function(){ $("div").animate({left: '250px'}, 1000, function(){ alert("I have reached to the right"); }); }); $("#left").click(function(){ $("div").animate({left: '0px'}, 1000, function(){ alert("I have reached to the left"); }); }); }); </script> <style> button{width:100px;cursor:pointer} #box{position:relative;margin:3px;padding:12px;height:100px; width:180px;background-color:#9c9cff;} </style> </head> <body> <p>Click on Left or Right button to see the result:</p> <div id="box">This is Box</div> <button id="right">Right Move</button> <button id="left">Left Move</button> </body> </html>