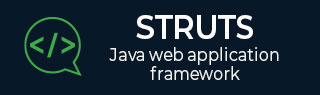
- Struts 2 教程
- Struts2 - 主页
- Struts2 - 基本 MVC 架构
- Struts2 - 概述
- Struts2 - 环境设置
- Struts2 - 架构
- Struts2 - 示例
- Struts2 - 配置
- Struts2 - 动作
- Struts2 - 拦截器
- Struts2 - 结果类型
- Struts2 - 价值堆栈/OGNL
- Struts2 - 文件上传
- Struts2 - 数据库访问
- Struts2 - 发送电子邮件
- Struts2 - 验证
- Struts2 - 本地化
- Struts2 - 类型转换
- Struts2 - 主题/模板
- Struts2 - 异常处理
- Struts2 - 注释
- Struts 2 集成
- Struts2-Spring
- Struts2 - 瓷砖
- Struts2-Hibernate
- Struts 2 有用资源
- Struts2 - 问题与解答
- Struts2 - 快速指南
- Struts2 - 有用的资源
- Struts2 - 讨论
Struts 2 - 发送电子邮件
本章介绍如何使用 Struts 2 应用程序发送电子邮件。
对于本练习,您需要从JavaMail API 1.4.4下载并安装 mail.jar ,并将mail.jar文件放置在 WEB-INF\lib 文件夹中,然后继续执行创建操作、视图和配置的标准步骤文件。
创建动作
下一步是创建一个负责发送电子邮件的操作方法。让我们创建一个名为Emailer.java的新类,其中包含以下内容。
package com.tutorialspoint.struts2; import java.util.Properties; import javax.mail.Message; import javax.mail.PasswordAuthentication; import javax.mail.Session; import javax.mail.Transport; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeMessage; import com.opensymphony.xwork2.ActionSupport; public class Emailer extends ActionSupport { private String from; private String password; private String to; private String subject; private String body; static Properties properties = new Properties(); static { properties.put("mail.smtp.host", "smtp.gmail.com"); properties.put("mail.smtp.socketFactory.port", "465"); properties.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory"); properties.put("mail.smtp.auth", "true"); properties.put("mail.smtp.port", "465"); } public String execute() { String ret = SUCCESS; try { Session session = Session.getDefaultInstance(properties, new javax.mail.Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication(from, password); } } ); Message message = new MimeMessage(session); message.setFrom(new InternetAddress(from)); message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(to)); message.setSubject(subject); message.setText(body); Transport.send(message); } catch(Exception e) { ret = ERROR; e.printStackTrace(); } return ret; } public String getFrom() { return from; } public void setFrom(String from) { this.from = from; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getTo() { return to; } public void setTo(String to) { this.to = to; } public String getSubject() { return subject; } public void setSubject(String subject) { this.subject = subject; } public String getBody() { return body; } public void setBody(String body) { this.body = body; } public static Properties getProperties() { return properties; } public static void setProperties(Properties properties) { Emailer.properties = properties; } }
如上面的源代码所示,Emailer.java具有与下面给出的 email.jsp 页面中的表单属性相对应的属性。这些属性是 -
From - 发件人的电子邮件地址。由于我们使用的是 Google 的 SMTP,因此我们需要一个有效的 gtalk id
密码- 上述帐户的密码
收件人- 将电子邮件发送给谁?
主题- 电子邮件的主题
正文- 实际的电子邮件消息
我们没有考虑对上述字段进行任何验证,验证将在下一章中添加。现在让我们看一下execute() 方法。execute() 方法使用 javax Mail 库通过提供的参数发送电子邮件。如果邮件发送成功,action 返回 SUCCESS,否则返回 ERROR。
创建主页
让我们编写主页JSP文件index.jsp,它将用于收集上述电子邮件相关信息 -
<%@ page language = "java" contentType = "text/html; charset = ISO-8859-1" pageEncoding = "ISO-8859-1"%> <%@ taglib prefix = "s" uri = "/struts-tags"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Email Form</title> </head> <body> <em>The form below uses Google's SMTP server. So you need to enter a gmail username and password </em> <form action = "emailer" method = "post"> <label for = "from">From</label><br/> <input type = "text" name = "from"/><br/> <label for = "password">Password</label><br/> <input type = "password" name = "password"/><br/> <label for = "to">To</label><br/> <input type = "text" name = "to"/><br/> <label for = "subject">Subject</label><br/> <input type = "text" name = "subject"/><br/> <label for = "body">Body</label><br/> <input type = "text" name = "body"/><br/> <input type = "submit" value = "Send Email"/> </form> </body> </html>
创建视图
我们将使用 JSP 文件success.jsp,如果操作返回 SUCCESS,将调用该文件,但如果操作返回错误,我们将有另一个视图文件。
<%@ page language = "java" contentType = "text/html; charset = ISO-8859-1" pageEncoding = "ISO-8859-1"%> <%@ taglib prefix = "s" uri = "/struts-tags"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Email Success</title> </head> <body> Your email to <s:property value = "to"/> was sent successfully. </body> </html>
以下是操作返回错误时的视图文件error.jsp 。
<%@ page language = "java" contentType = "text/html; charset = ISO-8859-1" pageEncoding = "ISO-8859-1"%> <%@ taglib prefix = "s" uri = "/struts-tags"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Email Error</title> </head> <body> There is a problem sending your email to <s:property value = "to"/>. </body> </html>
配置文件
现在让我们使用 struts.xml 配置文件将所有内容放在一起,如下所示 -
<?xml version = "1.0" Encoding = "UTF-8"?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <constant name = "struts.devMode" value = "true" /> <package name = "helloworld" extends = "struts-default"> <action name = "emailer" class = "com.tutorialspoint.struts2.Emailer" method = "execute"> <result name = "success">/success.jsp</result> <result name = "error">/error.jsp</result> </action> </package> </struts>
以下是web.xml文件的内容-
<?xml version = "1.0" Encoding = "UTF-8"?> <web-app xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns = "http://java.sun.com/xml/ns/javaee" xmlns:web = "http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation = "http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id = "WebApp_ID" version = "3.0"> <display-name>Struts 2</display-name> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> <filter> <filter-name>struts2</filter-name> <filter-class> org.apache.struts2.dispatcher.FilterDispatcher </filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> </web-app>
现在,右键单击项目名称,然后单击“导出”>“WAR 文件”以创建一个 War 文件。然后将此 WAR 部署到 Tomcat 的 webapps 目录中。最后,启动 Tomcat 服务器并尝试访问 URL http://localhost:8080/HelloWorldStruts2/index.jsp。这将产生以下屏幕 -
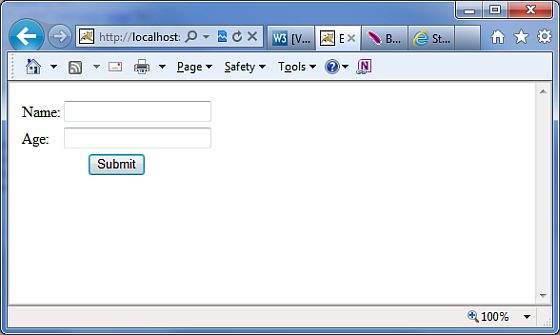
输入所需信息并单击“发送电子邮件”按钮。如果一切顺利,那么您应该会看到以下页面。
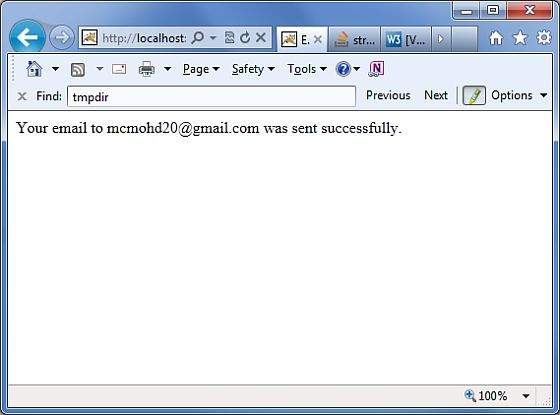