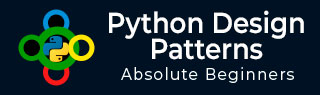
- Python 设计模式教程
- Python 设计模式 - 主页
- 介绍
- Python 设计模式 - 要点
- 模型视图控制器模式
- Python 设计模式 - 单例
- Python 设计模式 - 工厂
- Python 设计模式 - 构建器
- Python 设计模式 - 原型
- Python 设计模式 - 外观
- Python 设计模式 - 命令
- Python 设计模式 - 适配器
- Python 设计模式 - 装饰器
- Python 设计模式 - 代理
- 责任链模式
- Python 设计模式 - 观察者
- Python 设计模式 - 状态
- Python 设计模式 - 策略
- Python 设计模式 - 模板
- Python 设计模式 - 享元
- 抽象工厂
- 面向对象
- 面向对象的概念实现
- Python 设计模式 - 迭代器
- 词典
- 列表数据结构
- Python 设计模式 - 集
- Python 设计模式 - 队列
- 字符串和序列化
- Python 中的并发
- Python 设计模式 - 反
- 异常处理
- Python 设计模式资源
- 快速指南
- Python 设计模式 - 资源
- 讨论
责任链
责任链模式用于实现软件中的松耦合,其中来自客户端的指定请求通过其中包含的对象链传递。它有助于构建对象链。请求从一端进入并从一个对象移动到另一端。
此模式允许对象在不知道哪个对象将处理请求的情况下发送命令。
如何实施责任链模式?
现在我们将了解如何实施责任链模式。
class ReportFormat(object): PDF = 0 TEXT = 1 class Report(object): def __init__(self, format_): self.title = 'Monthly report' self.text = ['Things are going', 'really, really well.'] self.format_ = format_ class Handler(object): def __init__(self): self.nextHandler = None def handle(self, request): self.nextHandler.handle(request) class PDFHandler(Handler): def handle(self, request): if request.format_ == ReportFormat.PDF: self.output_report(request.title, request.text) else: super(PDFHandler, self).handle(request) def output_report(self, title, text): print '<html>' print ' <head>' print ' <title>%s</title>' % title print ' </head>' print ' <body>' for line in text: print ' <p>%s' % line print ' </body>' print '</html>' class TextHandler(Handler): def handle(self, request): if request.format_ == ReportFormat.TEXT: self.output_report(request.title, request.text) else: super(TextHandler, self).handle(request) def output_report(self, title, text): print 5*'*' + title + 5*'*' for line in text: print line class ErrorHandler(Handler): def handle(self, request): print "Invalid request" if __name__ == '__main__': report = Report(ReportFormat.TEXT) pdf_handler = PDFHandler() text_handler = TextHandler() pdf_handler.nextHandler = text_handler text_handler.nextHandler = ErrorHandler() pdf_handler.handle(report)
输出
上述程序生成以下输出 -

解释
上面的代码创建每月任务的报告,其中通过每个函数发送命令。它需要两个处理程序——用于 PDF 和文本。一旦所需的对象执行每个函数,它就会打印输出。