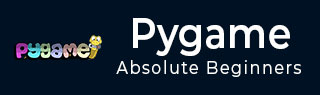
- Pygame 教程
- Pygame - 主页
- Pygame - 概述
- Pygame - 你好世界
- Pygame - 显示模式
- Pygame - 本地模块
- Pygame - 颜色对象
- Pygame - 事件对象
- Pygame - 键盘事件
- Pygame - 鼠标事件
- Pygame - 绘制形状
- Pygame - 加载图像
- Pygame - 在窗口中显示文本
- Pygame - 移动图像
- Pygame - 使用数字键盘移动
- Pygame - 用鼠标移动
- Pygame - 移动矩形对象
- Pygame - 使用文本作为按钮
- Pygame - 转换图像
- Pygame - 声音对象
- Pygame - 混合器通道
- Pygame - 播放音乐
- Pygame - 玩电影
- Pygame - 使用相机模块
- Pygame - 加载光标
- Pygame - 访问 CDROM
- Pygame - 精灵模块
- Pygame - PyOpenGL
- Pygame - 错误和异常
- Pygame 有用资源
- Pygame - 快速指南
- Pygame - 有用的资源
- Pygame - 讨论
Pygame - 精灵模块
任何在游戏窗口中绘制并且可以移动的位图都称为 Sprite。pygame.sprite 模块包含在游戏开发中有用的类和功能。除了用于创建精灵对象集合的 Sprite 类之外,还有一些函数可以实现精灵对象的碰撞。
Sprite 类充当游戏中不同对象的基类。您可能需要将另外一个对象分组。为此目的,还提供团体课程。
让我们首先通过子类化 sprite.Sprite 类来开发 Sprite 类。该 Block 类的每个对象都是一个填充黑色的矩形块。
class Block(pygame.sprite.Sprite): def __init__(self, color, width, height): super().__init__() self.image = pygame.Surface([width, height]) self.image.fill(color) self.rect = self.image.get_rect()
我们将创建 50 个块对象并将它们放入一个列表中。
for i in range(50): block = Block(BLACK, 20, 15) # Set a random location for the block block.rect.x = random.randrange(screen_width) block.rect.y = random.randrange(screen_height) # Add the block to the list of objects block_list.add(block) all_sprites_list.add(block)
我们创建一个红色块,将其称为播放器,并将其也添加到列表中。
# Create a RED player block player = Block(RED, 20, 15) all_sprites_list.add(player)
在游戏的事件循环中,检测红色块(玩家)随着鼠标运动和黑色块移动时的碰撞,并计算碰撞次数。
例子
事件循环代码如下 -
while not done: for event in pygame.event.get(): if event.type == pygame.QUIT: done = True # Clear the screen screen.fill(WHITE) # Get the current mouse position. This returns the position # as a list of two numbers. pos = pygame.mouse.get_pos() # Fetch the x and y out of the list, # just like we'd fetch letters out of a string. # Set the player object to the mouse location player.rect.x = pos[0] player.rect.y = pos[1] # See if the player block has collided with anything. blocks_hit_list = pygame.sprite.spritecollide(player, block_list, True) # Check the list of collisions. for block in blocks_hit_list: score += 1 print(score) # Draw all the spites all_sprites_list.draw(screen) # Go ahead and update the screen with what we've drawn. pygame.display.flip() # Limit to 60 frames per second clock.tick(60) pygame.quit()
输出
运行上面的代码。移动玩家方块以捕获尽可能多的黑色方块。分数将在控制台上回显。
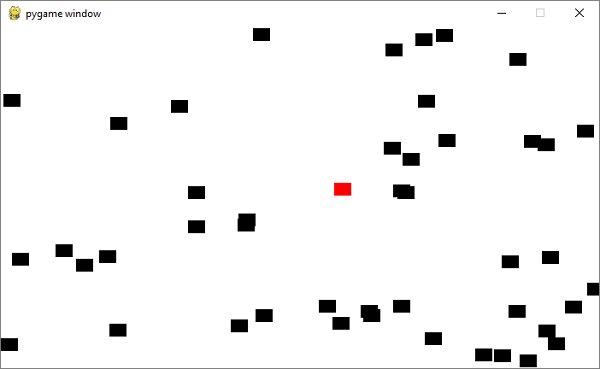