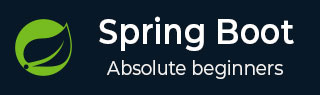
- Spring Boot 教程
- Spring Boot - 主页
- Spring Boot - 简介
- Spring Boot - 快速入门
- Spring Boot - 引导
- Spring Boot - Tomcat 部署
- Spring Boot - 构建系统
- Spring Boot - 代码结构
- Spring Bean 和依赖注入
- Spring Boot - 跑步者
- Spring Boot - 应用程序属性
- Spring Boot - 日志记录
- 构建 RESTful Web 服务
- Spring Boot - 异常处理
- Spring Boot - 拦截器
- Spring Boot - Servlet 过滤器
- Spring Boot - Tomcat 端口号
- Spring Boot - Rest 模板
- Spring Boot - 文件处理
- Spring Boot - 服务组件
- Spring Boot - Thymeleaf
- 使用 RESTful Web 服务
- Spring Boot - CORS 支持
- Spring Boot - 国际化
- Spring Boot - 调度
- Spring Boot - 启用 HTTPS
- Spring Boot-Eureka 服务器
- 向 Eureka 注册服务
- Zuul代理服务器和路由
- Spring Cloud配置服务器
- Spring Cloud 配置客户端
- Spring Boot - 执行器
- Spring Boot - 管理服务器
- Spring Boot - 管理客户端
- Spring Boot - 启用 Swagger2
- Spring Boot - 创建 Docker 镜像
- 追踪微服务日志
- Spring Boot - Flyway 数据库
- Spring Boot - 发送电子邮件
- Spring Boot-Hystrix
- Spring Boot - Web Socket
- Spring Boot - 批量服务
- Spring Boot-Apache Kafka
- Spring Boot - Twilio
- Spring Boot - 单元测试用例
- 休息控制器单元测试
- Spring Boot - 数据库处理
- 保护 Web 应用程序的安全
- Spring Boot - 使用 JWT 的 OAuth2
- Spring Boot - Google 云平台
- Spring Boot - Google OAuth2 登录
- Spring Boot 资源
- Spring Boot - 快速指南
- Spring Boot - 有用的资源
- Spring Boot - 讨论
Spring Boot - 国际化
国际化是一个使您的应用程序能够适应不同语言和地区而无需对源代码进行工程更改的过程。换句话说,国际化是本地化的准备。
在本章中,我们将详细学习如何在 Spring Boot 中实现国际化。
依赖关系
我们需要 Spring Boot Starter Web 和 Spring Boot Starter Thymeleaf 依赖项来在 Spring Boot 中开发 Web 应用程序。
梅文
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency>
摇篮
compile('org.springframework.boot:spring-boot-starter-web') compile group: 'org.springframework.boot', name: 'spring-boot-starter-thymeleaf'
区域解析器
我们需要确定您的应用程序的默认区域设置。我们需要在 Spring Boot 应用程序中添加 LocaleResolver bean。
@Bean public LocaleResolver localeResolver() { SessionLocaleResolver sessionLocaleResolver = new SessionLocaleResolver(); sessionLocaleResolver.setDefaultLocale(Locale.US); return sessionLocaleResolver; }
LocaleChange拦截器
LocaleChangeInterceptor 用于根据添加到请求的语言参数的值更改新的区域设置。
@Bean public LocaleChangeInterceptor localeChangeInterceptor() { LocaleChangeInterceptor localeChangeInterceptor = new LocaleChangeInterceptor(); localeChangeInterceptor.setParamName("language"); return localeChangeInterceptor; }
为了达到这种效果,我们需要将 LocaleChangeInterceptor 添加到应用程序的注册表拦截器中。配置类应扩展 WebMvcConfigurerAdapter 类并重写 addInterceptors() 方法。
@Override public void addInterceptors(InterceptorRegistry registry) { registry.addInterceptor(localeChangeInterceptor()); }
消息来源
Spring Boot 应用程序默认从类路径下的src/main/resources文件夹中获取消息源。默认区域设置消息文件名应为message.properties,每个区域设置的文件应命名为messages_XX.properties。“XX”代表区域设置代码。
所有消息属性都应用作密钥对值。如果在区域设置中找不到任何属性,应用程序将使用 messages.properties 文件中的默认属性。
默认的 messages.properties 将如下所示 -
welcome.text=Hi Welcome to Everyone
法语 messages_fr.properties 将如下所示 -
welcome.text=Salut Bienvenue à tous
注意- 消息源文件应保存为“UTF-8”文件格式。
HTML 文件
在 HTML 文件中,使用语法#{key}显示属性文件中的消息。
<h1 th:text = "#{welcome.text}"></h1>
完整代码如下
Maven – pom.xml
<?xml version = "1.0" encoding = "UTF-8"?> <project xmlns = "http://maven.apache.org/POM/4.0.0" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>demo</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>demo</name> <description>Demo project for Spring Boot</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.8.RELEASE</version> <relativePath /> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Gradle – build.gradle
buildscript { ext { springBootVersion = '1.5.8.RELEASE' } repositories { mavenCentral() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") } } apply plugin: 'java' apply plugin: 'eclipse' apply plugin: 'org.springframework.boot' group = 'com.tutorialspoint' version = '0.0.1-SNAPSHOT' sourceCompatibility = 1.8 repositories { mavenCentral() } dependencies { compile('org.springframework.boot:spring-boot-starter-web') compile group: 'org.springframework.boot', name: 'spring-boot-starter-thymeleaf' testCompile('org.springframework.boot:spring-boot-starter-test') }
主要的 Spring Boot 应用程序类文件如下 -
package com.tutorialspoint.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
控制器类文件如下 -
package com.tutorialspoint.demo.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; @Controller public class ViewController { @RequestMapping("/locale") public String locale() { return "locale"; } }
支持国际化的配置类
package com.tutorialspoint.demo; import java.util.Locale; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.web.servlet.LocaleResolver; import org.springframework.web.servlet.config.annotation.InterceptorRegistry; import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter; import org.springframework.web.servlet.i18n.LocaleChangeInterceptor; import org.springframework.web.servlet.i18n.SessionLocaleResolver; @Configuration public class Internationalization extends WebMvcConfigurerAdapter { @Bean public LocaleResolver localeResolver() { SessionLocaleResolver sessionLocaleResolver = new SessionLocaleResolver(); sessionLocaleResolver.setDefaultLocale(Locale.US); return sessionLocaleResolver; } @Bean public LocaleChangeInterceptor localeChangeInterceptor() { LocaleChangeInterceptor localeChangeInterceptor = new LocaleChangeInterceptor(); localeChangeInterceptor.setParamName("language"); return localeChangeInterceptor; } @Override public void addInterceptors(InterceptorRegistry registry) { registry.addInterceptor(localeChangeInterceptor()); } }
消息源 – messages.properties 如图所示 -
welcome.text = Hi Welcome to Everyone
消息源 – message_fr.properties 如图所示 -
welcome.text = Salut Bienvenue à tous
HTML 文件 locale.html 应放置在类路径上的 templates 目录下,如下所示 -
<!DOCTYPE html> <html> <head> <meta charset = "ISO-8859-1"/> <title>Internationalization</title> </head> <body> <h1 th:text = "#{welcome.text}"></h1> </body> </html>
您可以创建可执行 JAR 文件,并使用以下 Maven 或 Gradle 命令运行 Spring boot 应用程序 -
对于 Maven,请使用以下命令 -
mvn clean install
“BUILD SUCCESS”后,您可以在目标目录下找到JAR文件。
对于 Gradle,使用以下命令 -
gradle clean build
“BUILD SUCCESSFUL”后,您可以在build/libs目录下找到JAR文件。
现在,使用如下所示的命令运行 JAR 文件 -
java –jar <JARFILE>
您会发现应用程序已在 Tomcat 端口 8080 上启动。

现在在 Web 浏览器中输入 URL http://localhost:8080/locale,您可以看到以下输出 -

URL http://localhost:8080/locale?language=fr将为您提供如下所示的输出 -
