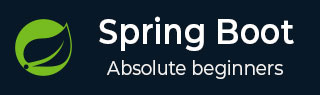
- Spring Boot 教程
- Spring Boot - 主页
- Spring Boot - 简介
- Spring Boot - 快速入门
- Spring Boot - 引导
- Spring Boot - Tomcat 部署
- Spring Boot - 构建系统
- Spring Boot - 代码结构
- Spring Bean 和依赖注入
- Spring Boot - 跑步者
- Spring Boot - 应用程序属性
- Spring Boot - 日志记录
- 构建 RESTful Web 服务
- Spring Boot - 异常处理
- Spring Boot - 拦截器
- Spring Boot - Servlet 过滤器
- Spring Boot - Tomcat 端口号
- Spring Boot - Rest 模板
- Spring Boot - 文件处理
- Spring Boot - 服务组件
- Spring Boot - Thymeleaf
- 使用 RESTful Web 服务
- Spring Boot - CORS 支持
- Spring Boot - 国际化
- Spring Boot - 调度
- Spring Boot - 启用 HTTPS
- Spring Boot-Eureka 服务器
- 向 Eureka 注册服务
- Zuul代理服务器和路由
- Spring Cloud配置服务器
- Spring Cloud 配置客户端
- Spring Boot - 执行器
- Spring Boot - 管理服务器
- Spring Boot - 管理客户端
- Spring Boot - 启用 Swagger2
- Spring Boot - 创建 Docker 镜像
- 追踪微服务日志
- Spring Boot - Flyway 数据库
- Spring Boot - 发送电子邮件
- Spring Boot-Hystrix
- Spring Boot - Web Socket
- Spring Boot - 批量服务
- Spring Boot-Apache Kafka
- Spring Boot - Twilio
- Spring Boot - 单元测试用例
- 休息控制器单元测试
- Spring Boot - 数据库处理
- 保护 Web 应用程序的安全
- Spring Boot - 使用 JWT 的 OAuth2
- Spring Boot - Google 云平台
- Spring Boot - Google OAuth2 登录
- Spring Boot 资源
- Spring Boot - 快速指南
- Spring Boot - 有用的资源
- Spring Boot - 讨论
Spring Boot-Apache Kafka
Apache Kafka 是一个开源项目,用于发布和订阅基于容错的消息系统。它具有快速、可扩展和分布式的设计。如果您是 Kafka 的初学者,或者想更好地了解它,请参阅此链接 - www.tutorialspoint.com/apache_kafka/
在本章中,我们将了解如何在 Spring Boot 应用程序中实现 Apache Kafka。
首先,我们需要在构建配置文件中添加 Spring Kafka 依赖项。
Maven 用户可以在 pom.xml 文件中添加以下依赖项。
<dependency> <groupId>org.springframework.kafka</groupId> <artifactId>spring-kafka</artifactId> <version>2.1.0.RELEASE</version> </dependency>
Gradle 用户可以在 build.gradle 文件中添加以下依赖项。
compile group: 'org.springframework.kafka', name: 'spring-kafka', version: '2.1.0.RELEASE'
生成消息
为了将消息生成到 Apache Kafka,我们需要为 Producer 配置定义 Configuration 类,如下所示 -
package com.tutorialspoint.kafkademo; import java.util.HashMap; import java.util.Map; import org.apache.kafka.clients.producer.ProducerConfig; import org.apache.kafka.common.serialization.StringSerializer; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.kafka.core.DefaultKafkaProducerFactory; import org.springframework.kafka.core.KafkaTemplate; import org.springframework.kafka.core.ProducerFactory; @Configuration public class KafkaProducerConfig { @Bean public ProducerFactory<String, String> producerFactory() { Map<String, Object> configProps = new HashMap<>(); configProps.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092"); configProps.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, StringSerializer.class); configProps.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class); return new DefaultKafkaProducerFactory<>(configProps); } @Bean public KafkaTemplate<String, String> kafkaTemplate() { return new KafkaTemplate<>(producerFactory()); } }
要发布消息,请自动连接 Kafka 模板对象并生成消息,如图所示。
@Autowired private KafkaTemplate<String, String> kafkaTemplate; public void sendMessage(String msg) { kafkaTemplate.send(topicName, msg); }
消费消息
为了消费消息,我们需要编写一个Consumer配置类文件,如下所示。
package com.tutorialspoint.kafkademo; import java.util.HashMap; import java.util.Map; import org.apache.kafka.clients.consumer.ConsumerConfig; import org.apache.kafka.common.serialization.StringDeserializer; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.kafka.annotation.EnableKafka; import org.springframework.kafka.config.ConcurrentKafkaListenerContainerFactory; import org.springframework.kafka.core.ConsumerFactory; import org.springframework.kafka.core.DefaultKafkaConsumerFactory; @EnableKafka @Configuration public class KafkaConsumerConfig { @Bean public ConsumerFactory<String, String> consumerFactory() { Map<String, Object> props = new HashMap<>(); props.put(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:2181"); props.put(ConsumerConfig.GROUP_ID_CONFIG, "group-id"); props.put(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class); props.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class); return new DefaultKafkaConsumerFactory<>(props); } @Bean public ConcurrentKafkaListenerContainerFactory<String, String> kafkaListenerContainerFactory() { ConcurrentKafkaListenerContainerFactory<String, String> factory = new ConcurrentKafkaListenerContainerFactory<>(); factory.setConsumerFactory(consumerFactory()); return factory; } }
接下来,编写一个监听器来监听消息。
@KafkaListener(topics = "tutorialspoint", groupId = "group-id") public void listen(String message) { System.out.println("Received Messasge in group - group-id: " + message); }
让我们从主 Spring Boot 应用程序类文件的 ApplicationRunner 类 run 方法中调用 sendMessage() 方法,并使用同一类文件中的消息。
您的主要 Spring Boot 应用程序类文件代码如下 -
package com.tutorialspoint.kafkademo; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.ApplicationArguments; import org.springframework.boot.ApplicationRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.kafka.annotation.KafkaListener; import org.springframework.kafka.core.KafkaTemplate; @SpringBootApplication public class KafkaDemoApplication implements ApplicationRunner { @Autowired private KafkaTemplate<String, String> kafkaTemplate; public void sendMessage(String msg) { kafkaTemplate.send("tutorialspoint", msg); } public static void main(String[] args) { SpringApplication.run(KafkaDemoApplication.class, args); } @KafkaListener(topics = "tutorialspoint", groupId = "group-id") public void listen(String message) { System.out.println("Received Messasge in group - group-id: " + message); } @Override public void run(ApplicationArguments args) throws Exception { sendMessage("Hi Welcome to Spring For Apache Kafka"); } }
下面给出了完整构建配置文件的代码。
Maven – pom.xml
<?xml version = "1.0" encoding = "UTF-8"?> <project xmlns = "http://maven.apache.org/POM/4.0.0" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>kafka-demo</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>kafka-demo</name> <description>Demo project for Spring Boot</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.9.RELEASE</version> <relativePath /> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.kafka</groupId> <artifactId>spring-kafka</artifactId> <version>2.1.0.RELEASE</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Gradle – build.gradle
buildscript { ext { springBootVersion = '1.5.9.RELEASE' } repositories { mavenCentral() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") } } apply plugin: 'java' apply plugin: 'eclipse' apply plugin: 'org.springframework.boot' group = 'com.tutorialspoint' version = '0.0.1-SNAPSHOT' sourceCompatibility = 1.8 repositories { mavenCentral() } dependencies { compile('org.springframework.boot:spring-boot-starter') compile group: 'org.springframework.kafka', name: 'spring-kafka', version: '2.1.0.RELEASE' testCompile('org.springframework.boot:spring-boot-starter-test') }
现在,创建一个可执行 JAR 文件,并使用以下 Maven 或 Gradle 命令运行 Spring Boot 应用程序,如下所示 -
对于 Maven,请使用如下所示的命令 -
mvn clean install
“BUILD SUCCESS”后,您可以在目标目录下找到JAR文件。
对于 Gradle,使用如下所示的命令 -
gradle clean build
“BUILD SUCCESSFUL”后,您可以在build/libs目录下找到JAR文件。
使用此处给出的命令运行 JAR 文件 -
java –jar <JARFILE>
您可以在控制台窗口中看到输出。