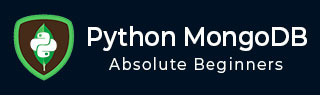
- Python MongoDB Tutorial
- Python MongoDB - Home
- Python MongoDB - Introduction
- Python MongoDB - Create Database
- Python MongoDB - Create Collection
- Python MongoDB - Insert Document
- Python MongoDB - Find
- Python MongoDB - Query
- Python MongoDB - Sort
- Python MongoDB - Delete Document
- Python MongoDB - Drop Collection
- Python MongoDB - Update
- Python MongoDB - Limit
- Python MongoDB Useful Resources
- Python MongoDB - Quick Guide
- Python MongoDB - Useful Resources
- Python MongoDB - Discussion
Python MongoDB - 快速指南
Python MongoDB - 简介
Pymongo 是一个 Python 发行版,它提供了使用 MongoDB 的工具,它是从 Python 与 MongoDB 数据库通信的首选方式。
安装
要安装 pymongo,首先请确保您已正确安装 python3(以及 PIP)和 MongoDB。然后执行以下命令。
C:\WINDOWS\system32>pip install pymongo Collecting pymongo Using cached https://files.pythonhosted.org/packages/cb/a6/b0ae3781b0ad75825e00e29dc5489b53512625e02328d73556e1ecdf12f8/pymongo-3.9.0-cp37-cp37m-win32.whl Installing collected packages: pymongo Successfully installed pymongo-3.9.0
确认
安装 pymongo 后,打开一个新的文本文档,将以下行粘贴到其中,然后将其另存为 test.py。
import pymongo
如果你已经正确安装了 pymongo,如果你执行如下所示的 test.py,你应该不会遇到任何问题。
D:\Python_MongoDB>test.py D:\Python_MongoDB>
Python MongoDB - 创建数据库
与其他数据库不同,MongoDB 不提供单独的命令来创建数据库。
一般来说,use命令用于选择/切换到特定的数据库。该命令首先验证我们指定的数据库是否存在,如果存在,则连接到该数据库。如果我们使用 use 命令指定的数据库不存在,则会创建一个新数据库。
因此,您可以使用Use命令在 MongoDB 中创建数据库。
句法
use DATABASE语句的基本语法如下 -
use DATABASE_NAME
例子
以下命令创建一个名为 mydb 的数据库。
>use mydb switched to db mydb
您可以使用db命令验证您的创建,这会显示当前数据库。
>db mydb
使用 Python 创建数据库
使用pymongo连接MongoDB,需要导入并创建一个MongoClient,然后就可以在属性passion中直接访问需要创建的数据库了。
例子
以下示例在 MangoDB 中创建一个数据库。
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['mydb'] print("Database created........") #Verification print("List of databases after creating new one") print(client.list_database_names())
输出
Database created........ List of databases after creating new one: ['admin', 'config', 'local', 'mydb']
您还可以在创建 MongoClient 时指定端口和主机名,并可以以字典方式访问数据库。
例子
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['mydb'] print("Database created........")
输出
Database created........
Python MongoDB - 创建集合
MongoDB 中的集合保存一组文档,类似于关系数据库中的表。
您可以使用createCollection()方法创建集合。此方法接受一个表示要创建的集合名称的字符串值和一个选项(可选)参数。
使用它您可以指定以下内容 -
集合的大小。
上限集合中允许的最大文档数。
我们创建的集合是否应该是上限集合(固定大小集合)。
我们创建的集合是否应该自动索引。
句法
以下是在 MongoDB 中创建集合的语法。
db.createCollection("CollectionName")
例子
以下方法创建一个名为ExampleCollection 的集合。
> use mydb switched to db mydb > db.createCollection("ExampleCollection") { "ok" : 1 } >
同样,以下是使用 createCollection() 方法的选项创建集合的查询。
>db.createCollection("mycol", { capped : true, autoIndexId : true, size : 6142800, max : 10000 } ) { "ok" : 1 } >
使用 Python 创建集合
以下 python 示例连接到 MongoDB (mydb) 中的数据库,并在其中创建一个集合。
例子
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['mydb'] #Creating a collection collection = db['example'] print("Collection created........")
输出
Collection created........
Python MongoDB - 插入文档
您可以使用insert()方法将文档存储到 MongoDB 中。此方法接受 JSON 文档作为参数。
句法
以下是插入方法的语法。
>db.COLLECTION_NAME.insert(DOCUMENT_NAME)
例子
> use mydb switched to db mydb > db.createCollection("sample") { "ok" : 1 } > doc1 = {"name": "Ram", "age": "26", "city": "Hyderabad"} { "name" : "Ram", "age" : "26", "city" : "Hyderabad" } > db.sample.insert(doc1) WriteResult({ "nInserted" : 1 }) >
同样,您也可以使用insert()方法插入多个文档。
> use testDB switched to db testDB > db.createCollection("sample") { "ok" : 1 } > data = [ {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" }, {"_id": "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" } ] [ {"_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad"}, {"_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore"}, {"_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai"} ] > db.sample.insert(data) BulkWriteResult({ "writeErrors" : [ ], "writeConcernErrors" : [ ], "nInserted" : 3, "nUpserted" : 0, "nMatched" : 0, "nModified" : 0, "nRemoved" : 0, "upserted" : [ ] }) >
使用 Python 创建集合
Pymongo 提供了一个名为 insert_one() 的方法来在 MangoDB 中插入文档。对于这个方法,我们需要以字典格式传递文档。
例子
以下示例在名为 example 的集合中插入一个文档。
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['mydb'] #Creating a collection coll = db['example'] #Inserting document into a collection doc1 = {"name": "Ram", "age": "26", "city": "Hyderabad"} coll.insert_one(doc1) print(coll.find_one())
输出
{'_id': ObjectId('5d63ad6ce043e2a93885858b'), 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'}
要使用 pymongo 将多个文档插入 MongoDB,您需要调用 insert_many() 方法。
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['mydb'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ {"_id": "101", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "102", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "103", "name": "Robert", "age": "28", "city": "Mumbai"} ] res = coll.insert_many(data) print("Data inserted ......") print(res.inserted_ids)
输出
Data inserted ...... ['101', '102', '103']
Python MongoDB - 查找
您可以使用find()方法从 MongoDB 读取/检索存储的文档。该方法以非结构化方式检索并显示 MongoDB 中的所有文档。
句法
以下是find()方法的语法。
>db.COLLECTION_NAME.find()
例子
假设我们使用以下查询将 3 个文档插入到名为 example 的集合中名为 testDB 的数据库中 -
> use testDB > db.createCollection("sample") > data = [ {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" }, {"_id": "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" } ] > db.sample.insert(data)
您可以使用 find() 方法检索插入的文档:
> use testDB switched to db testDB > db.sample.find() { "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad" } { "_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" } { "_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" } >
您还可以使用 findOne() 方法检索集合中的第一个文档:
> db.sample.findOne() { "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad" }
使用 Python 检索数据(查找)
pymongo 的find_One ()方法用于根据您的查询检索单个文档,如果没有匹配,此方法不会返回任何内容,如果您不使用任何查询,它会返回集合的第一个文档。
每当您需要仅检索结果的一个文档或者您确定查询仅返回一个文档时,此方法就会派上用场。
例子
以下 python 示例检索集合的第一个文档 -
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['mydatabase'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ {"_id": "101", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "102", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "103", "name": "Robert", "age": "28", "city": "Mumbai"} ] res = coll.insert_many(data) print("Data inserted ......") print(res.inserted_ids) #Retrieving the first record using the find_one() method print("First record of the collection: ") print(coll.find_one()) #Retrieving a record with is 103 using the find_one() method print("Record whose id is 103: ") print(coll.find_one({"_id": "103"}))
输出
Data inserted ...... ['101', '102', '103'] First record of the collection: {'_id': '101', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} Record whose id is 103: {'_id': '103', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'}
要在单个查询中获取多个文档(单个调用 od find 方法),可以使用pymongo 的find()方法。如果未传递任何查询,则这将返回集合的所有文档,如果您已将查询传递给此方法,则它将返回所有匹配的文档。
例子
#Getting the database instance db = client['myDB'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ {"_id": "101", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "102", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "103", "name": "Robert", "age": "28", "city": "Mumbai"} ] res = coll.insert_many(data) print("Data inserted ......") #Retrieving all the records using the find() method print("Records of the collection: ") for doc1 in coll.find(): print(doc1) #Retrieving records with age greater than 26 using the find() method print("Record whose age is more than 26: ") for doc2 in coll.find({"age":{"$gt":"26"}}): print(doc2)
输出
Data inserted ...... Records of the collection: {'_id': '101', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} {'_id': '102', 'name': 'Rahim', 'age': '27', 'city': 'Bangalore'} {'_id': '103', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'} Record whose age is more than 26: {'_id': '102', 'name': 'Rahim', 'age': '27', 'city': 'Bangalore'} {'_id': '103', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'}
Python MongoDB - 查询
使用find()方法检索时,您可以使用查询对象过滤文档。您可以将指定所需文档条件的查询作为参数传递给此方法。
运营商
以下是 MongoDB 查询中使用的运算符列表。
手术 | 句法 | 例子 |
---|---|---|
平等 | {“核心价值”} | db.mycol.find({"by":"教程点"}) |
少于 | {“键”:{$lt:“值”}} | db.mycol.find({"喜欢":{$lt:50}}) |
小于等于 | {“键”:{$lte:“值”}} | db.mycol.find({"喜欢":{$lte:50}}) |
比...更棒 | {“键”:{$gt:“值”}} | db.mycol.find({"喜欢":{$gt:50}}) |
大于等于 | {“键”{$gte:“值”}} | db.mycol.find({"喜欢":{$gte:50}}) |
不等于 | {“键”:{$ne:“值”}} | db.mycol.find({"喜欢":{$ne:50}}) |
示例1
以下示例检索名称为 sarmista 的集合中的文档。
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['sdsegf'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "1002", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "1003", "name": "Robert", "age": "28", "city": "Mumbai"}, {"_id": "1004", "name": "Romeo", "age": "25", "city": "Pune"}, {"_id": "1005", "name": "Sarmista", "age": "23", "city": "Delhi"}, {"_id": "1006", "name": "Rasajna", "age": "26", "city": "Chennai"} ] res = coll.insert_many(data) print("Data inserted ......") #Retrieving data print("Documents in the collection: ") for doc1 in coll.find({"name":"Sarmista"}): print(doc1)
输出
Data inserted ...... Documents in the collection: {'_id': '1005', 'name': 'Sarmista', 'age': '23', 'city': 'Delhi'}
例2
以下示例检索集合中年龄值大于 26 的文档。
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['ghhj'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "1002", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "1003", "name": "Robert", "age": "28", "city": "Mumbai"}, {"_id": "1004", "name": "Romeo", "age": "25", "city": "Pune"}, {"_id": "1005", "name": "Sarmista", "age": "23", "city": "Delhi"}, {"_id": "1006", "name": "Rasajna", "age": "26", "city": "Chennai"} ] res = coll.insert_many(data) print("Data inserted ......") #Retrieving data print("Documents in the collection: ") for doc in coll.find({"age":{"$gt":"26"}}): print(doc)
输出
Data inserted ...... Documents in the collection: {'_id': '1002', 'name': 'Rahim', 'age': '27', 'city': 'Bangalore'} {'_id': '1003', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'}
Python MongoDB - 排序
在检索集合的内容时,您可以使用sort()方法对它们进行升序或降序排序和排列。
对于此方法,您可以传递字段和排序顺序(1 或 -1)。其中,1为升序,-1为降序。
句法
以下是sort()方法的语法。
>db.COLLECTION_NAME.find().sort({KEY:1})
例子
假设我们创建了一个集合并向其中插入了 5 个文档,如下所示 -
> use testDB switched to db testDB > db.createCollection("myColl") { "ok" : 1 } > data = [ ... {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, ... {"_id": "1002", "name": "Rahim", "age": 27, "city": "Bangalore"}, ... {"_id": "1003", "name": "Robert", "age": 28, "city": "Mumbai"}, ... {"_id": "1004", "name": "Romeo", "age": 25, "city": "Pune"}, ... {"_id": "1005", "name": "Sarmista", "age": 23, "city": "Delhi"}, ... {"_id": "1006", "name": "Rasajna", "age": 26, "city": "Chennai"} ] > db.sample.insert(data) BulkWriteResult({ "writeErrors" : [ ], "writeConcernErrors" : [ ], "nInserted" : 6, "nUpserted" : 0, "nMatched" : 0, "nModified" : 0, "nRemoved" : 0, "upserted" : [ ] })
以下行检索集合中的所有文档,这些文档根据年龄按升序排序。
> db.sample.find().sort({age:1}) { "_id" : "1005", "name" : "Sarmista", "age" : 23, "city" : "Delhi" } { "_id" : "1004", "name" : "Romeo", "age" : 25, "city" : "Pune" } { "_id" : "1006", "name" : "Rasajna", "age" : 26, "city" : "Chennai" } { "_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" } { "_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" } { "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad" }
使用 Python 对文档进行排序
要按升序或降序对查询结果进行排序,pymongo 提供了sort()方法。向此方法传递一个数值,表示结果中所需的文档数量。
默认情况下,此方法根据指定字段按升序对文档进行排序。如果您需要按降序排序,请传递 -1 和字段名称 -
coll.find().sort("age",-1)
例子
以下示例检索根据年龄值升序排列的集合的所有文档 -
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['b_mydb'] #Creating a collection coll = db['myColl'] #Inserting document into a collection data = [ {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "1002", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "1003", "name": "Robert", "age": "28", "city": "Mumbai"}, {"_id": "1004", "name": "Romeo", "age": 25, "city": "Pune"}, {"_id": "1005", "name": "Sarmista", "age": 23, "city": "Delhi"}, {"_id": "1006", "name": "Rasajna", "age": 26, "city": "Chennai"} ] res = coll.insert_many(data) print("Data inserted ......") #Retrieving first 3 documents using the find() and limit() methods print("List of documents (sorted in ascending order based on age): ") for doc1 in coll.find().sort("age"): print(doc1)
输出
Data inserted ...... List of documents (sorted in ascending order based on age): {'_id': '1005', 'name': 'Sarmista', 'age': 23, 'city': 'Delhi'} {'_id': '1004', 'name': 'Romeo', 'age': 25, 'city': 'Pune'} {'_id': '1006', 'name': 'Rasajna', 'age': 26, 'city': 'Chennai'} {'_id': '1001', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} {'_id': '1002', 'name': 'Rahim', 'age': '27', 'city': 'Bangalore'} {'_id': '1003', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'}
Python MongoDB - 删除文档
您可以使用MongoDB的remove()方法删除集合中的文档。此方法接受两个可选参数 -
删除条件指定删除文档的条件。
只有一个,如果你传递 true 或 1 作为第二个参数,那么只会删除一个文档。
句法
以下是 remove() 方法的语法 -
>db.COLLECTION_NAME.remove(DELLETION_CRITTERIA)
例子
假设我们创建了一个集合并向其中插入了 5 个文档,如下所示 -
> use testDB switched to db testDB > db.createCollection("myColl") { "ok" : 1 } > data = [ ... {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, ... {"_id": "1002", "name": "Rahim", "age": 27, "city": "Bangalore"}, ... {"_id": "1003", "name": "Robert", "age": 28, "city": "Mumbai"}, ... {"_id": "1004", "name": "Romeo", "age": 25, "city": "Pune"}, ... {"_id": "1005", "name": "Sarmista", "age": 23, "city": "Delhi"}, ... {"_id": "1006", "name": "Rasajna", "age": 26, "city": "Chennai"} ] > db.sample.insert(data) BulkWriteResult({ "writeErrors" : [ ], "writeConcernErrors" : [ ], "nInserted" : 6, "nUpserted" : 0, "nMatched" : 0, "nModified" : 0, "nRemoved" : 0, "upserted" : [ ] })
以下查询删除集合中名称值为 Sarmista 的文档。
> db.sample.remove({"name": "Sarmista"}) WriteResult({ "nRemoved" : 1 }) > db.sample.find() { "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad" } { "_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" } { "_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" } { "_id" : "1004", "name" : "Romeo", "age" : 25, "city" : "Pune" } { "_id" : "1006", "name" : "Rasajna", "age" : 26, "city" : "Chennai" }
如果您调用remove()方法而不传递删除条件,则集合中的所有文档都将被删除。
> db.sample.remove({}) WriteResult({ "nRemoved" : 5 }) > db.sample.find()
使用Python删除文档
要从MangoDB集合中删除文档,可以使用delete_one()和delete_many()方法从集合中删除文档。
这些方法接受指定删除文档条件的查询对象。
如果匹配,detele_one() 方法会删除单个文档。如果未指定查询,此方法将删除集合中的第一个文档。
例子
以下 python 示例删除集合中 id 值为 1006 的文档。
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['lpaksgf'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "1002", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "1003", "name": "Robert", "age": "28", "city": "Mumbai"}, {"_id": "1004", "name": "Romeo", "age": 25, "city": "Pune"}, {"_id": "1005", "name": "Sarmista", "age": 23, "city": "Delhi"}, {"_id": "1006", "name": "Rasajna", "age": 26, "city": "Chennai"} ] res = coll.insert_many(data) print("Data inserted ......") #Deleting one document coll.delete_one({"_id" : "1006"}) #Retrieving all the records using the find() method print("Documents in the collection after update operation: ") for doc2 in coll.find(): print(doc2)
输出
Data inserted ...... Documents in the collection after update operation: {'_id': '1001', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} {'_id': '1002', 'name': 'Rahim', 'age': '27', 'city': 'Bangalore'} {'_id': '1003', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'} {'_id': '1004', 'name': 'Romeo', 'age': 25, 'city': 'Pune'} {'_id': '1005', 'name': 'Sarmista', 'age': 23, 'city': 'Delhi'}
同样, pymongo的delete_many()方法删除所有满足指定条件的文档。
例子
以下示例删除集合中年龄值大于 26 的所有文档 -
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['sampleDB'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "1002", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "1003", "name": "Robert", "age": "28", "city": "Mumbai"}, {"_id": "1004", "name": "Romeo", "age": "25", "city": "Pune"}, {"_id": "1005", "name": "Sarmista", "age": "23", "city": "Delhi"}, {"_id": "1006", "name": "Rasajna", "age": "26", "city": "Chennai"} ] res = coll.insert_many(data) print("Data inserted ......") #Deleting multiple documents coll.delete_many({"age":{"$gt":"26"}}) #Retrieving all the records using the find() method print("Documents in the collection after update operation: ") for doc2 in coll.find(): print(doc2)
输出
Data inserted ...... Documents in the collection after update operation: {'_id': '1001', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} {'_id': '1004', 'name': 'Romeo', 'age': '25', 'city': 'Pune'} {'_id': '1005', 'name': 'Sarmista', 'age': '23', 'city': 'Delhi'} {'_id': '1006', 'name': 'Rasajna', 'age': '26', 'city': 'Chennai'}
如果您调用delete_many() 方法而不传递任何查询,则此方法将删除集合中的所有文档。
coll.delete_many({})
Python MongoDB - 删除集合
您可以使用MongoDB 的drop()方法删除集合。
句法
以下是 drop() 方法的语法 -
db.COLLECTION_NAME.drop()
例子
以下示例删除名称为 Sample 的集合 -
> show collections myColl sample > db.sample.drop() true > show collections myColl
使用 Python 删除集合
您可以通过调用 drop() 方法从当前集合中删除/删除集合。
例子
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['example2'] #Creating a collection col1 = db['collection'] col1.insert_one({"name": "Ram", "age": "26", "city": "Hyderabad"}) col2 = db['coll'] col2.insert_one({"name": "Rahim", "age": "27", "city": "Bangalore"}) col3 = db['myColl'] col3.insert_one({"name": "Robert", "age": "28", "city": "Mumbai"}) col4 = db['data'] col4.insert_one({"name": "Romeo", "age": "25", "city": "Pune"}) #List of collections print("List of collections:") collections = db.list_collection_names() for coll in collections: print(coll) #Dropping a collection col1.drop() col4.drop() print("List of collections after dropping two of them: ") #List of collections collections = db.list_collection_names() for coll in collections: print(coll)
输出
List of collections: coll data collection myColl List of collections after dropping two of them: coll myColl
Python MongoDB - 更新
您可以使用update()方法或save()方法更新现有文档的内容。
update 方法修改现有文档,而 save 方法用新文档替换现有文档。
句法
以下是 MangoDB 的 update() 和 save() 方法的语法 -
>db.COLLECTION_NAME.update(SELECTION_CRITERIA, UPDATED_DATA) Or, db.COLLECTION_NAME.save({_id:ObjectId(),NEW_DATA})
例子
假设我们在数据库中创建了一个集合并在其中插入了 3 条记录,如下所示 -
> use testdatabase switched to db testdatabase > data = [ ... {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, ... {"_id": "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" }, ... {"_id": "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" } ] [ {"_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad"}, {"_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore"}, {"_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai"} ] > db.createCollection("sample") { "ok" : 1 } > db.sample.insert(data)
以下方法更新 id 为 1002 的文档的城市值。
>db.sample.update({"_id":"1002"},{"$set":{"city":"Visakhapatnam"}}) WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 }) > db.sample.find() { "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad" } { "_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Visakhapatnam" } { "_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" }
同样,您可以使用 save() 方法以相同的 id 保存文档,从而用新数据替换文档。
> db.sample.save({ "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Vijayawada" }) WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 }) > db.sample.find() { "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Vijayawada" } { "_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Visakhapatnam" } { "_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" }
使用 python 更新文档
与检索单个文档的 find_one() 方法类似,pymongo 的 update_one() 方法更新单个文档。
此方法接受指定要更新哪个文档以及更新操作的查询。
例子
以下 python 示例更新集合中文档的位置值。
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['myDB'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ {"_id": "101", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "102", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "103", "name": "Robert", "age": "28", "city": "Mumbai"} ] res = coll.insert_many(data) print("Data inserted ......") #Retrieving all the records using the find() method print("Documents in the collection: ") for doc1 in coll.find(): print(doc1) coll.update_one({"_id":"102"},{"$set":{"city":"Visakhapatnam"}}) #Retrieving all the records using the find() method print("Documents in the collection after update operation: ") for doc2 in coll.find(): print(doc2)
输出
Data inserted ...... Documents in the collection: {'_id': '101', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} {'_id': '102', 'name': 'Rahim', 'age': '27', 'city': 'Bangalore'} {'_id': '103', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'} Documents in the collection after update operation: {'_id': '101', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} {'_id': '102', 'name': 'Rahim', 'age': '27', 'city': 'Visakhapatnam'} {'_id': '103', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'}
同样, pymongo的update_many()方法会更新所有满足指定条件的文档。
例子
以下示例更新集合中所有文档中的位置值(空条件) -
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['myDB'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ {"_id": "101", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "102", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "103", "name": "Robert", "age": "28", "city": "Mumbai"} ] res = coll.insert_many(data) print("Data inserted ......") #Retrieving all the records using the find() method print("Documents in the collection: ") for doc1 in coll.find(): print(doc1) coll.update_many({},{"$set":{"city":"Visakhapatnam"}}) #Retrieving all the records using the find() method print("Documents in the collection after update operation: ") for doc2 in coll.find(): print(doc2)
输出
Data inserted ...... Documents in the collection: {'_id': '101', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} {'_id': '102', 'name': 'Rahim', 'age': '27', 'city': 'Bangalore'} {'_id': '103', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'} Documents in the collection after update operation: {'_id': '101', 'name': 'Ram', 'age': '26', 'city': 'Visakhapatnam'} {'_id': '102', 'name': 'Rahim', 'age': '27', 'city': 'Visakhapatnam'} {'_id': '103', 'name': 'Robert', 'age': '28', 'city': 'Visakhapatnam'}
Python MongoDB - 限制
在检索集合的内容时,您可以使用 limit() 方法限制结果中的文档数量。此方法接受一个数值,表示您希望在结果中包含的文档数量。
句法
以下是 limit() 方法的语法 -
>db.COLLECTION_NAME.find().limit(NUMBER)
例子
假设我们创建了一个集合并向其中插入了 5 个文档,如下所示 -
> use testDB switched to db testDB > db.createCollection("sample") { "ok" : 1 } > data = [ ... {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, ... {"_id": "1002", "name": "Rahim", "age": 27, "city": "Bangalore"}, ... {"_id": "1003", "name": "Robert", "age": 28, "city": "Mumbai"}, ... {"_id": "1004", "name": "Romeo", "age": 25, "city": "Pune"}, ... {"_id": "1005", "name": "Sarmista", "age": 23, "city": "Delhi"}, ... {"_id": "1006", "name": "Rasajna", "age": 26, "city": "Chennai"} ] > db.sample.insert(data) BulkWriteResult({ "writeErrors" : [ ], "writeConcernErrors" : [ ], "nInserted" : 6, "nUpserted" : 0, "nMatched" : 0, "nModified" : 0, "nRemoved" : 0, "upserted" : [ ] })
以下行检索集合的前 3 个文档。
> db.sample.find().limit(3) { "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad" } { "_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" } { "_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" }
使用 Python 限制文档
为了将查询结果限制为特定数量的文档,pymongo 提供了limit()方法。向此方法传递一个数值,表示结果中所需的文档数量。
例子
以下示例检索集合中的前三个文档。
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['l'] #Creating a collection coll = db['myColl'] #Inserting document into a collection data = [ {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "1002", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "1003", "name": "Robert", "age": "28", "city": "Mumbai"}, {"_id": "1004", "name": "Romeo", "age": 25, "city": "Pune"}, {"_id": "1005", "name": "Sarmista", "age": 23, "city": "Delhi"}, {"_id": "1006", "name": "Rasajna", "age": 26, "city": "Chennai"} ] res = coll.insert_many(data) print("Data inserted ......") #Retrieving first 3 documents using the find() and limit() methods print("First 3 documents in the collection: ") for doc1 in coll.find().limit(3): print(doc1)
输出
Data inserted ...... First 3 documents in the collection: {'_id': '1001', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} {'_id': '1002', 'name': 'Rahim', 'age': '27', 'city': 'Bangalore'} {'_id': '1003', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'}