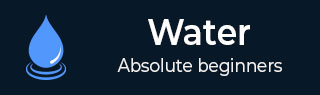
- Watir教程
- Watir - 主页
- Watir - 概述
- Watir - 简介
- Watir - 环境设置
- Watir - 安装浏览器驱动程序
- Watir - 使用浏览器
- Watir - 网页元素
- Watir - 定位网页元素
- Watir - 使用 Iframe
- Watir - 自动等待
- Watir - 无头测试
- Watir - 移动测试
- Watir - 捕获屏幕截图
- Watir - 页面对象
- Watir - 页面性能
- Watir - cookie
- Watir - 代理
- Watir - 警报
- Watir - 下载
- Watir - 浏览器 Windows
- Watir有用资源
- Watir - 快速指南
- Watir - 有用的资源
- Watir - 讨论
Watir - 网页元素
在本章中,我们将讨论如何在 Watir 中使用以下内容 -
- 使用文本框
- 使用组合
- 使用单选按钮
- 使用复选框
- 使用按钮
- 使用链接
- 与 Div 一起工作
使用文本框
句法
browser.text_field id: 'firstname' // will get the reference of the textbox
这里将尝试了解如何在 UI 上使用文本框。
考虑页面 Textbox.html 如下所示 -
<html> <head> <title>Testing UI using Watir</title> </head> <body> <script type = "text/javascript"> function wsentered() { console.log("inside wsentered"); var firstname = document.getElementById("firstname"); if (firstname.value != "") { document.getElementById("displayfirstname").innerHTML = "The name entered is : " + firstname.value; document.getElementById("displayfirstname").style.display = ""; } } </script> <div id = "divfirstname"> Enter First Name : <input type = "text" id = "firstname" name = "firstname" onchange = "wsentered()" /> </div> <br/> <br/> <div style = "display:none;" id = "displayfirstname"></div> </body> </html>
相应的输出如下所示 -

我们有一个文本框,当您输入名称时,会触发 onchange 事件,并且名称显示在下面。
现在让我们编写代码,其中我们将找到文本框并输入名称并触发 onchange 事件。
Watir密码
require 'watir' b = Watir::Browser.new :chrome b.goto('http://localhost/uitesting/textbox.html') t = b.text_field id: 'firstname' t.exists? t.set 'Riya Kapoor' t.value t.fire_event('onchange')
我们使用 Chrome 浏览器并将 pageurl 指定为http://localhost/uitesting/textbox.html。
使用goto api 浏览器将打开 pageurl,我们将找到 id:firstname 的 text_field。如果存在,我们将把 value 设置为 Riya Kapoor 并使用fire_event api 来触发 onchange 事件。
现在,让我们运行代码来显示输出,如下所示 -


使用组合
句法
browser.select_list id: 'months' // will get the reference of the dropdown
我们现在要测试的测试页面如下所示 -
<html> <head> <title>Dropdown</title> </head> <body> <script type = "text/javascript"> function wsselected() { var months = document.getElementById("months"); if (months.value != "") { document.getElementById("displayselectedmonth").innerHTML = "The month selected is : " + months.value; document.getElementById("displayselectedmonth").style.display = ""; } } </script> <form name = "myform" method = "POST"> <div> Month is : <select name = "months" id = "months" onchange = "wsselected()"> <option value = "">Select Month</option> <option value = "Jan">January</option> <option value = "Feb">February</option> <option value = "Mar">March</option> <option value = "Apr">April</option> <option value = "May">May</option> <option value = "Jun">June</option> <option value = "Jul">July</option> <option value = "Aug">August</option> <option value = "Sept">September</option> <option value = "Oct">October</option> <option value = "Nov">November</option> <option value = "Dec">December</option> </select> </div> <br/> <br/> <div style = "display:none;" id = "displayselectedmonth"> </div> </body> </html>
输出
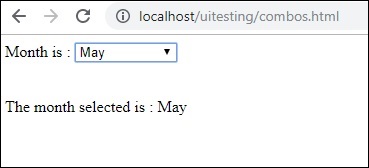
当您从下拉列表中选择月份时,下面会显示相同的内容。
现在让我们使用 Watir 进行相同的测试。
用于组合选择的 Watir 代码
require 'watir' b = Watir::Browser.new :chrome b.goto('http://localhost/uitesting/combos.html') t = b.select_list id: 'months' t.exists? t.select 'September' t.selected_options t.fire_event('onchange')
要使用组合,您需要使用 b.select_list api 后跟下拉列表的 id 来定位选择元素。要从下拉列表中选择值,您需要使用 t.select 和所需的值。
执行的输出如下 -

使用单选按钮
句法
browser.radio value: 'female' // will get the reference of the radio button with value “female”
这是一个测试页面,我们将使用它来处理单选按钮 -
<html> <head> <title>Testing UI using Watir</title> </head> <body> <form name = "myform" method = "POST"> <b>Select Gender?</b> <div> <br/> <input type = "radio" name = "gender" value = "male" checked> Male <br/> <input type = "radio" name = "gender" value = "female"> Female <br/> </div> </form> </body> </html>

我们将选择值为 Female 的单选按钮,如 Watir 代码所示 -
require 'watir' b = Watir::Browser.new b.goto('http://localhost/uitesting/radiobutton.html') t = b.radio value: 'female' t.exists? t.set b.screenshot.save 'radiobutton.png'
要使用单选按钮,我们需要告诉浏览器我们正在选择的值,即b.radio value:”female”
我们还截取屏幕截图并将其保存为 radiobutton.png,如下所示 -

使用复选框
句法
browser. checkbox value: 'Train' // will get the reference of the checkbox with value “Train”
这是复选框的测试页 -
<html> <head> <title>Testing UI using Watir</title> </head> <body> <form name = "myform" method = "POST"> <b>How would you like to travel?</b> <div> <br> <input type = "checkbox" name = "option1" value = "Car"> Car<br> <input type = "checkbox" name = "option2" value = "Bus"> Bus<br> <input type = "checkbox" name = "option3" value = "Train"> Train<br> <input type = "checkbox" name = "option4" value = "Air"> Airways<br> <br> </div> </form> </body> </html>

现在,让我们使用 Watir 在浏览器中找到该复选框,如下所示 -
require 'watir' b = Watir::Browser.new b.goto('http://localhost/uitesting/checkbox.html') t = b.checkbox value: 'Train' t.exists? t.set b.screenshot.save 'checkbox.png'
要在浏览器中找到复选框,请使用b.checkbox和要选择的值。

使用按钮
句法
browser.button(:name => "btnsubmit").click // will get the reference to the button element with has name “btnsubmit”
这是按钮的测试页 -
<html> <head> <title>Testing UI using Watir</title> </head> <body> <script type = "text/javascript"> function wsclick() { document.getElementById("buttondisplay").innerHTML = "Button is clicked"; document.getElementById("buttondisplay").style.display = ""; } </script> <form name = "myform" method = "POST"> <div> <br> <input type = "button" id = "btnsubmit" name = "btnsubmit" value = "submit" onclick = "wsclick()"/> <br> </div> </form> <br/> <div style = "display:none;" id = "buttondisplay"></div> </body> </html>

这是用于在给定页面上找到按钮的 watir 代码 -
require 'watir' b = Watir::Browser.new b.goto('http://localhost/uitesting/button.html') b.button(:name => "btnsubmit").click b.screenshot.save 'button.png'
这是截图按钮.png

使用链接
句法
browser.link text: 'Click Here' // will get the reference to the a tag with text ‘Click Here’
我们将使用以下测试页面来TestLink -
<html> <head> <title>Testing UI using Watir</title> </head> <body> <br/> <br/> <a href = "https://www.google.com">Click Here</a> <br/> </body> </html>
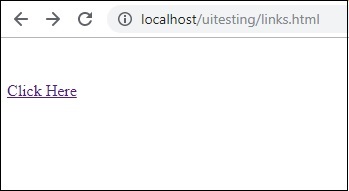
TestLink所需的 Watir 详细信息如下 -
require 'watir' b = Watir::Browser.new b.goto('http://localhost/uitesting/links.html') l = b.link text: 'Click Here' l.click b.screenshot.save 'links.png'
输出


与 Div 一起工作
句法
browser.div class: 'divtag' // will get the reference to div with class “divtag”
我们可以在其中测试 div 的测试页面。
<html> <head> <title>Testing UI using Watir</title> <style> .divtag { color: blue; font-size: 25px; } </style> </head> <body> <br/> <br/> <div class = "divtag"> UI Testing using Watir </div> <br/> </body> </html>
输出

用于测试 div 的 Watir 代码如下所示 -
require 'watir' b = Watir::Browser.new b.goto('http://localhost/uitesting/div.html') l = b.div class: 'divtag' l.exists? l.text b.screenshot.save 'divtag.png'
输出
