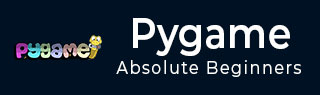
- Pygame 教程
- Pygame - 主页
- Pygame - 概述
- Pygame - 你好世界
- Pygame - 显示模式
- Pygame - 本地模块
- Pygame - 颜色对象
- Pygame - 事件对象
- Pygame - 键盘事件
- Pygame - 鼠标事件
- Pygame - 绘制形状
- Pygame - 加载图像
- Pygame - 在窗口中显示文本
- Pygame - 移动图像
- Pygame - 使用数字键盘移动
- Pygame - 用鼠标移动
- Pygame - 移动矩形对象
- Pygame - 使用文本作为按钮
- Pygame - 转换图像
- Pygame - 声音对象
- Pygame - 混合器通道
- Pygame - 播放音乐
- Pygame - 玩电影
- Pygame - 使用相机模块
- Pygame - 加载光标
- Pygame - 访问 CDROM
- Pygame - 精灵模块
- Pygame - PyOpenGL
- Pygame - 错误和异常
- Pygame 有用资源
- Pygame - 快速指南
- Pygame - 有用的资源
- Pygame - 讨论
Pygame - 移动图像
物体的移动是任何计算机游戏的一个重要方面。计算机游戏通过在增量位置绘制和擦除对象来创建运动错觉。以下代码通过在事件循环中增加 x 坐标位置并用背景颜色擦除它来绘制图像。
例子
image_filename = 'pygame.png' import pygame from pygame.locals import * from sys import exit pygame.init() screen = pygame.display.set_mode((400,300), 0, 32) pygame.display.set_caption("Moving Image") img = pygame.image.load(image_filename) x = 0 while True: screen.fill((255,255,255)) for event in pygame.event.get(): if event.type == QUIT: exit() screen.blit(img, (x, 100)) x= x+0.5 if x > 400: x = x-400 pygame.display.update()
输出
Pygame 徽标图像开始显示在左边框并反复向右移动。如果到达右边界,则其位置重置为左边界。
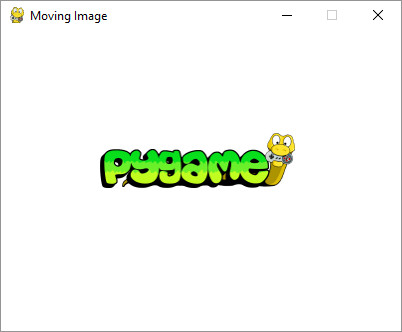
在下面的程序中,图像首先显示在 (0,150) 位置。当用户按下箭头键(左、右、上、下)时,图像的位置会改变 5 个像素。如果发生 KEYDOWN 事件,程序将检查键值是否为 K_LEFT、K_RIGHT、K_UP 或 K_DOWN。如果 x 坐标为 K_LEFT 或 K_RIGHT,则 x 坐标将更改 +5 或 -5。如果键值为 K_UP 或 K_DOWN,则 y 坐标的值会更改 -5 或 +5。
image_filename = 'pygame.png' import pygame from pygame.locals import * from sys import exit pygame.init() screen = pygame.display.set_mode((400,300)) pygame.display.set_caption("Moving with arrows") img = pygame.image.load(image_filename) x = 0 y= 150 while True: screen.fill((255,255,255)) screen.blit(img, (x, y)) for event in pygame.event.get(): if event.type == QUIT: exit() if event.type == KEYDOWN: if event.key == K_RIGHT: x= x+5 if event.key == K_LEFT: x=x-5 if event.key == K_UP: y=y-5 if event.key == K_DOWN: y=y+5 pygame.display.update()