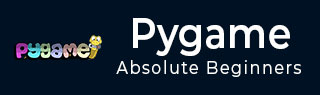
- Pygame 教程
- Pygame - 主页
- Pygame - 概述
- Pygame - 你好世界
- Pygame - 显示模式
- Pygame - 本地模块
- Pygame - 颜色对象
- Pygame - 事件对象
- Pygame - 键盘事件
- Pygame - 鼠标事件
- Pygame - 绘制形状
- Pygame - 加载图像
- Pygame - 在窗口中显示文本
- Pygame - 移动图像
- Pygame - 使用数字键盘移动
- Pygame - 用鼠标移动
- Pygame - 移动矩形对象
- Pygame - 使用文本作为按钮
- Pygame - 转换图像
- Pygame - 声音对象
- Pygame - 混合器通道
- Pygame - 播放音乐
- Pygame - 玩电影
- Pygame - 使用相机模块
- Pygame - 加载光标
- Pygame - 访问 CDROM
- Pygame - 精灵模块
- Pygame - PyOpenGL
- Pygame - 错误和异常
- Pygame 有用资源
- Pygame - 快速指南
- Pygame - 有用的资源
- Pygame - 讨论
Pygame - 移动矩形对象
Pygame.Rect 类具有存储和操作矩形区域的功能。矩形对象可以由左侧、顶部、宽度和高度值构造。矩形类中的函数可以复制、移动矩形对象并调整矩形对象的大小。
矩形对象具有以下虚拟属性 -

除了移动之外,Rect 类还具有测试矩形之间碰撞的方法。
复制() | 返回一个与原始矩形具有相同位置和大小的新矩形。 |
移动() | 返回移动给定偏移量的新矩形。x 和 y 参数可以是任何整数值,正数或负数。 |
move_ip() | 与 Rect.move() 方法相同,但就地操作。 |
膨胀(x,y) | 返回一个新的矩形,其大小按给定的偏移量更改。负值将缩小矩形。 |
inflate_ip(x, y) | 与 Rect.inflate() 方法相同,但就地操作。 |
夹具(矩形) | 返回一个新的矩形,该矩形被移动到完全位于参数 Rect 内。 |
剪辑(矩形) | 返回一个新的矩形,该矩形被裁剪为完全位于参数 Rect 内。 |
联合(矩形) | 返回一个新的矩形,该矩形完全覆盖所提供的两个矩形的区域。 |
union_ip(矩形) | 与 Rect.union() 方法相同,但操作就地。 |
包含(矩形) | 当参数完全位于 Rect 内时返回 true。 |
碰撞点((x,y)) | 如果给定点位于矩形内部,则返回 true。 |
碰撞直方图(矩形) | 如果任一矩形的任何部分重叠,则返回 true |
例子
在下面的程序中,用红色轮廓绘制了一个 Rect 对象。使用 copy() 方法,创建其克隆以进行移动。移动是通过 move_ip() 方法实现的。箭头键通过将 x/y 坐标增加/减少 + 或 -5 像素来移动复制矩形的位置。
import pygame from pygame.locals import * from sys import exit pygame.init() screen = pygame.display.set_mode((400,300)) rect1 = Rect(50, 60, 200, 80) rect2=rect1.copy() running = True x=0 y=0 while running: for event in pygame.event.get(): if event.type == QUIT: running = False if event.type == KEYDOWN: if event.key==K_LEFT: x= -5 y=0 if event.key == K_RIGHT: x=5 y=0 if event.key == K_UP: x = 0 y = -5 if event.key == K_DOWN: x = 0 y = 5 rect2.move_ip(x,y) screen.fill((127,127,127)) pygame.draw.rect(screen, (255,0,0), rect1, 1) pygame.draw.rect(screen, (0,0,255), rect2, 5) pygame.display.update() pygame.quit()
输出
以下输出显示带有红色轮廓的矩形是原始矩形。它的副本会响应箭头键而不断移动,并具有蓝色轮廓

例子
将 move_ip() 方法更改为 inflate_ip() 方法,以根据按下的箭头增大/缩小矩形。
while running: for event in pygame.event.get(): if event.type == QUIT: running = False if event.type == KEYDOWN: if event.key==K_LEFT: x= -5 y=0 if event.key == K_RIGHT: x=5 y=0 if event.key == K_UP: x = 0 y = -5 if event.key == K_DOWN: x = 0 y = 5 rect2.inflate_ip(x,y) screen.fill((127,127,127)) pygame.draw.rect(screen, (255,0,0), rect1, 1) pygame.draw.rect(screen, (0,0,255), rect2, 5) pygame.display.update()
输出
以下是箭头键按下活动的屏幕截图 -

例子
为了通过检测 MOUSEMOTION 事件使矩形移动,我们需要首先在原始矩形内按下鼠标。为了验证鼠标位置是否在矩形内部,我们使用 Rect 对象的 collidepoint() 方法。当鼠标移动时,矩形对象通过 move_ip() 方法移动到位。释放鼠标时移动应停止。
import pygame from pygame.locals import * from sys import exit pygame.init() screen = pygame.display.set_mode((400,300)) rect = Rect(50, 60, 200, 80) moving = False running = True while running: for event in pygame.event.get(): if event.type == QUIT: running = False elif event.type == MOUSEBUTTONDOWN: if rect.collidepoint(event.pos): moving = True elif event.type == MOUSEBUTTONUP: moving = False elif event.type == MOUSEMOTION and moving: rect.move_ip(event.rel) screen.fill((127,127,127)) pygame.draw.rect(screen, (255,0,0), rect) if moving: pygame.draw.rect(screen, (0,0,255), rect, 4) pygame.display.flip() pygame.quit()
输出


例子
要使用鼠标绘制矩形,请在 MOUSEBUTTONDOWN 和 MOUSEBUTTONUP 事件中捕获鼠标指针坐标,计算左上角坐标、宽度和高度并调用 rect() 函数。
import pygame from pygame.locals import * from sys import exit pygame.init() screen = pygame.display.set_mode((400,300)) pygame.display.set_caption("Draw Rectangle with Mouse") screen.fill((127,127,127)) x=0 y=0 w=0 h=0 drawmode=True running = True while running: for event in pygame.event.get(): if event.type == QUIT: running = False if event.type == MOUSEBUTTONDOWN: x,y = pygame.mouse.get_pos() drawmode = True if event.type == MOUSEBUTTONUP: x1,y1 = pygame.mouse.get_pos() w=x1-x h=y1-y drawmode= False rect = pygame.Rect(x,y,w,h) if drawmode == False: pygame.draw.rect(screen, (255,0,0), rect) pygame.display.flip() pygame.quit()
输出
