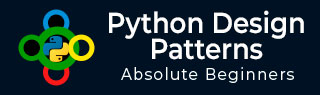
- Python 设计模式教程
- Python 设计模式 - 主页
- 介绍
- Python 设计模式 - 要点
- 模型视图控制器模式
- Python 设计模式 - 单例
- Python 设计模式 - 工厂
- Python 设计模式 - 构建器
- Python 设计模式 - 原型
- Python 设计模式 - 外观
- Python 设计模式 - 命令
- Python 设计模式 - 适配器
- Python 设计模式 - 装饰器
- Python 设计模式 - 代理
- 责任链模式
- Python 设计模式 - 观察者
- Python 设计模式 - 状态
- Python 设计模式 - 策略
- Python 设计模式 - 模板
- Python 设计模式 - 享元
- 抽象工厂
- 面向对象
- 面向对象的概念实现
- Python 设计模式 - 迭代器
- 词典
- 列表数据结构
- Python 设计模式 - 集
- Python 设计模式 - 队列
- 字符串和序列化
- Python 中的并发
- Python 设计模式 - 反
- 异常处理
- Python 设计模式资源
- 快速指南
- Python 设计模式 - 资源
- 讨论
Python 设计模式 - 享元
蝇量级模式属于结构设计模式类别。它提供了一种减少对象数量的方法。它包括有助于改进应用程序结构的各种功能。享元对象最重要的特征是不可变的。这意味着它们一旦构建就无法修改。该模式使用 HashMap 来存储引用对象。
如何实现享元模式?
以下程序有助于实现享元模式 -
class ComplexGenetics(object): def __init__(self): pass def genes(self, gene_code): return "ComplexPatter[%s]TooHugeinSize" % (gene_code) class Families(object): family = {} def __new__(cls, name, family_id): try: id = cls.family[family_id] except KeyError: id = object.__new__(cls) cls.family[family_id] = id return id def set_genetic_info(self, genetic_info): cg = ComplexGenetics() self.genetic_info = cg.genes(genetic_info) def get_genetic_info(self): return (self.genetic_info) def test(): data = (('a', 1, 'ATAG'), ('a', 2, 'AAGT'), ('b', 1, 'ATAG')) family_objects = [] for i in data: obj = Families(i[0], i[1]) obj.set_genetic_info(i[2]) family_objects.append(obj) for i in family_objects: print "id = " + str(id(i)) print i.get_genetic_info() print "similar id's says that they are same objects " if __name__ == '__main__': test()
输出
上述程序生成以下输出 -
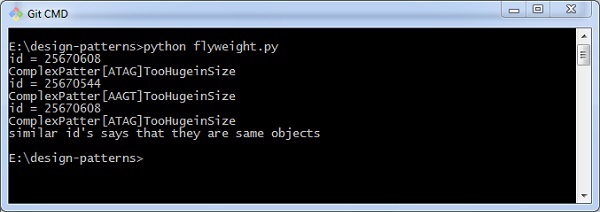