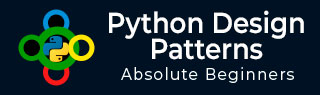
- Python 设计模式教程
- Python 设计模式 - 主页
- 介绍
- Python 设计模式 - 要点
- 模型视图控制器模式
- Python 设计模式 - 单例
- Python 设计模式 - 工厂
- Python 设计模式 - 构建器
- Python 设计模式 - 原型
- Python 设计模式 - 外观
- Python 设计模式 - 命令
- Python 设计模式 - 适配器
- Python 设计模式 - 装饰器
- Python 设计模式 - 代理
- 责任链模式
- Python 设计模式 - 观察者
- Python 设计模式 - 状态
- Python 设计模式 - 策略
- Python 设计模式 - 模板
- Python 设计模式 - 享元
- 抽象工厂
- 面向对象
- 面向对象的概念实现
- Python 设计模式 - 迭代器
- 词典
- 列表数据结构
- Python 设计模式 - 集
- Python 设计模式 - 队列
- 字符串和序列化
- Python 中的并发
- Python 设计模式 - 反
- 异常处理
- Python 设计模式资源
- 快速指南
- Python 设计模式 - 资源
- 讨论
Python 设计模式 - 装饰器
装饰器模式允许用户向现有对象添加新功能而不改变其结构。这种类型的设计模式属于结构模式,因为该模式充当现有类的包装器。
此模式创建一个装饰器类,它包装原始类并提供附加功能,保持类方法签名完整。
装饰器模式的动机是动态附加对象的附加职责。
如何实现装饰器设计模式
下面提到的代码是如何在Python中实现装饰器设计模式的简单演示。该插图涉及以课堂形式演示咖啡店。创建的咖啡类是一个抽象类,这意味着它不能被实例化。
import six from abc import ABCMeta @six.add_metaclass(ABCMeta) class Abstract_Coffee(object): def get_cost(self): pass def get_ingredients(self): pass def get_tax(self): return 0.1*self.get_cost() class Concrete_Coffee(Abstract_Coffee): def get_cost(self): return 1.00 def get_ingredients(self): return 'coffee' @six.add_metaclass(ABCMeta) class Abstract_Coffee_Decorator(Abstract_Coffee): def __init__(self,decorated_coffee): self.decorated_coffee = decorated_coffee def get_cost(self): return self.decorated_coffee.get_cost() def get_ingredients(self): return self.decorated_coffee.get_ingredients() class Sugar(Abstract_Coffee_Decorator): def __init__(self,decorated_coffee): Abstract_Coffee_Decorator.__init__(self,decorated_coffee) def get_cost(self): return self.decorated_coffee.get_cost() def get_ingredients(self): return self.decorated_coffee.get_ingredients() + ', sugar' class Milk(Abstract_Coffee_Decorator): def __init__(self,decorated_coffee): Abstract_Coffee_Decorator.__init__(self,decorated_coffee) def get_cost(self): return self.decorated_coffee.get_cost() + 0.25 def get_ingredients(self): return self.decorated_coffee.get_ingredients() + ', milk' class Vanilla(Abstract_Coffee_Decorator): def __init__(self,decorated_coffee): Abstract_Coffee_Decorator.__init__(self,decorated_coffee) def get_cost(self): return self.decorated_coffee.get_cost() + 0.75 def get_ingredients(self): return self.decorated_coffee.get_ingredients() + ', vanilla'
咖啡店抽象类的实现是通过一个单独的文件完成的,如下所述 -
import coffeeshop myCoffee = coffeeshop.Concrete_Coffee() print('Ingredients: '+myCoffee.get_ingredients()+ '; Cost: '+str(myCoffee.get_cost())+'; sales tax = '+str(myCoffee.get_tax())) myCoffee = coffeeshop.Milk(myCoffee) print('Ingredients: '+myCoffee.get_ingredients()+ '; Cost: '+str(myCoffee.get_cost())+'; sales tax = '+str(myCoffee.get_tax())) myCoffee = coffeeshop.Vanilla(myCoffee) print('Ingredients: '+myCoffee.get_ingredients()+ '; Cost: '+str(myCoffee.get_cost())+'; sales tax = '+str(myCoffee.get_tax())) myCoffee = coffeeshop.Sugar(myCoffee) print('Ingredients: '+myCoffee.get_ingredients()+ '; Cost: '+str(myCoffee.get_cost())+'; sales tax = '+str(myCoffee.get_tax()))
输出
上述程序生成以下输出 -
