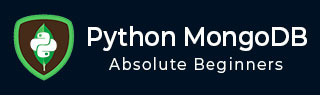
- Python MongoDB Tutorial
- Python MongoDB - Home
- Python MongoDB - Introduction
- Python MongoDB - Create Database
- Python MongoDB - Create Collection
- Python MongoDB - Insert Document
- Python MongoDB - Find
- Python MongoDB - Query
- Python MongoDB - Sort
- Python MongoDB - Delete Document
- Python MongoDB - Drop Collection
- Python MongoDB - Update
- Python MongoDB - Limit
- Python MongoDB Useful Resources
- Python MongoDB - Quick Guide
- Python MongoDB - Useful Resources
- Python MongoDB - Discussion
Python MongoDB - 限制
在检索集合的内容时,您可以使用 limit() 方法限制结果中的文档数量。此方法接受一个数值,表示您希望在结果中包含的文档数量。
句法
以下是 limit() 方法的语法 -
>db.COLLECTION_NAME.find().limit(NUMBER)
例子
假设我们创建了一个集合并向其中插入了 5 个文档,如下所示 -
> use testDB switched to db testDB > db.createCollection("sample") { "ok" : 1 } > data = [ ... {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, ... {"_id": "1002", "name": "Rahim", "age": 27, "city": "Bangalore"}, ... {"_id": "1003", "name": "Robert", "age": 28, "city": "Mumbai"}, ... {"_id": "1004", "name": "Romeo", "age": 25, "city": "Pune"}, ... {"_id": "1005", "name": "Sarmista", "age": 23, "city": "Delhi"}, ... {"_id": "1006", "name": "Rasajna", "age": 26, "city": "Chennai"} ] > db.sample.insert(data) BulkWriteResult({ "writeErrors" : [ ], "writeConcernErrors" : [ ], "nInserted" : 6, "nUpserted" : 0, "nMatched" : 0, "nModified" : 0, "nRemoved" : 0, "upserted" : [ ] })
以下行检索集合的前 3 个文档。
> db.sample.find().limit(3) { "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad" } { "_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" } { "_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" }
使用 Python 限制文档
为了将查询结果限制为特定数量的文档,pymongo 提供了limit()方法。向此方法传递一个数值,表示结果中所需的文档数量。
例子
以下示例检索集合中的前三个文档。
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['l'] #Creating a collection coll = db['myColl'] #Inserting document into a collection data = [ {"_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad"}, {"_id": "1002", "name": "Rahim", "age": "27", "city": "Bangalore"}, {"_id": "1003", "name": "Robert", "age": "28", "city": "Mumbai"}, {"_id": "1004", "name": "Romeo", "age": 25, "city": "Pune"}, {"_id": "1005", "name": "Sarmista", "age": 23, "city": "Delhi"}, {"_id": "1006", "name": "Rasajna", "age": 26, "city": "Chennai"} ] res = coll.insert_many(data) print("Data inserted ......") #Retrieving first 3 documents using the find() and limit() methods print("First 3 documents in the collection: ") for doc1 in coll.find().limit(3): print(doc1)
输出
Data inserted ...... First 3 documents in the collection: {'_id': '1001', 'name': 'Ram', 'age': '26', 'city': 'Hyderabad'} {'_id': '1002', 'name': 'Rahim', 'age': '27', 'city': 'Bangalore'} {'_id': '1003', 'name': 'Robert', 'age': '28', 'city': 'Mumbai'}