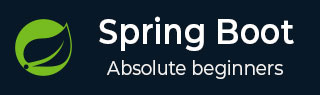
- Spring Boot 教程
- Spring Boot - 主页
- Spring Boot - 简介
- Spring Boot - 快速入门
- Spring Boot - 引导
- Spring Boot - Tomcat 部署
- Spring Boot - 构建系统
- Spring Boot - 代码结构
- Spring Bean 和依赖注入
- Spring Boot - 跑步者
- Spring Boot - 应用程序属性
- Spring Boot - 日志记录
- 构建 RESTful Web 服务
- Spring Boot - 异常处理
- Spring Boot - 拦截器
- Spring Boot - Servlet 过滤器
- Spring Boot - Tomcat 端口号
- Spring Boot - Rest 模板
- Spring Boot - 文件处理
- Spring Boot - 服务组件
- Spring Boot - Thymeleaf
- 使用 RESTful Web 服务
- Spring Boot - CORS 支持
- Spring Boot - 国际化
- Spring Boot - 调度
- Spring Boot - 启用 HTTPS
- Spring Boot-Eureka 服务器
- 向 Eureka 注册服务
- Zuul代理服务器和路由
- Spring Cloud配置服务器
- Spring Cloud 配置客户端
- Spring Boot - 执行器
- Spring Boot - 管理服务器
- Spring Boot - 管理客户端
- Spring Boot - 启用 Swagger2
- Spring Boot - 创建 Docker 镜像
- 追踪微服务日志
- Spring Boot - Flyway 数据库
- Spring Boot - 发送电子邮件
- Spring Boot-Hystrix
- Spring Boot - Web Socket
- Spring Boot - 批量服务
- Spring Boot-Apache Kafka
- Spring Boot - Twilio
- Spring Boot - 单元测试用例
- 休息控制器单元测试
- Spring Boot - 数据库处理
- 保护 Web 应用程序的安全
- Spring Boot - 使用 JWT 的 OAuth2
- Spring Boot - Google 云平台
- Spring Boot - Google OAuth2 登录
- Spring Boot 资源
- Spring Boot - 快速指南
- Spring Boot - 有用的资源
- Spring Boot - 讨论
Spring Boot - Google 云平台
Google Cloud Platform提供了在云环境中运行Spring Boot应用程序的云计算服务。在本章中,我们将了解如何在 GCP 应用引擎平台中部署 Spring Boot 应用程序。
首先,从 Spring Initializer 页面www.start.spring.io下载 Gradle 构建 Spring Boot 应用程序。请观察以下屏幕截图。
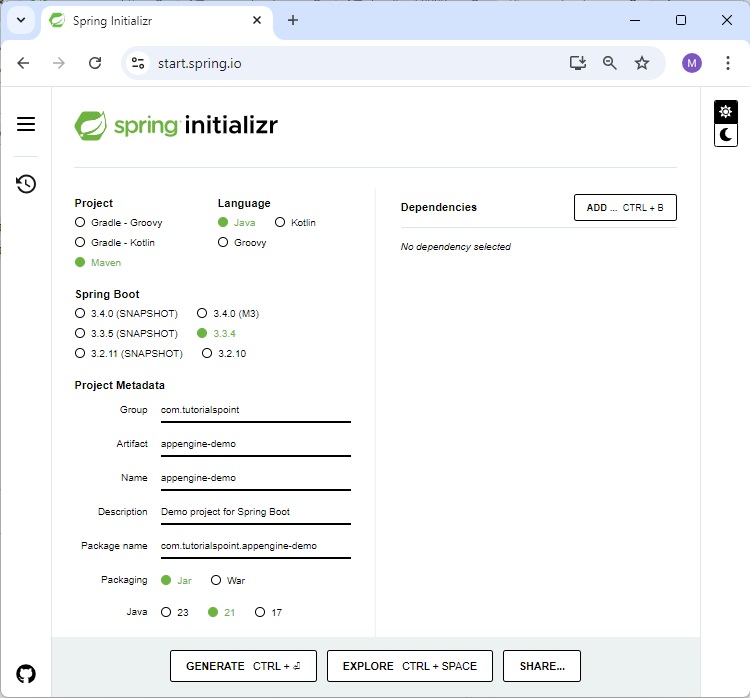
现在,在 build.gradle 文件中,添加 Google Cloud appengine 插件和 appengine 类路径依赖项。
build.gradle 文件的代码如下 -
buildscript { ext { springBootVersion = '1.5.9.RELEASE' } repositories { mavenCentral() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") classpath 'com.google.cloud.tools:appengine-gradle-plugin:1.3.3' } } apply plugin: 'java' apply plugin: 'eclipse' apply plugin: 'org.springframework.boot' apply plugin: 'com.google.cloud.tools.appengine' group = 'com.tutorialspoint' version = '0.0.1-SNAPSHOT' sourceCompatibility = 1.8 repositories { mavenCentral() } dependencies { compile('org.springframework.boot:spring-boot-starter-web') testCompile('org.springframework.boot:spring-boot-starter-test') }
现在,编写一个简单的 HTTP 端点,它返回字符串成功,如下所示 -
package com.tutorialspoint.appenginedemo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication @RestController public class AppengineDemoApplication { public static void main(String[] args) { SpringApplication.run(AppengineDemoApplication.class, args); } @RequestMapping(value = "/") public String success() { return "APP Engine deployment success"; } }
接下来,在 src/main/appengine 目录下添加 app.yml 文件,如下所示 -
runtime: java env: flex handlers: - url: /.* script: this field is required, but ignored
现在,转到 Google Cloud 控制台并单击页面顶部的激活 Google Cloud shell。

现在,使用 google cloud shell 将源文件和 Gradle 文件移动到 google cloud 计算机的主目录中。

现在,执行命令 gradle appengineDeploy ,它将把您的应用程序部署到 Google Cloud appengine 中。
注意- GCP 应启用计费,并且在将应用程序部署到 appengine 之前,您应在 GCP 中创建 appengine 平台。
将您的应用程序部署到 GCP appengine 平台需要几分钟的时间。
构建成功后,您可以在控制台窗口中看到服务 URL。
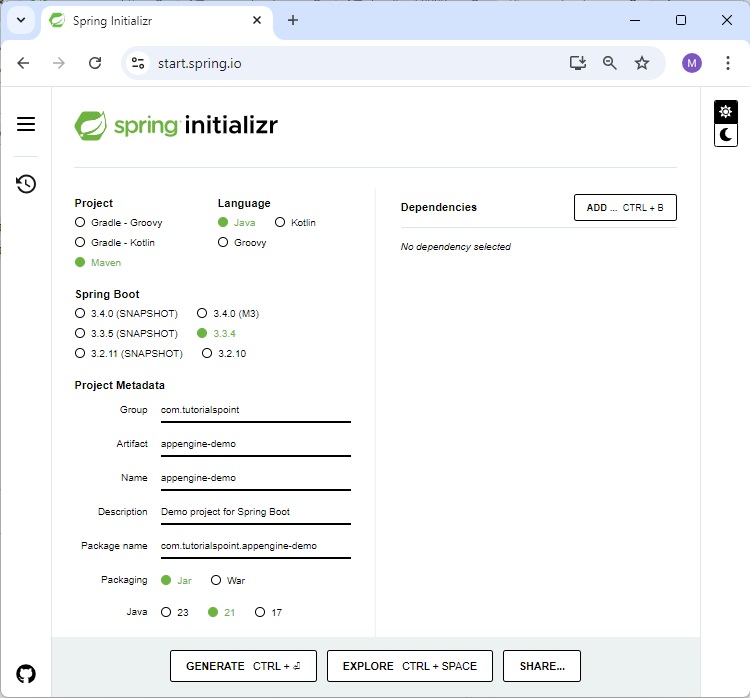
现在,点击服务 URL 并查看输出。

谷歌云SQL
要将 Google Cloud SQL 连接到 Spring Boot 应用程序,您应该将以下属性添加到 application.properties 文件中。
JDBC URL 格式
jdbc:mysql://google/<DATABASE-NAME>?cloudSqlInstance = <GOOGLE_CLOUD_SQL_INSTANCE_NAME> &socketFactory = com.google.cloud.sql.mysql.SocketFactory&user = <USERNAME>&password = <PASSWORD>
注意- Spring Boot 应用程序和 Google Cloud SQL 应位于同一 GCP 项目中。
application.properties 文件如下所示。
spring.dbProductService.driverClassName = com.mysql.jdbc.Driver spring.dbProductService.url = jdbc:mysql://google/PRODUCTSERVICE?cloudSqlInstance = springboot-gcp-cloudsql:asia-northeast1:springboot-gcp-cloudsql-instance&socketFactory = com.google.cloud.sql.mysql.SocketFactory&user = root&password = rootspring.dbProductService.username = root spring.dbProductService.password = root spring.dbProductService.testOnBorrow = true spring.dbProductService.testWhileIdle = true spring.dbProductService.timeBetweenEvictionRunsMillis = 60000 spring.dbProductService.minEvictableIdleTimeMillis = 30000 spring.dbProductService.validationQuery = SELECT 1 spring.dbProductService.max-active = 15 spring.dbProductService.max-idle = 10 spring.dbProductService.max-wait = 8000
YAML 文件用户可以将以下属性添加到您的 application.yml 文件中。
spring: datasource: driverClassName: com.mysql.jdbc.Driver url: "jdbc:mysql://google/PRODUCTSERVICE?cloudSqlInstance=springboot-gcp-cloudsql:asia-northeast1:springboot-gcp-cloudsql-instance&socketFactory=com.google.cloud.sql.mysql.SocketFactory&user=root&password=root" password: "root" username: "root" testOnBorrow: true testWhileIdle: true validationQuery: SELECT 1 max-active: 15 max-idle: 10 max-wait: 8000