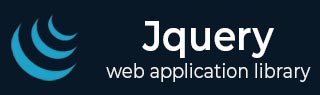
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 语法
jQuery 用于从 HTML 文档中选择任何 HTML 元素,然后对该所选元素执行任何操作。要选择 HTML 元素,需要使用 jQuery 选择器,我们将在下一章详细研究这些选择器。现在让我们看一下基本的jQuery 语法,以查找、选择或查询元素,然后对所选元素执行操作。
文件就绪事件
在研究jQuery 语法之前,让我们尝试了解什么是文档就绪事件。实际上,在执行任何 jQuery 语句之前,我们希望等待文档完全加载。这是因为 jQuery 是在 DOM 上工作的,如果在执行 jQuery 语句之前没有完整的 DOM,那么我们将不会得到想要的结果。
以下是文档就绪事件的基本语法:
$(document).ready(function(){ // jQuery code goes here... });
或者,您也可以对文档就绪事件使用以下语法:
$(function(){ // jQuery code goes here... });
您应该始终将文档就绪事件块保留在<script>...</script>标记内,并且可以在关闭之前将此脚本标记保留在<head>...</head>标记内或页面底部<正文>标签。
您可以使用这两种语法中的任何一种将 jQuery 代码保留在块内,该代码仅在下载完整 DOM 并准备好解析时才会执行。
jQuery 语法
以下是选择 HTML 元素然后对所选元素执行某些操作的基本语法:
$(document).ready(function(){ $(selector).action() });
任何 jQuery 语句都以美元符号$开头,然后我们将选择器放在大括号()内。此语法$(selector)足以返回选定的 HTML 元素,但如果您必须对选定的元素执行任何操作,则需要action()部分。
工厂函数$()是jQuery()函数的同义词。因此,如果您使用任何其他 JavaScript 库,其中 $ 符号与其他内容冲突,那么您可以用jQuery 名称替换$符号,并且可以使用函数jQuery()而不是$()。
例子
下面是几个例子来说明基本的 jQuery 语法。以下示例将从 HTML 文档中选择所有<p>元素并隐藏这些元素。尝试单击该图标来运行以下 jQuery 代码:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("p").hide() }); </script> </head> <body> <h1>jQuery Basic Syntax</h1> <p>This is p tag</p> <p>This is another p tag</p> <span>This is span tag</span> <div>This is div tag</div> </body> </html>
让我们使用jQuery()方法而不是$()重写上面的示例:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { jQuery("p").hide() }); </script> </head> <body> <h1>jQuery Basic Syntax</h1> <p>This is p tag</p> <p>This is another p tag</p> <span>This is span tag</span> <div>This is div tag</div> </body> </html>
以下是将所有<h1>元素的颜色更改为红色的 jQuery 语法。尝试单击该图标来运行以下 jQuery 代码:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("h1").css("color", "red") }); </script> </head> <body> <h1>jQuery Basic Syntax</h1> <p>This is p tag</p> <span>This is span tag</span> <div>This is div tag</div> </body> </html>
类似地,您可以更改所有类为“红色”的元素的颜色。尝试单击该图标来运行以下 jQuery 代码:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $(".red").css("color", "red") }); </script> </head> <body> <h1>jQuery Basic Syntax</h1> <p>This is p tag</p> <span>This is span tag</span> <div class="red">This is div tag</div> </body> </html>
到目前为止,我们已经看到了 jQuery 语法的非常基本的示例,可以让您清楚地了解 jQuery 到底如何在 HTML 文档上工作。您可以修改上面框中给出的代码,然后尝试运行这些程序以查看它们的实际运行情况。