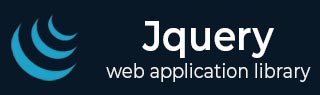
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 遍历后代
jQuery 提供了在 DOM 树内部向下遍历以查找给定元素的后代的方法。这些方法可用于查找 DOM 内给定元素的子元素、孙元素、曾孙元素等。
DOM树内部向下遍历有以下三种方法:
Children() - 返回匹配元素的所有直接子元素。
find() - 返回匹配元素的所有后代元素。
child ()方法与find()的不同之处在于,children()仅在 DOM 树中向下遍历单个级别,而find()方法可以向下遍历多个级别以选择后代元素(子元素、孙子元素、曾孙元素等)。以及。
jQuery Children() 方法
jQuery Children()方法返回匹配元素的所有直接子元素。以下是该方法的简单语法:
$(selector).children([filter])
我们可以选择在方法中提供一个过滤器选择器。如果提供了过滤器,将通过测试元素是否匹配来过滤元素。
概要
考虑以下 HTML 内容:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> <div class="grand-parent"> <ul class="parent"> <li class="child-three">Child Three</li> <li class="child-four">Child Four</li> </ul> </div> </div>
现在,如果我们使用Children()方法,如下所示:
$( ".great-grand-parent" ).children().css( "border", "2px solid red" );
它将产生以下结果:
<div class="great-grand-parent"> <div class="grand-parent" style="border:2px solid red"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> <div class="grand-parent" style="border:2px solid red"> <ul class="parent"> <li class="child-three">Child Three</li> <li class="child-four">Child Four</li> </ul> </div> </div>
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".great-grand-parent" ).children().css( "border", "2px solid red" ); }); }); </script> <style> .great-grand-parent *{display:block; border:2px solid #aaa; color:#aaa; padding:5px; margin:5px;} </style> </head> <body> <div class="great-grand-parent"> <div class="grand-parent" style="width:500px;"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> <div class="grand-parent" style="width:500px;"> <ul class="parent"> <li class="child-three">Child Three</li> <li class="child-four">Child Four</li> </ul> </div> </div> <br> <button>Mark Children</button> </body> </html>
jQuery find() 方法
jQuery find()方法返回匹配元素的所有后代。以下是该方法的简单语法:
$(selector).find([ilter)
在此方法中,过滤器选择器是必需的。要返回匹配元素的所有后代,我们需要将*作为过滤器传递,否则如果过滤器作为元素提供,则将通过测试元素是否匹配来过滤元素。
概要
考虑以下 HTML 内容:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> <div class="grand-parent"> <ul class="parent"> <li class="child-three">Child Three</li> <li class="child-four">Child Four</li> </ul> </div> </div>
现在,如果我们使用find("li")方法,如下所示:
$( ".grand-parent" ).find("li").css( "border", "2px solid red" );
它将产生以下结果:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent"> <li class="child-one" style="border:2px solid red">Child One</li> <li class="child-two" style="border:2px solid red">Child Two</li> </ul> </div> <div class="grand-parent" style="border:2px solid red"> <ul class="parent"> <li class="child-three" style="border:2px solid red">Child Three</li> <li class="child-four" style="border:2px solid red">Child Four</li> </ul> </div> </div>
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".grand-parent" ).find("li").css( "border", "2px solid red" ); }); }); </script> <style> .great-grand-parent *{display:block; border:2px solid #aaa; color:#aaa; padding:5px; margin:5px;} </style> </head> <body> <div class="great-grand-parent"> <div class="grand-parent" style="width:500px;"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> <div class="grand-parent" style="width:500px;"> <ul class="parent"> <li class="child-three">Child Three</li> <li class="child-four">Child Four</li> </ul> </div> </div> <br> <button>Mark Children</button> </body> </html>
jQuery 遍历参考
您可以在以下页面获取遍历 DOM 的所有 jQuery 方法的完整参考:jQuery 遍历参考。