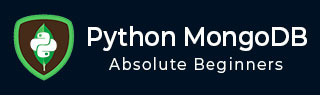
- Python MongoDB Tutorial
- Python MongoDB - Home
- Python MongoDB - Introduction
- Python MongoDB - Create Database
- Python MongoDB - Create Collection
- Python MongoDB - Insert Document
- Python MongoDB - Find
- Python MongoDB - Query
- Python MongoDB - Sort
- Python MongoDB - Delete Document
- Python MongoDB - Drop Collection
- Python MongoDB - Update
- Python MongoDB - Limit
- Python MongoDB Useful Resources
- Python MongoDB - Quick Guide
- Python MongoDB - Useful Resources
- Python MongoDB - Discussion
Python MongoDB - 删除集合
您可以使用MongoDB 的drop()方法删除集合。
句法
以下是 drop() 方法的语法 -
db.COLLECTION_NAME.drop()
例子
以下示例删除名称为 Sample 的集合 -
> show collections myColl sample > db.sample.drop() true > show collections myColl
使用 Python 删除集合
您可以通过调用 drop() 方法从当前集合中删除/删除集合。
例子
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['example2'] #Creating a collection col1 = db['collection'] col1.insert_one({"name": "Ram", "age": "26", "city": "Hyderabad"}) col2 = db['coll'] col2.insert_one({"name": "Rahim", "age": "27", "city": "Bangalore"}) col3 = db['myColl'] col3.insert_one({"name": "Robert", "age": "28", "city": "Mumbai"}) col4 = db['data'] col4.insert_one({"name": "Romeo", "age": "25", "city": "Pune"}) #List of collections print("List of collections:") collections = db.list_collection_names() for coll in collections: print(coll) #Dropping a collection col1.drop() col4.drop() print("List of collections after dropping two of them: ") #List of collections collections = db.list_collection_names() for coll in collections: print(coll)
输出
List of collections: coll data collection myColl List of collections after dropping two of them: coll myColl