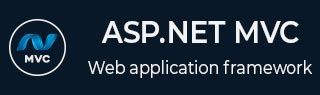
- ASP.NET MVC 教程
- ASP.NET MVC - 主页
- ASP.NET MVC - 概述
- ASP.NET MVC - 模式
- ASP.NET MVC - 环境设置
- ASP.NET MVC - 入门
- ASP.NET MVC - 生命周期
- ASP.NET MVC - 路由
- ASP.NET MVC - 控制器
- ASP.NET MVC - 操作
- ASP.NET MVC - 过滤器
- ASP.NET MVC - 选择器
- ASP.NET MVC - 视图
- ASP.NET MVC - 数据模型
- ASP.NET MVC - 帮助程序
- ASP.NET MVC - 模型绑定
- ASP.NET MVC - 数据库
- ASP.NET MVC - 验证
- ASP.NET MVC - 安全
- ASP.NET MVC - 缓存
- ASP.NET MVC - 剃刀
- ASP.NET MVC - 数据注释
- Nuget包管理
- ASP.NET MVC - Web API
- ASP.NET MVC - 脚手架
- ASP.NET MVC - 引导程序
- ASP.NET MVC - 单元测试
- ASP.NET MVC - 部署
- ASP.NET MVC - 自托管
- ASP.NET MVC 有用资源
- ASP.NET MVC - 快速指南
- ASP.NET MVC - 有用的资源
- ASP.NET MVC - 讨论
ASP.NET MVC - 数据库
在本教程中创建的所有 ASP.NET MVC 应用程序中,我们一直将硬编码数据从控制器传递到视图模板。但是,为了构建真正的 Web 应用程序,您可能需要使用真正的数据库。在本章中,我们将了解如何使用数据库引擎来存储和检索应用程序所需的数据。
为了存储和检索数据,我们将使用称为实体框架的 .NET Framework 数据访问技术来定义和使用模型。
实体框架 (EF) 支持 Code First 技术,该技术允许您通过编写简单的类来创建模型对象,然后将从您的类动态创建数据库,从而实现非常干净且快速的开发工作流程。
让我们看一个简单的示例,我们将在示例中添加对实体框架的支持。
步骤 1 - 要安装实体框架,请右键单击您的项目并选择 NuGet 包管理器 → 管理解决方案的 NuGet 包...

它将打开NuGet 包管理器。在搜索框中搜索实体框架。

选择实体框架并单击“安装”按钮。它将打开预览对话框。

单击“确定”继续。

单击“我接受”按钮开始安装。

安装实体框架后,您将在窗口中看到消息,如上面的屏幕截图所示。
添加数据库上下文
我们需要向 Employee Model 添加另一个类,该类将使用以下代码与实体框架通信以检索和保存数据。
using System; using System.Collections.Generic; using System.Data.Entity; using System.Linq; using System.Web; namespace MVCSimpleApp.Models{ public class Employee{ public int ID { get; set; } public string Name { get; set; } public DateTime JoiningDate { get; set; } public int Age { get; set; } } public class EmpDBContext : DbContext{ public EmpDBContext() { } public DbSet<Employee> Employees { get; set; } } }
如上所示,EmpDBContext派生自称为DbContext的 EF 类。在此类中,我们有一个名为 DbSet 的属性,它基本上代表您要查询和保存的实体。
连接字符串
我们需要在 Web.config 文件中的数据库的 <configuration> 标记下指定连接字符串。
<connectionStrings> <add name = "EmpDBContext" connectionString = "Data Source = (LocalDb)\v14.0;AttachDbFilename = |DataDirectory|\EmpDB.mdf;Initial Catalog = EmployeeDB;Integrated Security = SSPI;" providerName = "System.Data.SqlClient"/> </connectionStrings>
您实际上不需要添加 EmpDBContext 连接字符串。如果不指定连接字符串,实体框架将使用 DbContext 类的完全限定名称在用户目录中创建 localDB 数据库。在这个演示中,为了简单起见,我们不会添加连接字符串。
现在我们需要更新 EmployeeController.cs 文件,以便我们可以实际从数据库中保存和检索数据,而不是使用硬编码数据。
首先,我们添加创建一个私有 EmpDBContext 类对象,然后更新 Index、Create 和 Edit 操作方法,如以下代码所示。
using MVCSimpleApp.Models; using System.Linq; using System.Web.Mvc; namespace MVCSimpleApp.Controllers { public class EmployeeController : Controller{ private EmpDBContext db = new EmpDBContext(); // GET: Employee public ActionResult Index(){ var employees = from e in db.Employees orderby e.ID select e; return View(employees); } // GET: Employee/Create public ActionResult Create(){ return View(); } // POST: Employee/Create [HttpPost] public ActionResult Create(Employee emp){ try{ db.Employees.Add(emp); db.SaveChanges(); return RedirectToAction("Index"); }catch{ return View(); } } // GET: Employee/Edit/5 public ActionResult Edit(int id){ var employee = db.Employees.Single(m => m.ID == id); return View(employee); } // POST: Employee/Edit/5 [HttpPost] public ActionResult Edit(int id, FormCollection collection){ try{ var employee = db.Employees.Single(m => m.ID == id); if (TryUpdateModel(employee)){ //To Do:- database code db.SaveChanges(); return RedirectToAction("Index"); } return View(employee); }catch{ return View(); } } } }
然后我们使用以下 URL http://localhost:63004/Employee运行该应用程序。您将看到以下输出。

正如您所看到的,视图上没有数据,这是因为我们还没有在由 Visual Studio 创建的数据库中添加任何记录。
让我们转到 SQL Server 对象资源管理器,您将看到创建的数据库名称与 DBContext 类中的名称相同。

让我们扩展这个数据库,您将看到它有一个表,其中包含 Employee 模型类中的所有字段。

要查看此表中的数据,请右键单击“员工”表并选择“查看数据”。

您会看到我们目前没有记录。

让我们直接在数据库中添加一些记录,如下图所示。

刷新浏览器,您将看到数据现已从数据库更新到视图中。

让我们通过单击“新建”链接从浏览器添加一条记录。它将显示“创建”视图。

让我们在以下字段中添加一些数据。

单击“创建”按钮,它将更新索引视图并将此新记录添加到数据库中。

现在让我们进入 SQL Server 对象资源管理器并刷新数据库。右键单击“员工”表并选择“查看数据”菜单选项。您将看到该记录已添加到数据库中。
